1 import numpy as np 2 from Interfaces import List 3 4 v class ArrayList(List): 5 ... 6 Arraylist: Implementation of a List interface using Arrays. 7 ... 8 v def _init_(self): ... init: Initialize the state (array, n and j). 10 11 12 self.n = e 13 self.j = 0 14 self.a = self.new_array (1) 15 16 v def new array (self, n : int) ->np.array: 17 ... 18 new_array: Create a new array of size n 19 Input: 20 n: the size of the new array 21 Return: 22 An array of size n 23 ... 24 return np.zeros (n, np.object) 25 26 v def resize (self): 27 ... 28 resize: Create a new array and copy the old values. 29 ... 30 pass 31 32 v def get (self, i : int) -> object: 33 get: returns the element at position i Inputs: i: Index that is integer non negative and at most n 34 35 36 37 ... 38 pass 39 40 v def set(self, i: int, x : object) -> object: 41 42 set: Set the value x at the index i 43 Inputs: 44 i: Index that is integer non negative and at most n 45 x: Object type, i.e., any object 46 47 pass 48 49 v def append (self, x : object) : 50 self.add (self.n, x) 51 52 v def add (self, i : int, x : object) : 53 ... 54 add: shift one position all the items j>=i, insert an element at position_ i of the list and increment the number of elements in the list 55 56 57 Inputs: i: Index that is integer non negative and at most n x: Object type, i.e., any object 58 59 60 ... 61 pass 62 63 v def remove (self, i : int) -> object: 64 pass 65 66 v def size(self) -> int: 67 return self.n 68 69 v def str_(self): 70 for i in range (0, self.n): s += "%r" % self.a[(i + self.j) % len (self.a)) if i < self.n-1: 71 v 72 73 v 74 75 return s + *]*
1 import numpy as np 2 from Interfaces import List 3 4 v class ArrayList(List): 5 ... 6 Arraylist: Implementation of a List interface using Arrays. 7 ... 8 v def _init_(self): ... init: Initialize the state (array, n and j). 10 11 12 self.n = e 13 self.j = 0 14 self.a = self.new_array (1) 15 16 v def new array (self, n : int) ->np.array: 17 ... 18 new_array: Create a new array of size n 19 Input: 20 n: the size of the new array 21 Return: 22 An array of size n 23 ... 24 return np.zeros (n, np.object) 25 26 v def resize (self): 27 ... 28 resize: Create a new array and copy the old values. 29 ... 30 pass 31 32 v def get (self, i : int) -> object: 33 get: returns the element at position i Inputs: i: Index that is integer non negative and at most n 34 35 36 37 ... 38 pass 39 40 v def set(self, i: int, x : object) -> object: 41 42 set: Set the value x at the index i 43 Inputs: 44 i: Index that is integer non negative and at most n 45 x: Object type, i.e., any object 46 47 pass 48 49 v def append (self, x : object) : 50 self.add (self.n, x) 51 52 v def add (self, i : int, x : object) : 53 ... 54 add: shift one position all the items j>=i, insert an element at position_ i of the list and increment the number of elements in the list 55 56 57 Inputs: i: Index that is integer non negative and at most n x: Object type, i.e., any object 58 59 60 ... 61 pass 62 63 v def remove (self, i : int) -> object: 64 pass 65 66 v def size(self) -> int: 67 return self.n 68 69 v def str_(self): 70 for i in range (0, self.n): s += "%r" % self.a[(i + self.j) % len (self.a)) if i < self.n-1: 71 v 72 73 v 74 75 return s + *]*
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
![1
import numpy as np
from Interfaces import List
3
4 v class ArrayList (List):
5
6
ArrayList: Implementation of a List interface using Arrays.
7
8 v
def _init_ (self):
9
10
-_init_: Initialize the state (array, n and j).
11
12
self.n = e
self.j = e
self.a = self.new_array (1)
13
14
15
16 v
def new_array (self, n : int) ->np.array:
17
18
new_array: Create a new array of size n
Input:
19
20
n: the size of the new array
21
Return:
22
An array of size n
23
24
return np.zeros (n, np.object)
25
26 v
def resize(self):
27
28
resize
Create a new array and copy the old values.
29
30
pass
31
32 y
def get (self, i : int) -> object:
33
34
get: returns the element at position i
35
Inputs:
36
i: Index that is integer non negative and at most n
37
38
pass
39
40 v
def set (self, i : int, x : object) -> object:
41
42
set: Set the value x at the indexi.
43
Inputs:
44
i: Index that is integer non negative and at most n
45
x: Object type, i.e., any object
46
47
pass
48
49
def append (self, x : object) :
y
50
self.add (self.n, x)
51
52 v
def add (self, i : int, x : object) :
53
54
add: shift one position all the items j>=i, insert an element
55
at position_i of the list and increment the number of elements
56
in the list
57
Inputs:
i: Index that is integer non negative and at most n
x: Object type, i.e., any object
58
59
60
...
61
pass
62
63 v
def remove (self, i : int) -> object:
64
pass
65
66 v
def size(self) -> int:
67
return self.n
68
69 v
def str_(self):
70
for i in range (0, self.n):
s += "%r" % self.a[ (i + self.j) % len (self.a)]
if i < self.n-1:
71 v
72
73 y
74
s +=","
75
return s +)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F5cc32607-3a96-40ee-84c8-a275278c96cb%2F638f3181-5ef6-479c-bb13-3df22bd6af7f%2F84cudpf_processed.png&w=3840&q=75)
Transcribed Image Text:1
import numpy as np
from Interfaces import List
3
4 v class ArrayList (List):
5
6
ArrayList: Implementation of a List interface using Arrays.
7
8 v
def _init_ (self):
9
10
-_init_: Initialize the state (array, n and j).
11
12
self.n = e
self.j = e
self.a = self.new_array (1)
13
14
15
16 v
def new_array (self, n : int) ->np.array:
17
18
new_array: Create a new array of size n
Input:
19
20
n: the size of the new array
21
Return:
22
An array of size n
23
24
return np.zeros (n, np.object)
25
26 v
def resize(self):
27
28
resize
Create a new array and copy the old values.
29
30
pass
31
32 y
def get (self, i : int) -> object:
33
34
get: returns the element at position i
35
Inputs:
36
i: Index that is integer non negative and at most n
37
38
pass
39
40 v
def set (self, i : int, x : object) -> object:
41
42
set: Set the value x at the indexi.
43
Inputs:
44
i: Index that is integer non negative and at most n
45
x: Object type, i.e., any object
46
47
pass
48
49
def append (self, x : object) :
y
50
self.add (self.n, x)
51
52 v
def add (self, i : int, x : object) :
53
54
add: shift one position all the items j>=i, insert an element
55
at position_i of the list and increment the number of elements
56
in the list
57
Inputs:
i: Index that is integer non negative and at most n
x: Object type, i.e., any object
58
59
60
...
61
pass
62
63 v
def remove (self, i : int) -> object:
64
pass
65
66 v
def size(self) -> int:
67
return self.n
68
69 v
def str_(self):
70
for i in range (0, self.n):
s += "%r" % self.a[ (i + self.j) % len (self.a)]
if i < self.n-1:
71 v
72
73 y
74
s +=","
75
return s +)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
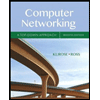
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
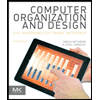
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
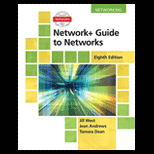
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
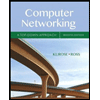
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
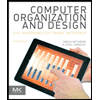
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
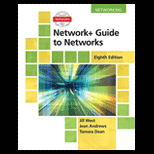
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
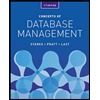
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
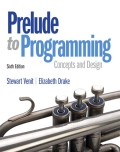
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
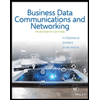
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY