Draw the follClass Structure: 1. NotificationSystem: This is the main class that manages the notification system. It has the following attributes and methods: - Attributes: - users: a list of User objects representing all the users registered in the system - tweets: a list of Tweet objects representing all the tweets posted in the system - Methods: - register_user(user): adds a new user to the system - post_tweet(user, message): posts a new tweet on behalf of a user - get_timeline(user): returns a list of tweets from all users that the specified user follows - get_mentions(user): returns a list of tweets that mention the specified user 2. User: This class represents a user in the system. It has the following attributes and methods: - Attributes: - username: a string representing the username of the user - followers: a list of User objects representing all users who follow this user - following: a list of User objects representing all users whom this user follows - Methods: - follow(user): adds another user to this user's following list and adds this user to that user's followers list 3. Tweet: This class represents a tweet in the system. It has the following attributes and methods: - Attributes: - author: a User object representing the author of this tweet - message: a string representing the content of this tweet - Methods: None Functions' Specification: 1. register_user(user) - Input: A User object representing the new user to be registered in the system. - Output: None. - Description: Adds a new user to the notification system. 2. post_tweet(user, message) - Input: A User object representing the author of this tweet, and a string representing its content. - Output: None. - Description: Posts a new tweet on behalf of an existing user. 3. get_timeline(user) - Input: A User object representing the user whose timeline is requested. - Output: A list of Tweet objects representing all the tweets from all users that the specified user follows. - Description: Returns a list of tweets from all users that the specified user follows. 4. get_mentions(user) - Input: A User object representing the user whose mentions are requested. - Output: A list of Tweet objects representing all the tweets that mention the specified user. - Description: Returns a list of tweets that mention the specified user. 5. follow(user1, user2) - Input: Two User objects representing the users involved in this action (user1 follows user2). - Output: None. - Description: Adds another user to this user's following list and adds this user to that other user's followers list.
Draw the follClass Structure:
1. NotificationSystem: This is the main class that manages the notification system. It has the following attributes and methods:
- Attributes:
- users: a list of User objects representing all the users registered in the system
- tweets: a list of Tweet objects representing all the tweets posted in the system
- Methods:
- register_user(user): adds a new user to the system
- post_tweet(user, message): posts a new tweet on behalf of a user
- get_timeline(user): returns a list of tweets from all users that the specified user follows
- get_mentions(user): returns a list of tweets that mention the specified user
2. User: This class represents a user in the system. It has the following attributes and methods:
- Attributes:
- username: a string representing the username of the user
- followers: a list of User objects representing all users who follow this user
- following: a list of User objects representing all users whom this user follows
- Methods:
- follow(user): adds another user to this user's following list and adds this user to that user's followers list
3. Tweet: This class represents a tweet in the system. It has the following attributes and methods:
- Attributes:
- author: a User object representing the author of this tweet
- message: a string representing the content of this tweet
- Methods:
None
Functions' Specification:
1. register_user(user)
- Input: A User object representing the new user to be registered in the system.
- Output: None.
- Description: Adds a new user to the notification system.
2. post_tweet(user, message)
- Input: A User object representing the author of this tweet, and a string representing its content.
- Output: None.
- Description: Posts a new tweet on behalf of an existing user.
3. get_timeline(user)
- Input: A User object representing the user whose timeline is requested.
- Output: A list of Tweet objects representing all the tweets from all users that the specified user follows.
- Description: Returns a list of tweets from all users that the specified user follows.
4. get_mentions(user)
- Input: A User object representing the user whose mentions are requested.
- Output: A list of Tweet objects representing all the tweets that mention the specified user.
- Description: Returns a list of tweets that mention the specified user.
5. follow(user1, user2)
- Input: Two User objects representing the users involved in this action (user1 follows user2).
- Output: None.
- Description: Adds another user to this user's following list and adds this user to that other user's followers list.

Step by step
Solved in 3 steps with 5 images

Draw the following Class Structure as a (class digram):
1. NotificationSystem: This is the main class that manages the notification system. It has the following attributes and methods:
- Attributes:
- users: a list of User objects representing all the users registered in the system
- tweets: a list of Tweet objects representing all the tweets posted in the system
- Methods:
- register_user(user): adds a new user to the system
- post_tweet(user, message): posts a new tweet on behalf of a user
- get_timeline(user): returns a list of tweets from all users that the specified user follows
- get_mentions(user): returns a list of tweets that mention the specified user
2. User: This class represents a user in the system. It has the following attributes and methods:
- Attributes:
- username: a string representing the username of the user
- followers: a list of User objects representing all users who follow this user
- following: a list of User objects representing all users whom this user follows
- Methods:
- follow(user): adds another user to this user's following list and adds this user to that user's followers list
3. Tweet: This class represents a tweet in the system. It has the following attributes and methods:
- Attributes:
- author: a User object representing the author of this tweet
- message: a string representing the content of this tweet
- Methods:
None
Functions' Specification:
1. register_user(user)
- Input: A User object representing the new user to be registered in the system.
- Output: None.
- Description: Adds a new user to the notification system.
2. post_tweet(user, message)
- Input: A User object representing the author of this tweet, and a string representing its content.
- Output: None.
- Description: Posts a new tweet on behalf of an existing user.
3. get_timeline(user)
- Input: A User object representing the user whose timeline is requested.
- Output: A list of Tweet objects representing all the tweets from all users that the specified user follows.
- Description: Returns a list of tweets from all users that the specified user follows.
4. get_mentions(user)
- Input: A User object representing the user whose mentions are requested.
- Output: A list of Tweet objects representing all the tweets that mention the specified user.
- Description: Returns a list of tweets that mention the specified user.
5. follow(user1, user2)
- Input: Two User objects representing the users involved in this action (user1 follows user2).
- Output: None.
- Description: Adds another user to this user's following list and adds this user to that other user's followers list.
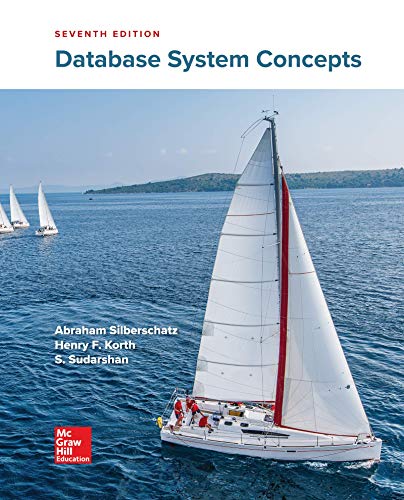
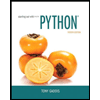
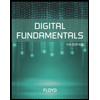
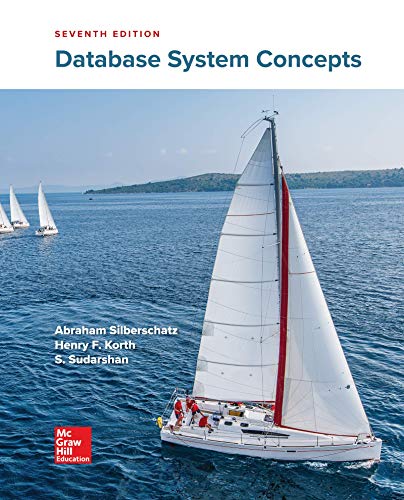
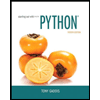
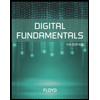
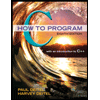
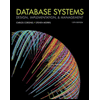
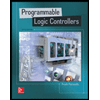