Car Class Project The car classwill havethe following attributes: •year: an integer that holds the car's model year •model: a string that holds the make of the car •make: a string that holds the model of the car •speed: an integer that holds the car's current speed Your class should contain thefollowing: A docstring that briefly describes the class and lists the attributes. The docstring will serve as the documentation for your class. •A constructor (__init__ method) that takes the car's year model and make as optional arguments. The constructor will set the value of the speed attribute to 0.•An __str__ method that returns the car's year model and makein a string. •To test your class, create acar object and use the print function to verify that the constructor and __str__ methodsare working correctly. •An accessor method which returns the value stored in the speed instance variable. Call this method getSpeed(). •A modifier method called accelerate() which adds 5 to the speed variable each time it is called •A modifier method called brake() which subtracts 5 from the speed variable each time it is called. Do not allow the value of the speed variable to be < 0. Design a program to test your class in themain.pyfunctionwhich does the following: •Createa car object •Use the print function to display the year,model,and make of the car. •Write a loop that calls the accelerate method 5 times. After each call, get the current speed and display it. •Write anotherloop that calls the brake method 5 times. After each call, get the current speed and displ
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Car Class Project
The car classwill havethe following attributes:
•year: an integer that holds the car's model year
•model: a string that holds the make of the car
•make: a string that holds the model of the car
•speed: an integer that holds the car's current speed
Your class should contain thefollowing:
A docstring that briefly describes the class and lists the attributes. The docstring will serve as the documentation for your class.
•A constructor (__init__ method) that takes the car's year model and make as optional arguments. The constructor will set the value of the speed attribute to 0.•An __str__ method that returns the car's year model and makein a string.
•To test your class, create acar object and use the print function to verify that the constructor and __str__ methodsare working correctly.
•An accessor method which returns the value stored in the speed instance variable. Call this method getSpeed().
•A modifier method called accelerate() which adds 5 to the speed variable each time it is called
•A modifier method called brake() which subtracts 5 from the speed variable each time it is called. Do not allow the value of the speed variable to be < 0.
Design a program to test your class in themain.pyfunctionwhich does the following:
•Createa car object •Use the print function to display the year,model,and make of the car.
•Write a loop that calls the accelerate method 5 times. After each call, get the current speed and display it.
•Write anotherloop that calls the brake method 5 times. After each call, get the current speed and display it.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

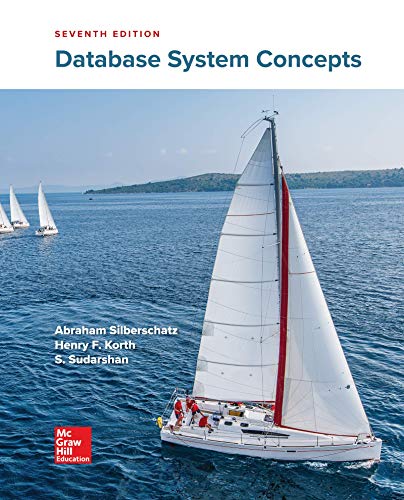
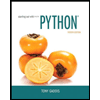
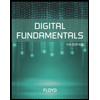
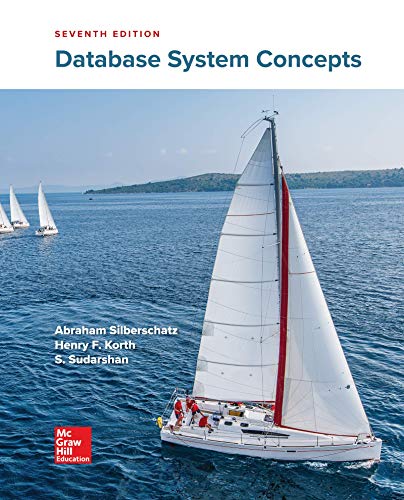
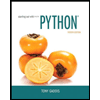
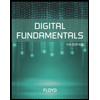
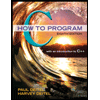
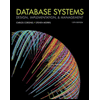
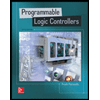