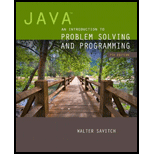
Explanation of Solution
Creating “Main.java”:
- Create a class named “Main”.
- Define the “main ()” method.
- Declare required variables.
- Do till the user enters “n” using “while” condition.
- Get the string from the user.
- Check if the length of the string is greater than or equal to 20.
- Create an object “e” for the class. Here the exception is thrown using default constructor.
- Get and print the message using the method “e.getMessage()”.
- Else,
- Print the number of characters.
- Ask whether the user wants to continue or not and store the response in a variable “response”.
- Check if the response is equal to “n”.
- Break the loop.
- Define the “main ()” method.
Creating “MessageTooLongException.java”:
- Create a class named “MessageTooLongException” that extends “Exception”.
- Define a default constructor that calls the parent class’s method using “super ()” by passing a message.
- Define a parameterized constructor that calls the parent class’s method using “super ()” by passing a message that is given as the argument.
Program:
MessageTooLongException.java:
//Define a class
public class MessageTooLongException extends Exception
{
//Default constructor
public MessageTooLongException()
{
//Call the parent class by passing the message
super("Message Too Long!");
}
//Parameterized constructor
public MessageTooLongException(String message)
{
//Call the parent class by passing the message
super(message);
}
}
Main.java:
//import the package
import java.util.Scanner;
//Main class
class Main
{
//Define main method
public static void main(String[] args)
{
//Create an object for the scanner class
Scanner sc = new Scanner(System.in);
//Declare required variables
String str, response = "y";
//Do till the user enters 'n'
while(response...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
- What's wrong with my pseudocode? // The calcDiscountPrice function accepts an item’s price and // the discount percentage as arguments. It uses those // values to calculate and return the discounted price. Function Real calcDiscountPrice(Real price, Real percentage) // Calculate the discount. Declare Real discount = price * percentage // Subtract the discount from the price. Declare Real discountPrice = price – discount // Return the discount price. Return discount End Functionarrow_forwardNeed help converting my pseudocode to python, AND have a flowchart showing everything!The code: Function getScore() // Prompt the user to enter a test score Display "Enter a test score as a percentage (0-100): " Input score // Return the score entered by the user Return scoreEnd Function Function getGPAPoint(Integer score) // Determine GPA point based on the score If score >= 90 Then Return 4.0 Else If score >= 80 Then Return 3.0 Else If score >= 70 Then Return 2.0 Else If score >= 60 Then Return 1.0 Else Return 0.0 End IfEnd Function Function getAverage() // Initialize variables to store the sum of scores and GPA points totalScore = 0 totalGPA = 0.0 // Loop to collect 5 test scores For i = 1 to 5 Do score = getScore() // Call getScore function to get a test score totalScore = totalScore + score // Add score to totalScore gpaPoint = getGPAPoint(score) // Convert…arrow_forwardWhere did I make an error in my pseudocode module???Code:Module main() Call raiseToPower(2, 1.5) End main Module raiseToPower(Real value, Integer power) Declare Real result Set result = value ^ power Display result End raiseToPowerarrow_forward
- I need help writing pseudocode for calculating class score average by putting in 5 test scores, and showing the average from all 5 inputs and the GPA score.Starting with 3 functions outside of a main module. The functions are getScore(), getGPAPoint(Integer score), and getAverage(). Function getscore is an input for a grade as a class percentage. Function getGPAPoint will calculate the score into a GPA point and return as a float (values of 90-100 as 4.0, 80-89 as 3.0, 70-79 as 2.0, 60-69 as 1.0, and anything below 60 as 0.) Function averageGPA will finally make a call to both previous functions when the user inputs numbers 5 times that then calculates the average (add up all the scores, divide by 5) and the average grade alongside displaying the average GPA. End result is a main module that makes a proper call to the averageGPA function and display its results. Need help with this!arrow_forwardPlease original work Why is integration between data collection and business analysis so important to success in an organization that uses business analytics? How can a company that is just starting to use business analytics set up its program for success right from the beginning? Please cite in text references and add weblinksarrow_forwardHow to make a 1 bit adder with CLA?arrow_forward
- I need help writing pseudocode for this function. The following pseudocode statement calls a function named half, which returns a value that is half that of the argument. (Assume both the result and number variables are Real.) Set result = half(number)arrow_forwardNeed help converting my pseudocode to python, AND have a flowchart showing everything!The code:Main Module Call InputModuleEnd Main Module Module InputModule // This module gets input from the user Declare Principal, AnnualRate, Years as Float Output "Enter the Principal amount (P): " Input Principal Output "Enter the Annual Interest Rate (in percentage, e.g., 5 for 5%): " Input AnnualRate Output "Enter the number of Years to repay the loan: " Input Years Call DisplayPayment(Principal, AnnualRate, Years)End Module Module DisplayPayment(Principal, AnnualRate, Years) // This module calculates and displays the monthly payment Declare R, N as Float Declare MonthlyPayment as Float Declare PowerFactor as Float // second local variable // Calculate monthly interest rate R and number of months N Set R = (AnnualRate / 100) / 12 Set N = Years * 12 // Calculate PowerFactor = (1 + R)^N Set PowerFactor = (1 + R) ^ N // Calculate…arrow_forwardWhats wrong with my pseudocode? Where did I make an error?Code: Module main() Declare Real mileage Call getMileage() Display “You’ve drive a total of “, mileage, “ miles End Module Module getMilage() Display “Enter your mileage: “ Input mileage End Modulearrow_forward
- I need help!! Writing a long pseudocode for a modular program that will display the monthly payment on a mortgage. P=Principal amount borrowed (loan)R=Rate of interest computed for each monthN=Number of months to pay back the loan or mortgageThe help I need is creating a module that you can input the principle, rate of percentage, and years to repay the loan, and another module "displaypayment" that accepts the 3 values and calculates the monthly payment needed for the rates. Lastly 2 local variables needed!Equation:Monthly Payment=[(R*(1+R)^N)/((1+R)^N-1)]*Parrow_forwardTwo pseudocode questions I need help with: How do I design a module called findSum that will display the sum of two integer passed by parameter, and a module called findArea that will display the area of a rectangle when passed 2 real values for the length and width of the rectangle?arrow_forwardFor the pseudocode module, what is displayed with the call findValue(1, 4, 2)?Module findValue(Integer a, Integer b, Integer c) Declare Integer value value = b + c - a Display valuearrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
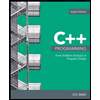
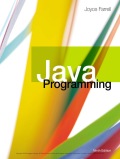
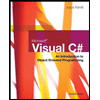
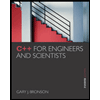
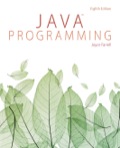