class Widget: """A class representing a simple Widget === Instance Attributes (the attributes of this class and their types) === name: the name of this Widget (str) cost: the cost of this Widget (int); cost >= 0 === Sample Usage (to help you understand how this class would be used) === >>> my_widget = Widget('Puzzle', 15) >>> my_widget.name 'Puzzle' >>> my_widget.cost 15 >>> my_widget.is_cheap() False >>> your_widget = Widget("Rubik's Cube", 6) >>> your_widget.name
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
class Widget:
"""A class representing a simple Widget
=== Instance Attributes (the attributes of this class and their types) ===
name: the name of this Widget (str)
cost: the cost of this Widget (int); cost >= 0
=== Sample Usage (to help you understand how this class would be used) ===
>>> my_widget = Widget('Puzzle', 15)
>>> my_widget.name
'Puzzle'
>>> my_widget.cost
15
>>> my_widget.is_cheap()
False
>>> your_widget = Widget("Rubik's Cube", 6)
>>> your_widget.name
"Rubik's Cube"
>>> your_widget.cost
6
>>> your_widget.is_cheap()
True
"""
# Add your methods here
if __name__ == '__main__':
import doctest
# Uncomment the line below if you prefer to test your examples with doctest
# doctest.testmod()

Step by step
Solved in 3 steps with 1 images

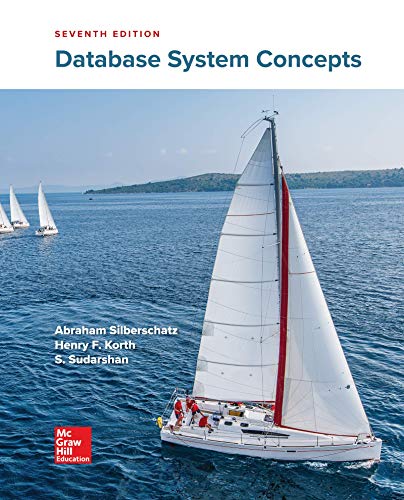
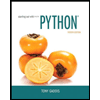
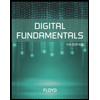
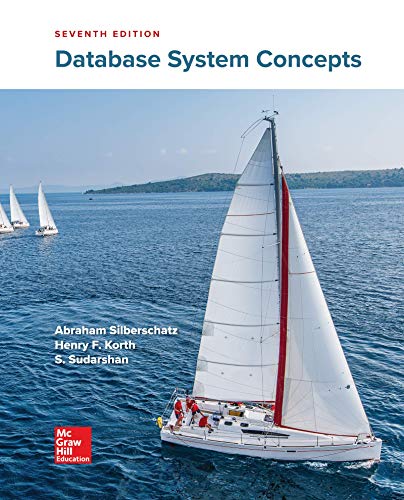
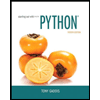
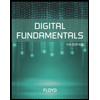
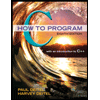
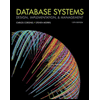
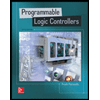