1. A polynomial can be represented as a linked list, where each node called a polyNode contains the coefficient and the exponent of a term of the polynomial. For example, the polynomial 4x³ + 3x²-5 would be represented as the linked list: 43 I 3 L -50 - 5xo 2 7. 4x3 3x² Write a Polynomial class that has methods for creating a polynomial, reading and writing a polynomial, and adding a pair of polymomials. In order to add 2 polynomials, traverse both lists. If a particular exponent value is present in either one, it should also be present in the resulting polynomial unless its coefficient is zero.
1. A polynomial can be represented as a linked list, where each node called a polyNode contains the coefficient and the exponent of a term of the polynomial. For example, the polynomial 4x³ + 3x²-5 would be represented as the linked list: 43 I 3 L -50 - 5xo 2 7. 4x3 3x² Write a Polynomial class that has methods for creating a polynomial, reading and writing a polynomial, and adding a pair of polymomials. In order to add 2 polynomials, traverse both lists. If a particular exponent value is present in either one, it should also be present in the resulting polynomial unless its coefficient is zero.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![**CSC 236 - Lab 3 (2 programs) LLL**
1. **Polynomial Representation Using Linked Lists**
A polynomial can be represented as a linked list, where each node, called a polyNode, contains the coefficient and the exponent of a term of the polynomial.
For example, the polynomial \(4x^3 + 3x^2 - 5\) would be represented as the linked list:
\[
\begin{array}{c}
\overset{4x^3}{\fbox{4 \, | \, 3 \rightarrow}} \,
\overset{3x^2}{\fbox{3 \, | \, 2 \rightarrow}} \,
\overset{-5x^0}{\fbox{-5 \, | \, 0 \, /}}
\end{array}
\]
- **Task:** Write a Polynomial class that has methods for creating a polynomial, reading and writing a polynomial, and adding a pair of polynomials.
- **Addition of Polynomials:** In order to add two polynomials, traverse both lists. If a particular exponent value is present in either one, it should also be present in the resulting polynomial unless its coefficient is zero.
2. **Student Course Enrollment at Middlesex County College**
Each student at Middlesex County College takes a different number of courses, so the registrar has decided to use linear linked lists to store each student’s class schedule and an array to represent the entire student body.
A portion of this data structure is shown below:
\[
\begin{array}{c}
\begin{array}{ccc}
ID & \text{link} & \text{sec} & Cr & \text{link} \\
\phi & & \overset{1}{\fbox{CSC162 \, | \, 1 \, | \, 3 \rightarrow}} \,
\overset{2}{\fbox{HIS101 \, | \, 2 \, | \, 4 \, /}} \\
1 & 1111 & & \\
1 & 1234 & & \\
2 & 2357 & \overset{4}{\fbox{CSC236 \, | \, 4 \, |](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd3ed3f65-79b2-4bdf-866b-dcdc9cf257bd%2F1f90d7ff-7445-4109-8af0-95f61ac3f0c9%2Fsf5ug1r_processed.jpeg&w=3840&q=75)
Transcribed Image Text:**CSC 236 - Lab 3 (2 programs) LLL**
1. **Polynomial Representation Using Linked Lists**
A polynomial can be represented as a linked list, where each node, called a polyNode, contains the coefficient and the exponent of a term of the polynomial.
For example, the polynomial \(4x^3 + 3x^2 - 5\) would be represented as the linked list:
\[
\begin{array}{c}
\overset{4x^3}{\fbox{4 \, | \, 3 \rightarrow}} \,
\overset{3x^2}{\fbox{3 \, | \, 2 \rightarrow}} \,
\overset{-5x^0}{\fbox{-5 \, | \, 0 \, /}}
\end{array}
\]
- **Task:** Write a Polynomial class that has methods for creating a polynomial, reading and writing a polynomial, and adding a pair of polynomials.
- **Addition of Polynomials:** In order to add two polynomials, traverse both lists. If a particular exponent value is present in either one, it should also be present in the resulting polynomial unless its coefficient is zero.
2. **Student Course Enrollment at Middlesex County College**
Each student at Middlesex County College takes a different number of courses, so the registrar has decided to use linear linked lists to store each student’s class schedule and an array to represent the entire student body.
A portion of this data structure is shown below:
\[
\begin{array}{c}
\begin{array}{ccc}
ID & \text{link} & \text{sec} & Cr & \text{link} \\
\phi & & \overset{1}{\fbox{CSC162 \, | \, 1 \, | \, 3 \rightarrow}} \,
\overset{2}{\fbox{HIS101 \, | \, 2 \, | \, 4 \, /}} \\
1 & 1111 & & \\
1 & 1234 & & \\
2 & 2357 & \overset{4}{\fbox{CSC236 \, | \, 4 \, |

Transcribed Image Text:### Program #2
#### 1. Show `StudentsADT` Interface
#### 2. Create a `Course` Class
Design a `Course` class with the following methods:
- **Default Constructor**
- **Overloaded Constructor**
- **Copy Constructor**
- `setCourseName`
- `setSectionNumber`
- `setNumberOfCredits`
- `setLink`
- `getCourseName`
- `getSectionNumber`
- `getNumberOfCredits`
- `getLink`
- `toString`
#### 3. Create a `Students` Class
Design a `Students` class with the following methods:
- **Default Constructor**
- **Overloaded Constructor**
- **Copy Constructor**
- `setMaxNumberOfStudents`
- `addStudent`
- `addCourse` (takes 2 arguments)
- `dropCourse` (takes 2 arguments)
- `toString`
#### 4. Create an Inner Class `Student` Inside `Students` Class
Design an inner class `Student` within the `Students` class with the following methods:
- **Default Constructor**
- **Overloaded Constructor**
- `setID`
- `setCourses`
- `getID`
- `getCourses`
- `addCourse` (takes 1 argument)
- `dropCourse` (takes 1 argument)
- `toString`
#### 5. Create a `StudentsDemo` Class by Adding Students with IDs: 1111, 1234, 2357
- **Display the Following Menu:**
```
"What action would you like to implement?"
1: Show all Students
2: Add a Course
3: Drop a Course
9: Quit
```
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
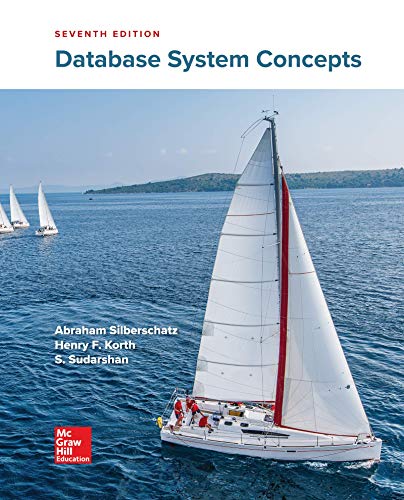
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
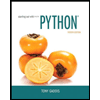
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
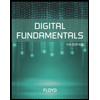
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
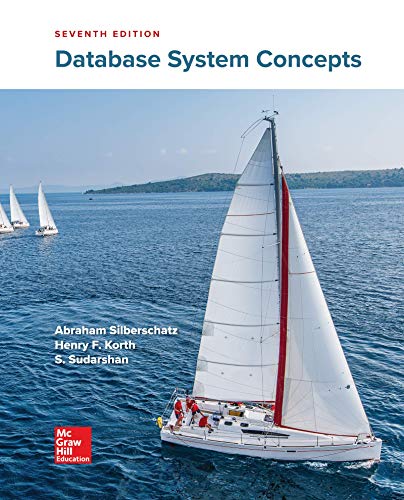
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
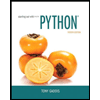
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
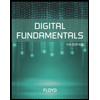
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
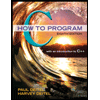
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
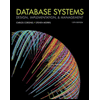
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
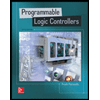
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education