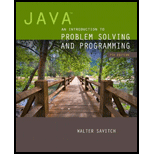
Java: An Introduction to Problem Solving and Programming (7th Edition)
7th Edition
ISBN: 9780133766264
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 9, Problem 9PP
Program Plan Intro
Lap Timer using Applet
Program Plan:
Filename: “LapTimerApplication.java”
- Import the required packages.
- Declare the class “LapTimerApplication”.
- Declare the required variables.
- Define the constructor.
- Set the window size
- Close the window.
- Create an object for “Container” class.
- Initially set the background color as white.
- Set the layout.
- Create the buttons.
- Add the action to these buttons.
- Add the buttons on the window screen.
- Create the object for the labels.
- Add the label on the window screen.
- Create an object for the “LapTimer” class.
- Declare the “actionPerformed” method.
- Declare the required variables with the values.
- If the action command is start, then execute the “if” condition.
- Inside the “try” block, call the method “start ()” and set the labels.
- In catch block, set the error message.
- If the action command is lap, then execute the “if” condition.
- Inside the “try” block, call the method “markLap ()” and set the labels.
- In catch block, set the error message.
- Declare the “main ()” method.
- Create an object for “LapTimer” class.
- Show the window.
Filename: “LapTimer.java”
- Import required package
- Define the class “LapTimer”.
- Declare required private variables “running”, “startTime”, “lapStart”, “lapTime”, “totalTime”, “lapsCompleted”, “lapsInRace”.
- Define the constructor.
- Assign the values.
- Define the method “start ()”.
- Check the condition “running == true || lapsCompleted > 0”.
- Throw “TimerException” exception with a message.
- Assign “true” to the variable “running”.
- Calculate the value of “startTime”.
- Assign the value of “startTime” to “lapStart”.
- Throw “TimerException” exception with a message.
- Check the condition “running == true || lapsCompleted > 0”.
- Define the method “markLap ()”.
- Check if value of “running” is “false”.
- Check if laps completed are equal to 0.
- Throw “”TimerException exception with a message.
- Increment the value of “lapsCompleted”.
- Get the current time and store it in a variable “currentTime”.
- Calculate “lapTime” by subtracting “lapStart” from “currentTime”.
- Calculate “totalTime” by subtracting “startTime” from “currentTime”.
- Assign the value of “currentTime” to “lapStart”.
- Check if the value of “lapsCompleted” and “lapsInRace” are equal.
- Assign “false” to “running”.
- Check if laps completed are equal to 0.
- Check if value of “running” is “false”.
- Define the method “getLapTime ()”.
- Check if “lapsCompleted” is 0.
- Throw “”TimerException exception with a message.
- Return “lapTime”.
- Throw “”TimerException exception with a message.
- Check if “lapsCompleted” is 0.
- Define the method “getTotalTime ()”.
- Check if “lapsCompleted” is 0.
- Throw “”TimerException exception with a message.
- Return “totalTime”.
- Throw “”TimerException exception with a message.
- Check if “lapsCompleted” is 0.
- Define the method “getLapsRemaining ()”.
- Return the value.
- Define the “main ()” function.
- Declare a variable and assign it with a value.
- Create an object for the class “LapTimer”.
- Call the function “start ()”.
- Call the function “markLap ()”.
- Print the number of laps.
- Print the lap time by calling the function “getLapTime ()”.
- Print the total time by calling the function “getTotalTime ()”.
- Print the remaining number of laps by calling the function “getLapsRemaining ()”.
Filename: “TimerException.java”
- Define an exception class named “TimerException”.
- Define a parameterized constructor for the class.
- Call the parent class’s method by passing a message.
- Define a parameterized constructor for the class.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Capsim Team PowerPoint Presentations - Slide Title: Key LearningsWhat were the key learnings that you discovered as a team through your Capsim simulation?
Write the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.
Draw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Ch. 9.1 - Prob. 1STQCh. 9.1 - What output would the code in the previous...Ch. 9.1 - Prob. 3STQCh. 9.1 - Prob. 4STQCh. 9.1 - Prob. 5STQCh. 9.1 - Prob. 6STQCh. 9.1 - Prob. 7STQCh. 9.1 - Prob. 8STQCh. 9.1 - In the code given in Self-Test Question 1,...Ch. 9.1 - In the code given in Self-Test Question 1,...
Ch. 9.1 - Prob. 11STQCh. 9.1 - Prob. 12STQCh. 9.1 - Prob. 13STQCh. 9.1 - Prob. 14STQCh. 9.2 - Prob. 15STQCh. 9.2 - Prob. 16STQCh. 9.2 - Prob. 17STQCh. 9.2 - Prob. 18STQCh. 9.2 - Prob. 19STQCh. 9.2 - Prob. 20STQCh. 9.2 - Suppose that, in Self-Test Question 19, we change...Ch. 9.2 - Prob. 22STQCh. 9.2 - Prob. 23STQCh. 9.3 - Prob. 24STQCh. 9.3 - Prob. 25STQCh. 9.3 - Prob. 26STQCh. 9.3 - Prob. 27STQCh. 9.3 - Prob. 28STQCh. 9.3 - Repeat Self-Test Question 27, but change the value...Ch. 9.3 - Prob. 30STQCh. 9.3 - Prob. 31STQCh. 9.3 - Prob. 32STQCh. 9.3 - Consider the following program: a. What output...Ch. 9.3 - Write an accessor method called getPrecision that...Ch. 9.3 - Prob. 35STQCh. 9.4 - Rewrite the class ColorDemo in Listing 9.13 so...Ch. 9.4 - Prob. 37STQCh. 9 - Write a program that allows students to schedule...Ch. 9 - Prob. 2ECh. 9 - Prob. 3ECh. 9 - Prob. 4ECh. 9 - Prob. 5ECh. 9 - Write code that reads a string from the keyboard...Ch. 9 - Create a class Rational that represents a rational...Ch. 9 - Prob. 9ECh. 9 - Suppose that you are going to create an object...Ch. 9 - Revise the class RoomCounter described in the...Ch. 9 - Prob. 12ECh. 9 - Write a class LapTimer that can be used to time...Ch. 9 - Prob. 1PCh. 9 - Prob. 2PCh. 9 - Prob. 3PCh. 9 - Write a program that uses the class calculator in...Ch. 9 - Prob. 3PPCh. 9 - Prob. 7PPCh. 9 - Prob. 9PPCh. 9 - Suppose that you are in change of customer service...Ch. 9 - Write an application that implements a trip-time...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.arrow_forward2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forward
- hello please explain the architecture in the diagram below. thanks youarrow_forwardComplete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forward
- Hello please look at the attached picture. I need an detailed explanation of the architecturearrow_forwardInformation Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
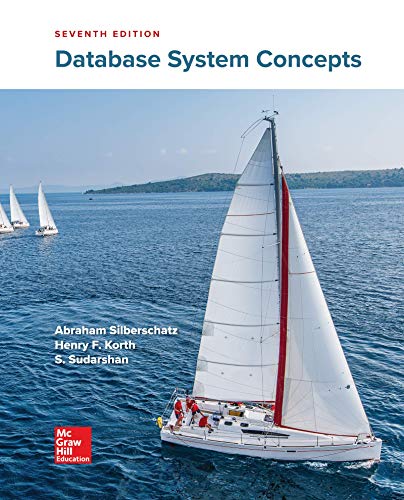
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
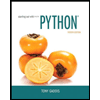
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
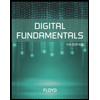
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
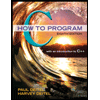
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
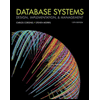
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
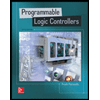
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
6 Stages of UI Design; Author: DesignerUp;https://www.youtube.com/watch?v=_6Tl2_eM0DE;License: Standard Youtube License