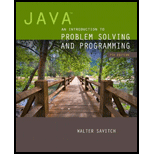
Concept explainers
Explanation of Solution
Creating “TimeOfDay.java”:
- Import required packages.
- Define the “TimeOfDay” class.
- Declare required variables.
- Define the constructor and instantiate the variables.
- Give function definition “setTimeTo ()” to set the time.
- Declare required variables.
- Create an object for the “Scanner” class.
- Split the string using a delimiter “:”.
- Inside the “try” block,
- Get the hour and store it in a variable.
- Catch the exception “Exception”.
- Throw the exception “InvalidFormattingException” with a message.
- Check if the hour value is valid.
- Throw “InvalidHourException” with a message.
- Get the remaining string and cut off the last two characters.
- Check if the string’s length is less than 3.
- Throw “InvalidFormattingException” with a message.
- Get the minutes and store it in a variable.
- Get “am” or “pm” and store it in a variable.
- Inside “try” block,
- Convert the minute string to integer.
- Catch the exception “Exception”.
- Throw “InvalidFormattingException” with a message.
- Check if minute is less than 0 or greater than 59.
- Throw “InvalidMinuteException” with a message.
- Check whether the string is not equal to “am” and “pm”.
- Throw “InvalidFormattingException” with a message.
- Check if the string is equal to “am”.
- Assign “true” to “isAM”.
- Else,
- Assign “false” to “isAM”.
- Function to produce the resultant string.
- Append all the value to a string.
- Define the “main ()” method.
- Create an object for the “Scanner” class.
- Create an object for the class “TimeOfDay”.
- Declare a variable “time”.
- Inside “try” block.
- Get the time from the user.
- Call the function “setTimeTo ()” by passing the time.
- Catch the exception “InvalidFormattingException”.
- Print the message using “getMessage ()” function.
- Catch the exception “InvalidHourException”.
- Print the message using “getMessage ()” function.
- Catch the exception “InvalidMinuteException”.
- Print the message using “getMessage ()” function.
- Print the time.
Creating “InvalidFormattingException.java”:
- Define a class “InvalidFormattingException” that extends “Exception”.
- Define a parameterized constructor.
- Call the parent class’s method using “super ()” by passing the message.
- Define a parameterized constructor.
Creating “InvalidHourException.java”:
- Define a class “InvalidHourException” that extends “InvalidFormattingException”.
- Define a parameterized constructor.
- Call the parent class’s method using “super ()” by passing the message.
- Define a parameterized constructor.
Creating “InvlaidMinuteException.java”:
- Define a class “InvalidMinuteException” that extends “InvalidFormattingException”.
- Define a parameterized constructor.
- Call the parent class’s method using “super ()” by passing the message.
- Define a parameterized constructor.
Program:
TimeOfDay.java:
//Import required packages
import java.util.*;
import java.util.Scanner;
//Define the main class
class TimeOfDay
{
//Declare required variables
private int hour, minute;
private boolean isAM;
//Constructor
public TimeOfDay()
{
//Instantiate the values
hour = 0;
minute = 0;
isAM = false;
}
//Function definition to set the time
public void setTimeTo(String aTime) throws InvalidFormattingException, InvalidHourException, InvalidMinuteException
{
//Declare required variables
int hourFound;
int minuteFound;
String indicatorFound;
//Create an object for the scanner class
Scanner reader = new Scanner(aTime);
//Split using the delimiter
reader.useDelimiter(":");
//Try block
try
{
//Assign the hour
hourFound = reader.nextInt();
}
//Catch the exception
catch (Exception e)
{
//Throw the exception with a message
throw new InvalidFormattingException("Hour not an integer");
}
//Check the condition
if(hourFound<1 || hourFound>12)
//Throw the exception with a message
throw new InvalidHourException("Hour not in the range of 1 to 12");
//Get the remaining string
String restOfString = reader.next();
reader = new Scanner(restOfString);
//Remove the last two characters
if(restOfString.length()<3)
//Throw the exception
throw new InvalidFormattingException("Bad format");
//Get the substring
String minuteString = restOfString.substring(0, restOfString.length()-2);
//Get the substring
String amString = restOfString.substring(restOfString.length()-2);
//Try block
try
{
//Convert the minute to integer
minuteFound = Integer.parseInt(minuteString);
}
//Catch the exception
catch (Exception e)
{
//Throw the exception
throw new InvalidFormattingException("Minute not an integer");
}
//Check the condition
if(minuteFound<0 || minuteFound>59)
//Throw the exception with a message
throw new InvalidMinuteException("Minute not in the range of 0 to 59");
//Check condition
if(!amString...

Want to see the full answer?
Check out a sample textbook solution
Chapter 9 Solutions
Java: An Introduction to Problem Solving and Programming (7th Edition)
- Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forward4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forward
- Of the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forwardManagement Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forward
- Please original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forwardPlease original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forward
- What are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forwardDiscuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forwardDetermine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
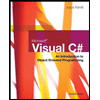
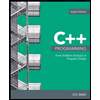