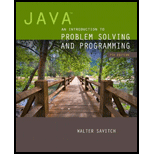
Explanation of Solution
Exception classes:
The two classes that derives “InvalidFormattingException” are “InvalidHourException” and “InvalidMinuteException”.
InvalidHourException.java:
//Create a class that extends Exception
public class InvalidHourException extends InvalidFormattingException
{
//Define a parameterized constructor
public InvalidHourException(String reason)
{
//Call the parent class's method by passing a message
super(reason);
}
}
Explanation:
The above class “InvalidHourException” extends “InvalidFormattingException” and this exception occurs when the hour entered by the user in invalid.
InvalidMinuteException.java:
//Create a class that extends Exception
public class InvalidMinuteException extends InvalidFormattingException
{
//Define a parameterized constructor
public InvalidMinuteException(String reason)
{
//Call the parent class's method by passing a message
super(reason);
}
}
Explanation:
The above class “InvalidMinuteException” extends “InvalidFormattingException” and this exception occurs when the minute entered by the user in invalid.
Complete program:
Main.java:
//Import required packages
import java.util.*;
import java.util.Scanner;
//Define the main class
class Main
{
//Declare required variables
private int hour, minute;
private boolean isAM;
//Constructor
public TimeOfDay()
{
//Instantiate the values
hour = 0;
minute = 0;
isAM = false;
}
//Function definition to set the time
public void setTimeTo(String aTime) throws InvalidFormattingException, InvalidHourException, InvalidMinuteException
{
//Declare required variables
int hourFound;
int minuteFound;
String indicatorFound;
//Create an object for the scanner class
Scanner reader = new Scanner(aTime);
//Split using the delimiter
reader.useDelimiter(":");
//Try block
try
{
//Assign the hour
hourFound = reader.nextInt();
}
//Catch the exception
catch (Exception e)
{
//Throw the exception with a message
throw new InvalidFormattingException("Hour not an integer");
}
//Check the condition
if(hourFound<1 || hourFound>12)
//Throw the exception with a message
throw new InvalidHourException("Hour not in the range of 1 to 12");
//Get the remaining string
String restOfString = reader.next();
reader = new Scanner(restOfString);
//Remove the last two characters
if(restOfString.length()<3)
//Throw the exception
throw new InvalidFormattingException("Bad format");
//Get the substring
String minuteString = restOfString.substring(0, restOfString.length()-2);
//Get the substring
String amString = restOfString.substring(restOfString.length()-2);
//Try block
try
{
//Convert the minute to integer
minuteFound = Integer.parseInt(minuteString);
}
//Catch the exception
catch (Exception e)
{
//Throw the exception
throw new InvalidFormattingException("Minute not an integer");
}
//Check the condition
if(minuteFound<0 || minuteFound>59)
//Throw the exception with a message
throw new InvalidMinuteException("Minute not in the range of 0 to 59");
//Check condition
if(!amString.equals("am") && !amString.equals("pm"))
//Throw the exception with a message
throw new InvalidFormattingException("Did not have am/pm");
//Assign the value
hour = hourFound;
//Assign the value
minute = minuteFound;
//Check if the string is am
if(amString...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
- This week we will be building a regression model conceptually for our discussion assignment. Consider your current workplace (or previous/future workplace if not currently working) and answer the following set of questions. Expand where needed to help others understand your thinking: What is the most important factor (variable) that needs to be predicted accurately at work? Why? Justify its selection as your dependent variable.arrow_forwardAccording to best practices, you should always make a copy of a config file and add a date to filename before editing? Explain why this should be done and what could be the pitfalls of not doing it.arrow_forwardIn completing this report, you may be required to rely heavily on principles relevant, for example, the Work System, Managerial and Functional Levels, Information and International Systems, and Security. apply Problem Solving Techniques (Think Outside The Box) when completing. should reflect relevance, clarity, and organisation based on research. Your research must be demonstrated by Details from the scenario to support your analysis, Theories from your readings, Three or more scholarly references are required from books, UWIlinc, etc, in-text or narrated citations of at least four (4) references. “Implementation of an Integrated Inventory Management System at Green Fields Manufacturing” Green Fields Manufacturing is a mid-sized company specialising in eco-friendly home and garden products. In recent years, growing demand has exposed the limitations of their fragmented processes and outdated systems. Different departments manage production schedules, raw material requirements, and…arrow_forward
- 1. Create a Book record that implements the Comparable interface, comparing the Book objects by year - title: String > - author: String - year: int Book + compareTo(other Book: Book): int + toString(): String Submit your source code on Canvas (Copy your code to text box or upload.java file) > Comparable 2. Create a main method in Book record. 1) In the main method, create an array of 2 objects of Book with your choice of title, author, and year. 2) Sort the array by year 3) Print the object. Override the toString in Book to match the example output: @Javadoc Declaration Console X Properties Book [Java Application] /Users/kuan/.p2/pool/plugins/org.eclipse.justj.openjdk.hotspo [Book: year=1901, Book: year=2010]arrow_forwardQ5-The efficiency of a 200 KVA, single phase transformer is 98% when operating at full load 0.8 lagging p.f. the iron losses in the transformer is 2000 watt. Calculate the i) Full load copper losses ii) half load copper losses and efficiency at half load. Ans: 1265.306 watt, 97.186%arrow_forward2. Consider the following pseudocode for partition: function partition (A,L,R) pivotkey = A [R] t = L for i L to R-1 inclusive: if A[i] A[i] t = t + 1 end if end for A [t] A[R] return t end function Suppose we call partition (A,0,5) on A=[10,1,9,2,8,5]. Show the state of the list at the indicated instances. Initial A After i=0 ends After 1 ends After i 2 ends After i = 3 ends After i = 4 ends After final swap 10 19 285 [12 pts]arrow_forward
- .NET Interactive Solving Sudoku using Grover's Algorithm We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules: •No column may contain the same value twice •No row may contain the same value twice If we assign each square in our sudoku to a variable like so: 1 V V₁ V3 V2 we want our circuit to output a solution to this sudoku. Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the conversion of classical decision problems into oracles for Grover's algorithm. Turning the Problem into a Circuit We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit that…arrow_forwardusing r languagearrow_forward8. Cash RegisterThis exercise assumes you have created the RetailItem class for Programming Exercise 5. Create a CashRegister class that can be used with the RetailItem class. The CashRegister class should be able to internally keep a list of RetailItem objects. The class should have the following methods: A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list. A method named get_total that returns the total price of all the RetailItem objects stored in the CashRegister object’s internal list. A method named show_items that displays data about the RetailItem objects stored in the CashRegister object’s internal list. A method named clear that should clear the CashRegister object’s internal list. Demonstrate the CashRegister class in a program that allows the user to select several items for purchase. When the user is ready to check out, the…arrow_forward
- 5. RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description Units in Inventory PriceItem #1 Jacket 12 59.95Item #2 Designer Jeans 40 34.95Item #3 Shirt 20 24.95arrow_forwardFind the Error: class Information: def __init__(self, name, address, age, phone_number): self.__name = name self.__address = address self.__age = age self.__phone_number = phone_number def main(): my_info = Information('John Doe','111 My Street', \ '555-555-1281')arrow_forwardFind the Error: class Pet def __init__(self, name, animal_type, age) self.__name = name; self.__animal_type = animal_type self.__age = age def set_name(self, name) self.__name = name def set_animal_type(self, animal_type) self.__animal_type = animal_typearrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
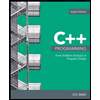
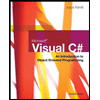
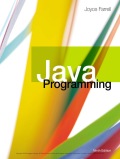
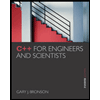
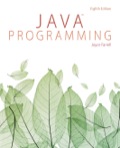