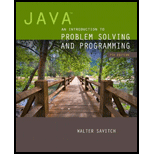
Java: An Introduction to Problem Solving and Programming (7th Edition)
7th Edition
ISBN: 9780133766264
Author: Walter Savitch
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 9, Problem 3PP
Program Plan Intro
Date format converter
Program plan:
“DateFormatConverter.java”:
- Import required packages.
- Define the class “DateFormatConverter”.
- Declare required variables.
- Define an array and assign it with the months.
- Define the “main()” method.
- Do until the user enters other than “Y” or “y”.
- Inside the “try” block,
- Get the date from the user.
- Trim the input.
- Store the month value in a variable.
- Check if that value is less than 0.
-
- Assign “false” to “validInput”.
- Throw an exception.
- Check if month value is less than 1 or greater than 12.
-
- Assign “false” to “validInput”.
- Throw an exception.
- Otherwise,
-
- Get the date value and store it in a variable.
- Call the function “dayCheck()”.
- Catch “MonthException”.
- Print the error message.
- Check if the function “validInput()” returns true.
- Print the month name and its date.
- Get the response from the user whether the user wants to do it again or not.
- Inside the “try” block,
- Do until the user enters other than “Y” or “y”.
- Give function to convert string to integer for month.
- Get the position of “/”.
- Check if that position is equal to 2.
- Return the value.
- Check if that position is equal to 1.
- Return the integer value.
- Otherwise,
- Return -1.
- Give function to convert string to integer for day.
- Get the number of characters.
- Check if that number is equal to 2.
- Return the value.
- Check if that number is equal to 1.
- Return the integer value.
- Otherwise,
- Return -1.
- Function definition to convert ASCII value to integer.
- Switch to the digit.
- Assign the value according to the character digit.
- Switch to the digit.
- Give function definition to check the day.
- Inside the “try” block,
- Switch to the month number.
- If the month number is 1, 3, 5, 7, 8, 10 and 12,
-
- Check if the day is less than 1 or greater than 31.
- Assign “false” to “validInput”.
- Print the error message.
- Otherwise,
- Assign “true” to “validInput”.
- Break the case.
- Otherwise,
- Check if the day is less than 1 or greater than 31.
- If the month number is 4, 6, 9 and 11,
-
- Check if the day is less than 1 or greater than 30.
- Assign “false” to “validInput”.
- Print the error message.
- Otherwise,
- Assign “true” to “validInput”.
- Break the case.
- Otherwise,
- Check if the day is less than 1 or greater than 30.
- If the month number is 2,
-
- Check if the day is less than 1 or greater than 29.
- Assign “false” to “validInput”.
- Print the error message.
- Otherwise,
- Assign “true” to “validInput”.
- Break the case.
- Otherwise,
- Check if the day is less than 1 or greater than 29.
- Switch to the month number.
- Inside the “catch” block for “DayException”, print the error message.
- Inside the “try” block,
“DayException.java”:
- Define the exception class “DayException”.
- Define a default constructor.
- Call the parent class’s method.
- Define two parameterized constructors.
- Call the parent class’s method by passing a message.
- Define a default constructor.
“MonthException.java”:
- Define the exception class “MonthException”.
- Define a default constructor.
- Call the parent class’s method.
- Define two parameterized constructors.
- Call the parent class’s method by passing a message.
- Define a default constructor.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
using r language
8. Cash RegisterThis exercise assumes you have created the RetailItem class for Programming Exercise 5. Create a CashRegister class that can be used with the RetailItem class. The CashRegister class should be able to internally keep a list of RetailItem objects. The class should have the following methods:
A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list.
A method named get_total that returns the total price of all the RetailItem objects stored in the CashRegister object’s internal list.
A method named show_items that displays data about the RetailItem objects stored in the CashRegister object’s internal list.
A method named clear that should clear the CashRegister object’s internal list.
Demonstrate the CashRegister class in a program that allows the user to select several items for purchase. When the user is ready to check out, the…
5. RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price.
Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them:
Description Units in Inventory PriceItem #1 Jacket 12 59.95Item #2 Designer Jeans 40 34.95Item #3 Shirt 20 24.95
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
Ch. 9.1 - Prob. 1STQCh. 9.1 - What output would the code in the previous...Ch. 9.1 - Prob. 3STQCh. 9.1 - Prob. 4STQCh. 9.1 - Prob. 5STQCh. 9.1 - Prob. 6STQCh. 9.1 - Prob. 7STQCh. 9.1 - Prob. 8STQCh. 9.1 - In the code given in Self-Test Question 1,...Ch. 9.1 - In the code given in Self-Test Question 1,...
Ch. 9.1 - Prob. 11STQCh. 9.1 - Prob. 12STQCh. 9.1 - Prob. 13STQCh. 9.1 - Prob. 14STQCh. 9.2 - Prob. 15STQCh. 9.2 - Prob. 16STQCh. 9.2 - Prob. 17STQCh. 9.2 - Prob. 18STQCh. 9.2 - Prob. 19STQCh. 9.2 - Prob. 20STQCh. 9.2 - Suppose that, in Self-Test Question 19, we change...Ch. 9.2 - Prob. 22STQCh. 9.2 - Prob. 23STQCh. 9.3 - Prob. 24STQCh. 9.3 - Prob. 25STQCh. 9.3 - Prob. 26STQCh. 9.3 - Prob. 27STQCh. 9.3 - Prob. 28STQCh. 9.3 - Repeat Self-Test Question 27, but change the value...Ch. 9.3 - Prob. 30STQCh. 9.3 - Prob. 31STQCh. 9.3 - Prob. 32STQCh. 9.3 - Consider the following program: a. What output...Ch. 9.3 - Write an accessor method called getPrecision that...Ch. 9.3 - Prob. 35STQCh. 9.4 - Rewrite the class ColorDemo in Listing 9.13 so...Ch. 9.4 - Prob. 37STQCh. 9 - Write a program that allows students to schedule...Ch. 9 - Prob. 2ECh. 9 - Prob. 3ECh. 9 - Prob. 4ECh. 9 - Prob. 5ECh. 9 - Write code that reads a string from the keyboard...Ch. 9 - Create a class Rational that represents a rational...Ch. 9 - Prob. 9ECh. 9 - Suppose that you are going to create an object...Ch. 9 - Revise the class RoomCounter described in the...Ch. 9 - Prob. 12ECh. 9 - Write a class LapTimer that can be used to time...Ch. 9 - Prob. 1PCh. 9 - Prob. 2PCh. 9 - Prob. 3PCh. 9 - Write a program that uses the class calculator in...Ch. 9 - Prob. 3PPCh. 9 - Prob. 7PPCh. 9 - Prob. 9PPCh. 9 - Suppose that you are in change of customer service...Ch. 9 - Write an application that implements a trip-time...
Knowledge Booster
Similar questions
- Find the Error: class Information: def __init__(self, name, address, age, phone_number): self.__name = name self.__address = address self.__age = age self.__phone_number = phone_number def main(): my_info = Information('John Doe','111 My Street', \ '555-555-1281')arrow_forwardFind the Error: class Pet def __init__(self, name, animal_type, age) self.__name = name; self.__animal_type = animal_type self.__age = age def set_name(self, name) self.__name = name def set_animal_type(self, animal_type) self.__animal_type = animal_typearrow_forwardTask 2: Comparable Interface and Record (10 Points) 1. You are tasked with creating a Java record of Dog (UML is shown below). The dog record should include the dog's name, breed, age, and weight. You are required to implement the Comparable interface for the Dog record so that you can sort the records based on the dogs' ages. Create a Java record named Dog.java. name: String breed: String age: int weight: double + toString(): String > Dog + compareTo(otherDog: Dog): int > Comparable 2. In the Dog record, establish a main method and proceed to generate an array named dogList containing three Dog objects, each with the following attributes: Dog1: name: "Buddy", breed: "Labrador Retriever", age: 5, weight: 25.5 Dog2: name: "Max", breed: "Golden Retriever", age: 3, weight: 30 Dog3: name: "Charlie", breed: "German Shepherd", age: 2, weight: 22 3. Print the dogs in dogList before sorting the dogList by age. (Please check the example output for the format). • 4. Sort the dogList using…arrow_forward
- The OSI (Open Systems Interconnection) model is a conceptual framework that standardises the functions of a telecommunication or computing system into seven distinct layers, facilitating communication and interoperability between diverse network protocols and technologies. Discuss the OSI model's physical layer specifications when designing the physical network infrastructure for a new office.arrow_forwardIn a network, information about how to reach other IP networks or hosts is stored in a device's routing table. Each entry in the routing table provides a specific path to a destination, enabling the router to forward data efficiently across the network. The routing table contains key parameters determining the available routes and how traffic is directed toward its destination. Briefly explain the main parameters that define a routing entry.arrow_forwardYou are troubleshooting a network issue where an employee's computer cannot connect to the corporate network. The computer is connected to the network via an Ethernet cable that runs to a switch. Suspecting a possible layer 1 or layer 2 problem, you decide to check the LED status indicators on both the computer's NIC and the corresponding port on the switch. Describe five LED link states and discuss what each indicates to you as a network technician.arrow_forward
- You are a network expert tasked with upgrading the network infrastructure for a growing company expanding its operations across multiple floors. The new network setup needs to support increased traffic and future scalability and provide flexibility for network management. The company is looking to implement Ethernet switches to connect various devices, including workstations, printers, and IP cameras. As part of your task, you must select the appropriate types of Ethernet switches to meet the company's needs. Evaluate the general Ethernet switch categories you would consider for this project, including their features and how they differ.arrow_forwardYou are managing a Small Office Home Office (SOHO) network connected to the Internet via a fibre link provided by your Internet Service Provider (ISP). Recently, you have noticed a significant decrease in Internet speed, and after contacting your ISP, they confirmed that no issues exist on their end. Considering that the problem may lie within your local setup, identify three potential causes of the slow Internet connection, focusing on physical factors affecting the fibre equipment.arrow_forwardYour organisation has recently installed a new network in a building it has acquired. As the network administrator, you have set up a dedicated telecommunications room to house all the rack-mounted servers, switches, and routers. To ensure optimal performance and longevity of the equipment, you need to monitor certain environmental factors in the room. Identify the environmental factors that should be monitored and explain why each is important to maintain the proper functioning of the telecommunications equipment.arrow_forward
- Your organisation is preparing to move into a newly constructed office building that has never been occupied or wired for network services. As the network administrator, your manager has tasked you with designing a structured cabling plan that will support data, voice, and other network services across all building floors. The cabling plan must account for future expansion, efficient data transmission, and compliance with industry standards. Identify and explain the different subsystems you would include in the structured cabling scheme, following the ANSI/TIA/EIA 568 standard.arrow_forwardAs a technical advisor responsible for designing a network in a newly constructed building, you have decided to utilise twisted pair cables to efficiently deliver data and voice services. Given the specific requirements for connectivity in this setup, identify the appropriate connector types that can be used with twisted pair cables, explaining how each connector works in detail.arrow_forwardIn computer networks, communication between devices or nodes relies on predefined rules known as network protocols. These protocols ensure that data can be transmitted accurately and efficiently between different devices, even on separate networks or running different operating systems. Explain how a network protocol facilitates the transmission of data between nodes.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
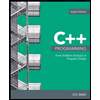
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
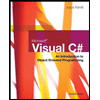
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
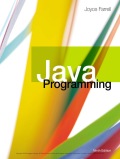
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
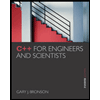
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
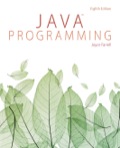
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT