After the user fills in all the entry fields and click on "Add" button, the program checks if the ID already exists. If yes, pop up a message box saying the member ID already exists. Otherwise add the the account to the member account set (a dictionary or list). Disply the new account at the bottom Text field. After the user fills in the ID field and click on "Delete" button, the program checks if the ID already exists. If yes, delete the member associated with the ID. Otherwise, pop up a message box saying the member does not exists After the user fills in the ID field and click on “Edit" button, the program checks if the ID already exists. If yes, the program extracts the user input from the Phnoe and Weight fileds and update this member info accordingly. Otherwise, pop up a message box saying the member does not exists. Update cannot be made. After the user fills in the ID field and click on "Search" button, the program checks if the ID already exists. If yes, the program displays all information (Name, Phon, Weigh, Gender) of the member associated with the ID at the coresponding widget. Otherwise, pop up a message box saying the ID does not exists. After the user clicks on the "View All" button, the program displays all member info at the Text widget. b) d)
class ClubMember:
def __init__(self,id,name,gender,weight,phone):
self.id = id
self.name = name
self.gender = gender
self.weight = weight
self.phone = phone
def __str__(self):
return "Id:"+str(self.id)+",Name:"+self.name+",Gender:"+self.gender+",Weight:"+str(self.weight)+",Phone:"+str(self.phone)
class Club:
def __init__(self,name):
self.name = name
self.members = {}
self.membersCount = 0
def run(self):
print("Welcome to "+self.name+"!")
while True:
print("""
1. Add New Member
2. Delete a Member
3. Search for a member
4. Browse All Members
5. Edit Member
6. Exit
""")
choice = int(input("Choice:"))
if choice == 1:
self.membersCount = self.membersCount+1
id = self.membersCount
name = input("Member name:")
gender = input("Gender:")
weight = int(input("Weight:"))
phone = int(input("Phone No."))
member = ClubMember(id,name,gender,weight,phone)
self.members[id] = member
elif choice == 2:
id = int(input("Enter id of member to delete:"))
del self.members[id]
break
elif choice == 3:
id = int(input("Enter member id to search:"))
member = self.members[id]
print(member)
elif choice == 4:
print("Details of All members:")
for member in self.members.values():
print(member)
elif choice == 5:
id = int(input("Enter id of member to edit:"))
self.members[id].weight = int(input("Enter new weight of member:"))
self.members[id].phone = int(input("Enter new phone no. of member:"))
elif choice == 6:
break
club = Club("Weight Loss Club")
club.run()



Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 8 images

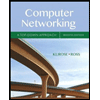
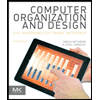
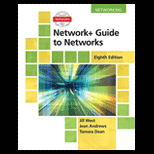
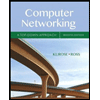
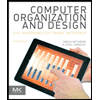
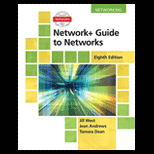
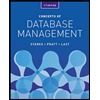
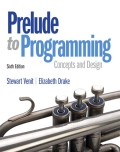
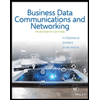