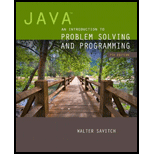
Concept explainers
Explanation of Solution
Exception class:
The exception class to find whether the time entered is valid or not. The time should be in the format “hour:minute” followed by “am” or “pm”.
InvalidFormattingException.java:
//Create a class that extends Exception
public class InvalidFormattingException extends Exception
{
//Define a parameterized constructor
public InvalidFormattingException(String reason)
{
//Call the parent class's method by passing a message
super(reason);
}
}
Explanation:
The above class “InvalidFormattingException” occurs when the time entered by the user in invalid.
Complete program:
Main.java:
//Import required packages
import java.util.*;
import java.util.Scanner;
//Define the main class
class Main
{
//Declare required variables
private int hour, minute;
private boolean isAM;
//Constructor
public Main()
{
//Instantiate the values
hour = 0;
minute = 0;
isAM = false;
}
//Function definition to set the time
public void setTimeTo(String aTime) throws InvalidFormattingException
{
//Declare required variables
int hourFound;
int minuteFound;
String indicatorFound;
//Create an object for the scanner class
Scanner reader = new Scanner(aTime);
//Split using the delimiter
reader.useDelimiter(":");
//Try block
try
{
//Assign the hour
hourFound = reader.nextInt();
}
//Catch the exception
catch (Exception e)
{
//Throw the exception with a message
throw new InvalidFormattingException("Hour not an integer");
}
//Get the remaining string
String restOfString = reader.next();
reader = new Scanner(restOfString);
//Remove the last two characters
if(restOfString.length()<3)
//Throw the exception
throw new InvalidFormattingException("Bad format");
//Get the substring
String minuteString = restOfString.substring(0, restOfString.length()-2);
//Get the substring
String amString = restOfString.substring(restOfString.length()-2);
//Try block
try
{
//Convert the minute to integer
minuteFound = Integer.parseInt(minuteString);
}
//Catch the exception
catch (Exception e)
{
//Throw the exception
throw new InvalidFormattingException("Minute not an integer");
}
//Check condition
if(!amString.equals("am") && !amString.equals("pm"))
//Throw the exception with a message
throw new InvalidFormattingException("Did not have am/pm");
//Assign the value
hour = hourFound;
//Assign the value
minute = minuteFound;
//Check if the string is am
if(amString...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
- use C++ programing language Write a program that prompts the user to enter a person's date of birth in numeric form such as 8-27-1980. The program then outputs the date of birth in the form: August 27, 1980. Your program must contain at least two exception classes: invalidDay and invalidMonth. If the user enters an invalid value for day, then the program should throw and catch an invalidDay object. Similar conventions for the invalid values of month and year. (Note that your program must handle a leap year.)arrow_forwardWrite a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heartrate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get age() with the message "Invalid age." Handle the exception in_main_and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info.arrow_forwardWrite a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info.arrow_forward
- Write a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info. **This is the output i am having trouble with. on line 20 The print string shows the print(ve, 'could not calculate heart rate info.') And i know that the extra space i see at the begining of that second line of output is due to the comma but I cannot figure out how to make the same line print but with out the space from the comma after…arrow_forwardWrite a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in_main_ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info.arrow_forwardWrite a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat_burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info. **This is the output i am having trouble with. on line 20 The print string shows the print(ve, 'could not calculate heart rate info.') And i know that the extra space i see at the begining of that second line of output is due to the comma but I cannot figure out how to make the same line print but with out the space from the comma after…arrow_forward
- Write a program that calculates an adult's fat-burning heart rate, which is 70% of 220 minus the person's age. Complete fat burning_heart_rate() to calculate the fat burning heart rate. The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: If the input is: 17 the output is: Invalid age. Could not calculate heart rate info.arrow_forwardModify the GreenvilleRevenue program created in Chapter 10, Case Study 1 so that it performs the following tasks: The program prompts the user for the number of contestants in this year’s competition; the number must be between 0 and 30. Use exception-handling techniques to ensure a valid value and display the error message:Number must be between 0 and 30 The program prompts the user for talent codes. Use exception-handling techniques to ensure a valid code and update the displayed message to the following message:x is not a valid talent code. Assigned as Invalid.where x was the invalid code entered into the console. After data entry is complete, the program prompts the user for codes so the user can view lists of appropriate contestants. Use exception-handling techniques for the code verification and display the following message: Enter a talent type or Z to quit >> x x is not a valid code and for valid codes: Enter a talent type or Z to quit >> S Contestants with talent…arrow_forwardModify the GreenvilleRevenue program created in Chapter 10, Case Study 1 so that it performs the following tasks: The program prompts the user for the number of contestants in this year’s competition; the number must be between 0 and 30. Use exception-handling techniques to ensure a valid value and display the error message:Number must be between 0 and 30 The program prompts the user for talent codes. Use exception-handling techniques to ensure a valid code and update the displayed message to the following message:x is not a valid talent code. Assigned as Invalid.where x was the invalid code entered into the console. After data entry is complete, the program prompts the user for codes so the user can view lists of appropriate contestants. Use exception-handling techniques for the code verification and display the following message: Enter a talent type or Z to quit >> x x is not a valid code and for valid codes: Enter a talent type or Z to quit >> S Contestants with talent…arrow_forward
- The adult's age must be between the ages of 18 and 75 inclusive. If the age entered is not in this range, raise a ValueError exception in get_age() with the message "Invalid age." Handle the exception in __main__ and print the ValueError message along with "Could not calculate heart rate info." Ex: If the input is: 35 the output is: Fat burning heart rate for a 35 year-old: 129.5 bpm If the input is: 17 the output is: Invalid age. Could not calculate heart rate info.?arrow_forwardDevelop an exception class named InvalidMonthException that extends the Exception class. Instances of the class will be thrown based on the following conditions: The value for a month number is not between 1 and 12 The value for a month name is not January, February, March, … December Develop a class named Month. The class should define an integer field named monthNumber that holds the number of a month. For example, January would be 1, February would be 2, and so forth. In addition, provide the following methods: A no-argument constructor that sets the monthNumberto 1. An overloaded constructor that accepts the number of the month as an argument. The constructor should set the monthNumberfield to the parameter value if the parameter contains the value 1 – 12. Otherwise, throw an InvalidMonthException exception back to the caller. The exception should note that the month number was incorrect. An overloaded constructor that accepts a string containing the name of the month,…arrow_forwardTime Converter 1. Write a program that converts dates from a numerical month-day format to alphabetic month-day format. IE 1/31 or 01/31 would have an output of January 31. 1. User enters the month and day as a single string. It is then converted. (10%) 2. Create 2 exception classes (20%) 1. MonthException thrown for invalid months 2. DayException-thrown for invalid days for the given month 3. You can assume Feb is always 28 days 2. This should run in a for loop and end when a user is done entering dates (70%)arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
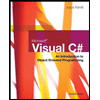
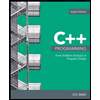