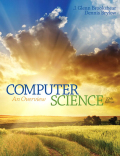
EBK COMPUTER SCIENCE: AN OVERVIEW
12th Edition
ISBN: 8220102744196
Author: BRYLOW
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 8, Problem 13CRP
Explanation of Solution
Function for reversing the order of a linked list:
//function for reversing the order of a linked list
//void Reverselist(Linkedlist list)
{
//creating pointers to the linked list
nodeType*current,*temp,*first;
//assign the head position to current node
current=head;
//make the first pointer to NULL
first=NULL;
//change the order of the list by using the while loop
while(current!=NULL)
{
first=temp;
current=first;
current=current->link;
}
//assign the head to the first pointer
first=head;
}
Explanation:
- Suppose *current, *first and *temp be any three points that point to the nodes in a list.
- The address of the head node is stored by the current pointer.
current=head;
- The NULL is initialized by the first pointer...
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
reminder it an exercice not a grading work
GETTING STARTED
Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website.
Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”.
If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically.
With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet.
If cell B6 does not display your name, delete the file and download a new copy from the SAM website.
Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…
Need help completing this algorithm here in coding! 2
Whats wrong the pseudocode here??
Chapter 8 Solutions
EBK COMPUTER SCIENCE: AN OVERVIEW
Ch. 8.1 - Give examples (outside of computer science) of...Ch. 8.1 - Prob. 2QECh. 8.1 - Prob. 3QECh. 8.1 - Prob. 4QECh. 8.1 - Prob. 5QECh. 8.2 - In what sense are data structures such as arrays,...Ch. 8.2 - Prob. 2QECh. 8.2 - Prob. 3QECh. 8.3 - Prob. 1QECh. 8.3 - Prob. 2QE
Ch. 8.3 - Prob. 3QECh. 8.3 - Prob. 4QECh. 8.3 - Modify the function in Figure 8.19 so that it...Ch. 8.3 - Prob. 7QECh. 8.3 - Prob. 8QECh. 8.3 - Draw a diagram representing how the tree below...Ch. 8.4 - Prob. 1QECh. 8.4 - Prob. 2QECh. 8.4 - Prob. 3QECh. 8.4 - Prob. 4QECh. 8.5 - Prob. 1QECh. 8.5 - Prob. 3QECh. 8.5 - Prob. 4QECh. 8.6 - In what ways are abstract data types and classes...Ch. 8.6 - What is the difference between a class and an...Ch. 8.6 - Prob. 3QECh. 8.7 - Suppose the Vole machine language (Appendix C) has...Ch. 8.7 - Prob. 2QECh. 8.7 - Using the extensions described at the end of this...Ch. 8.7 - In the chapter, we introduced a machine...Ch. 8 - Prob. 1CRPCh. 8 - Prob. 2CRPCh. 8 - (Asterisked problems are associated with optional...Ch. 8 - Prob. 4CRPCh. 8 - (Asterisked problems are associated with optional...Ch. 8 - Prob. 6CRPCh. 8 - Prob. 7CRPCh. 8 - Prob. 8CRPCh. 8 - Prob. 9CRPCh. 8 - Prob. 10CRPCh. 8 - Prob. 11CRPCh. 8 - Prob. 12CRPCh. 8 - Prob. 13CRPCh. 8 - Prob. 14CRPCh. 8 - Prob. 15CRPCh. 8 - Prob. 16CRPCh. 8 - Prob. 17CRPCh. 8 - Prob. 18CRPCh. 8 - Design a function to compare the contents of two...Ch. 8 - (Asterisked problems are associated with optional...Ch. 8 - (Asterisked problems are associated with optional...Ch. 8 - Prob. 22CRPCh. 8 - Prob. 23CRPCh. 8 - Prob. 24CRPCh. 8 - (Asterisked problems are associated with optional...Ch. 8 - Prob. 26CRPCh. 8 - Prob. 27CRPCh. 8 - Prob. 28CRPCh. 8 - Prob. 29CRPCh. 8 - Prob. 30CRPCh. 8 - Design a nonrecursive algorithm to replace the...Ch. 8 - Prob. 32CRPCh. 8 - Prob. 33CRPCh. 8 - Prob. 34CRPCh. 8 - Draw a diagram showing how the binary tree below...Ch. 8 - Prob. 36CRPCh. 8 - Prob. 37CRPCh. 8 - Prob. 38CRPCh. 8 - Prob. 39CRPCh. 8 - Prob. 40CRPCh. 8 - Modify the function in Figure 8.24 print the list...Ch. 8 - Prob. 42CRPCh. 8 - Prob. 43CRPCh. 8 - Prob. 44CRPCh. 8 - Prob. 45CRPCh. 8 - Prob. 46CRPCh. 8 - Using pseudocode similar to the Java class syntax...Ch. 8 - Prob. 48CRPCh. 8 - Identify the data structures and procedures that...Ch. 8 - Prob. 51CRPCh. 8 - In what way is a class more general than a...Ch. 8 - Prob. 53CRPCh. 8 - Prob. 54CRPCh. 8 - Prob. 55CRPCh. 8 - Prob. 1SICh. 8 - Prob. 2SICh. 8 - In many application programs, the size to which a...Ch. 8 - Prob. 4SICh. 8 - Prob. 5SICh. 8 - Prob. 6SICh. 8 - Prob. 7SICh. 8 - Prob. 8SI
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Help! how do I fix my python coding question for this? (my code also provided)arrow_forwardNeed help with coding in this in python!arrow_forwardIn the diagram, there is a green arrow pointing from Input C (complete data) to Transformer Encoder S_B, which I don’t understand. The teacher model is trained on full data, but S_B should instead receive missing data—this arrow should not point there. Please verify and recreate the diagram to fix this issue. Additionally, the newly created diagram should meet the same clarity standards as the second diagram (Proposed MSCATN). Finally provide the output image of the diagram in image format .arrow_forward
- Please provide me with the output image of both of them . below are the diagrams code make sure to update the code and mentionned clearly each section also the digram should be clearly describe like in the attached image. please do not provide the same answer like in other question . I repost this question because it does not satisfy the requirment I need in terms of clarifty the output of both code are not very well details I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder…arrow_forwardWhy I need ?arrow_forwardHere are two diagrams. Make them very explicit, similar to Example Diagram 3 (the Architecture of MSCTNN). graph LR subgraph Teacher_Model_B [Teacher Model (Pretrained)] Input_Teacher_B[Input C (Complete Data)] --> Teacher_Encoder_B[Transformer Encoder T] Teacher_Encoder_B --> Teacher_Prediction_B[Teacher Prediction y_T] Teacher_Encoder_B --> Teacher_Features_B[Internal Features F_T] end subgraph Student_B_Model [Student Model B (Handles Missing Labels)] Input_Student_B[Input C (Complete Data)] --> Student_B_Encoder[Transformer Encoder E_B] Student_B_Encoder --> Student_B_Prediction[Student B Prediction y_B] end subgraph Knowledge_Distillation_B [Knowledge Distillation (Student B)] Teacher_Prediction_B -- Logits Distillation Loss (L_logits_B) --> Total_Loss_B Teacher_Features_B -- Feature Alignment Loss (L_feature_B) --> Total_Loss_B Partial_Labels_B[Partial Labels y_p] -- Prediction Loss (L_pred_B) --> Total_Loss_B Total_Loss_B -- Backpropagation -->…arrow_forward
- Please provide me with the output image of both of them . below are the diagrams code I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder --> Student_A_Features[Student A Features F_A] end subgraph Knowledge_Distillation_A [Knowledge Distillation (Student A)] Teacher_Prediction -- Logits Distillation Loss (L_logits_A) --> Total_Loss_A Teacher_Features -- Feature Alignment Loss (L_feature_A) --> Total_Loss_A Ground_Truth_A[Ground Truth y_gt] -- Prediction Loss (L_pred_A)…arrow_forwardI'm reposting my question again please make sure to avoid any copy paste from the previous answer because those answer did not satisfy or responded to the need that's why I'm asking again The knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges…arrow_forwardThe knowledge distillation part is not very clear in the diagram. Please create two new diagrams by separating the two student models: First Diagram (Student A - Missing Values): Clearly illustrate the student training process. Show how knowledge distillation happens between the teacher and Student A. Explain what the teacher teaches Student A (e.g., handling missing values) and how this teaching occurs (e.g., through logits, features, or attention). Second Diagram (Student B - Missing Labels): Similarly, detail the training process for Student B. Clarify how knowledge distillation works between the teacher and Student B. Specify what the teacher teaches Student B (e.g., dealing with missing labels) and how the knowledge is transferred. Since these are two distinct challenges (missing values vs. missing labels), they should not be combined in the same diagram. Instead, create two separate diagrams for clarity. For reference, I will attach a second image…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
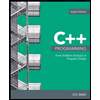
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
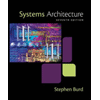
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
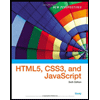
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
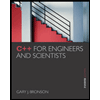
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
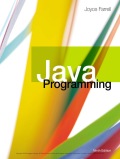
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage