1. An abstract class called Pet is defined below: public abstract class Pet { private String id; private String name; private static int count = 0; public Pet(String name) { count++; id = "P" + count; this.name = name; } public String toString() { return "ID: " + id + ", Name: " + name; } abstract public void sound(); } The Cat class is a subclass of the Pet class. The class specifications are given below: • It has a private field named age to record the cat’s age. • The class constructor sets both name and age fields of the cat with the given parameter values. The field age is set to 0 if the given value is negative. • It provides an accessor method for the field age. • It overwrites the toString method to return a string consisting of the cat’s ID, name and age. e.g. ID: P15, Name: Molly, Age: 4 • It prints out "Miaow" in a termina
1. An abstract class called Pet is defined below:
public abstract class Pet
{
private String id;
private String name;
private static int count = 0;
public Pet(String name)
{
count++;
id = "P" + count;
this.name = name;
}
public String toString()
{
return "ID: " + id + ", Name: " + name;
}
abstract public void sound();
}
The Cat class is a subclass of the Pet class. The class specifications are given below:
• It has a private field named age to record the cat’s age.
• The class constructor sets both name and age fields of the cat with the given parameter
values. The field age is set to 0 if the given value is negative.
• It provides an accessor method for the field age.
• It overwrites the toString method to return a string consisting of the cat’s ID, name
and age. e.g.
ID: P15, Name: Molly, Age: 4
• It prints out "Miaow" in a terminal when the method sound is called.
Define the Cat class in full.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

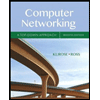
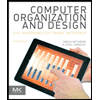
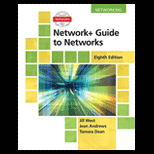
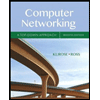
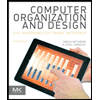
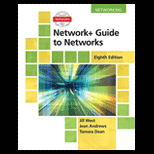
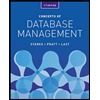
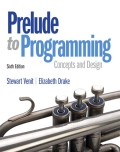
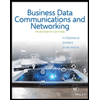