Create a base class called Shape with two final variables: PI and shapeType. PI should be set to 3.14159 and shapeType should be a String that indicates the type of shape. Create a subclass called Circle that extends Shape. Circle should have an instance variable called radius and a constructor that initializes radius. Override the toString() method to display the details of the circle, including its shape type, radius, and area. Create another subclass called Rectangle that extends Shape. Rectangle should have two instance variables called length and width, and a constructor that initializes length and width. Override the toString() method to display the details of the rectangle, including its shape type, length, width, and area. In the main method, create objects of Circle and Rectangle and call their toString() methods to demonstrate inheritance and the use of final variables.
Inheritance
Create a base class called Shape with two final variables: PI and shapeType. PI should be set to 3.14159 and shapeType should be a String that indicates the type of shape.
Create a subclass called Circle that extends Shape. Circle should have an instance variable called radius and a constructor that initializes radius. Override the toString() method to display the details of the circle, including its shape type, radius, and area.
Create another subclass called Rectangle that extends Shape. Rectangle should have two instance variables called length and width, and a constructor that initializes length and width. Override the toString() method to display the details of the rectangle, including its shape type, length, width, and area.
In the main method, create objects of Circle and Rectangle and call their toString() methods to demonstrate inheritance and the use of final variables.

Step by step
Solved in 4 steps with 3 images

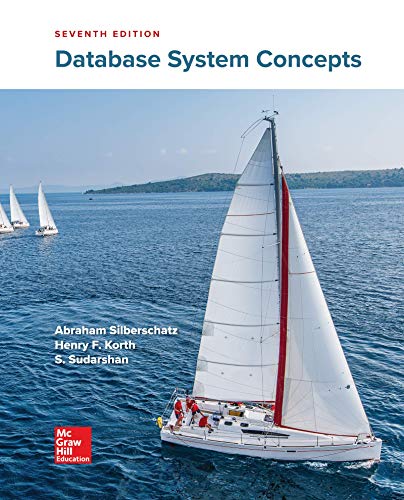
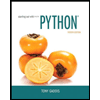
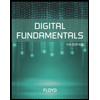
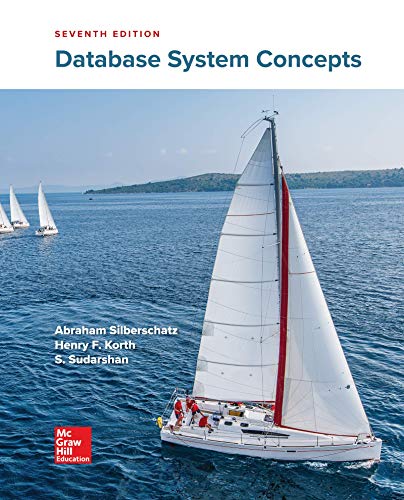
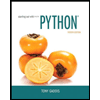
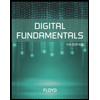
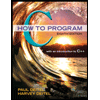
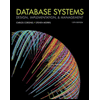
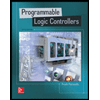