public abstract class Shape { public Shape() { } public abstract double area() ; public String who() { return "Shape" ; } } public class Circle extends Shape { private int radius ; public Circle( int r ) { radius = r ; } @Override public double area() { return Math.PI * radius * radius ; } } public class Square extends Shape { private int _sideLength ; public Square( int sideLength ) { /* TODO question 45 */ } @Override public double area() { return _sideLength * _sideLength ; } } Implement the Square constructor, and make sure it throws an exception when the input parameter is not a valid length. The input parameter must be positive to be considered valid
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
public abstract class Shape {
public Shape() { }
public abstract double area() ;
public String who() { return "Shape" ; }
}
public class Circle extends Shape {
private int radius ;
public Circle( int r )
{
radius = r ;
}
@Override
public double area()
{
return Math.PI * radius * radius ;
}
}
public class Square extends Shape {
private int _sideLength ;
public Square( int sideLength )
{
/* TODO question 45 */
}
@Override
public double area()
{
return _sideLength * _sideLength ;
}
}
Implement the Square constructor, and make sure it throws an exception when the input parameter is not a valid length. The input parameter must be positive to be considered valid.

Step by step
Solved in 3 steps

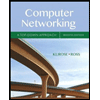
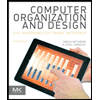
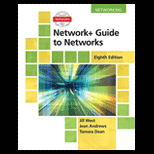
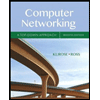
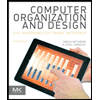
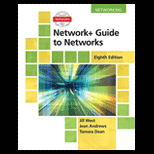
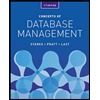
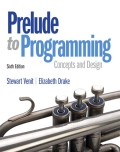
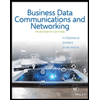