Draw a UML class diagram for the following code: public class ArrayQueue { private Object[] array; private int front; private int rear; private int size; public ArrayQueue(int capacity) { array = new Object[capacity]; front = 0; rear = -1; size = 0; } public boolean isEmpty() { return size == 0; } public void enqueue(T item) { if (size == array.length) { throw new IllegalStateException("Queue is full"); } rear = (rear + 1) % array.length; array[rear] = item; size++; } public T dequeue() { if (isEmpty()) { throw new IllegalStateException("Queue is empty"); } T item = (T) array[front]; array[front] = null; front = (front + 1) % array.length; size--; return item; } public void dequeueAll() { while (!isEmpty()) { dequeue(); } } public T peek() { if (isEmpty()) { throw new IllegalStateException("Queue is empty"); } return (T) array[front]; } public static void main(String[] args) { ArrayQueue queue = new ArrayQueue<>(5); System.out.println("Is Queue Empty? " + queue.isEmpty()); queue.enqueue(10); queue.enqueue(20); queue.enqueue(30); System.out.println("Front item: " + queue.peek()); System.out.println("Dequeuing: " + queue.dequeue()); System.out.println("Dequeuing: " + queue.dequeue()); System.out.println("Is Queue Empty? " + queue.isEmpty()); queue.enqueue(40); queue.enqueue(50); System.out.println("Dequeuing all:"); queue.dequeueAll(); System.out.println("Is Queue Empty? " + queue.isEmpty()); } }
Draw a UML class diagram for the following code:
public class ArrayQueue<T> {
private Object[] array;
private int front;
private int rear;
private int size;
public ArrayQueue(int capacity) {
array = new Object[capacity];
front = 0;
rear = -1;
size = 0;
}
public boolean isEmpty() {
return size == 0;
}
public void enqueue(T item) {
if (size == array.length) {
throw new IllegalStateException("Queue is full");
}
rear = (rear + 1) % array.length;
array[rear] = item;
size++;
}
public T dequeue() {
if (isEmpty()) {
throw new IllegalStateException("Queue is empty");
}
T item = (T) array[front];
array[front] = null;
front = (front + 1) % array.length;
size--;
return item;
}
public void dequeueAll() {
while (!isEmpty()) {
dequeue();
}
}
public T peek() {
if (isEmpty()) {
throw new IllegalStateException("Queue is empty");
}
return (T) array[front];
}
public static void main(String[] args) {
ArrayQueue<Integer> queue = new ArrayQueue<>(5);
System.out.println("Is Queue Empty? " + queue.isEmpty());
queue.enqueue(10);
queue.enqueue(20);
queue.enqueue(30);
System.out.println("Front item: " + queue.peek());
System.out.println("Dequeuing: " + queue.dequeue());
System.out.println("Dequeuing: " + queue.dequeue());
System.out.println("Is Queue Empty? " + queue.isEmpty());
queue.enqueue(40);
queue.enqueue(50);
System.out.println("Dequeuing all:");
queue.dequeueAll();
System.out.println("Is Queue Empty? " + queue.isEmpty());
}
}

Step by step
Solved in 3 steps with 1 images

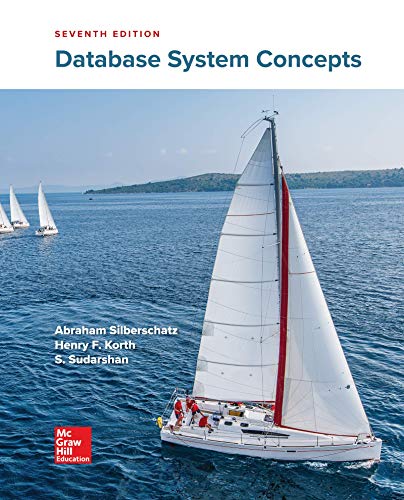
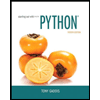
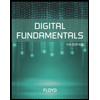
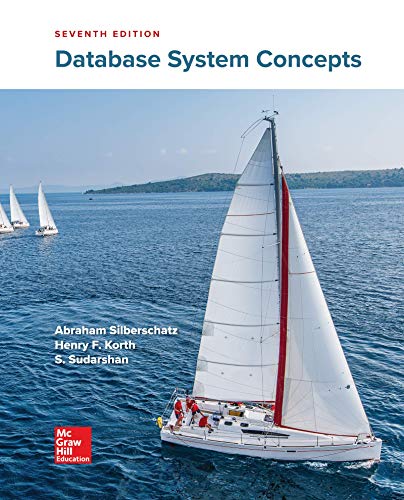
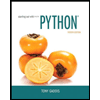
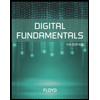
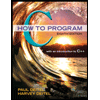
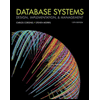
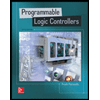