How do I create an object? class VendingMachine: def __init__(self): self.bottles = 20 def purchase(self, amount): self.bottles = self.bottles - amount def restock(self, amount): self.bottles = self.bottles + amount def get_inventory(self): return self.bottles def report(self): print(f'Inventory: {self.bottles} bottles') if __name__ == "__main__": # TODO: Create VendingMachine object # TODO: Purchase input number of drinks purchase = input() # TODO: Restock input number of bottles restock = input() # TODO: Report inventory print('Inventory:' VendingMachine - purchase + restock)
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
How do I create an object?
class VendingMachine:
def __init__(self):
self.bottles = 20
def purchase(self, amount):
self.bottles = self.bottles - amount
def restock(self, amount):
self.bottles = self.bottles + amount
def get_inventory(self):
return self.bottles
def report(self):
print(f'Inventory: {self.bottles} bottles')
if __name__ == "__main__":
# TODO: Create VendingMachine object
# TODO: Purchase input number of drinks
purchase = input()
# TODO: Restock input number of bottles
restock = input()
# TODO: Report inventory
print('Inventory:' VendingMachine - purchase + restock)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

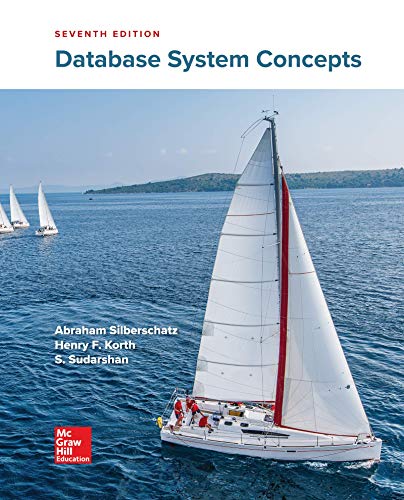
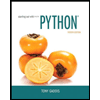
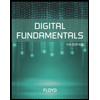
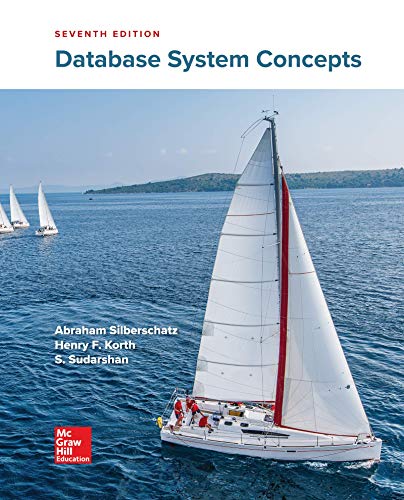
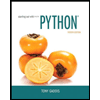
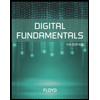
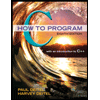
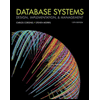
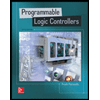