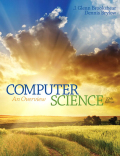
Concept explainers
Explanation of Solution
The function for concatenating two linked lists:
The function to concatenate two linked is dined as below.
//Define method concatenate
static void concatenate(node *a, node *b)
{
//If condition satisfies
if( a != NULL && b!= NULL )
{
//If condition satisfies
if (a->nxt == NULL)
//Assign value
a->nxt = b;
//If condition satisfies
else
//Call method
concatenate(a->nxt,b);
}
//If condition satisfies
else
{
//Display message
cout << "Either a or b is NULL\n";
}
}
Explanation:
- The function “concatenate()” concatenates values in two linked lists.
- It checks initially whether two linked lists are empty or not.
- If both linked lists are non-empty, it proceeds with checking the end of first linked list.
- Assign second linked list to the end of first linked list.
- The result is been displayed finally.
Complete Program:
//Include library
#include <iostream>
//Use namespace
using namespace std;
//Define structure
struct node
{
//Declare variable
int dta;
//Declare pointer
node *nxt;
};
//Define class
class lnkdLst
{
//Define access specifier
private:
//Define nodes
node *head,*tail;
//Define access specifier
public:
//Define constructor
lnkdLst()
{
//Assign value
head = NULL;
//Assign value
tail = NULL;
}
//Define method
void AdNode(int n)
{
//Assign value
node *tmp = new node;
//Assign value
tmp->dta = n;
//Assign value
tmp->nxt = NULL;
//If condition satisfies
if(head == NULL)
{
//Assign value
head = tmp;
//Assign value
tail = tmp;
}
//If condition satisfies
else
{
//Assign value
...
Trending nowThis is a popular solution!

Chapter 8 Solutions
EBK COMPUTER SCIENCE: AN OVERVIEW
- Please answer Java OOP Questions.arrow_forward.NET Interactive Solving Sudoku using Grover's Algorithm We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules: •No column may contain the same value twice •No row may contain the same value twice If we assign each square in our sudoku to a variable like so: 1 V V₁ V3 V2 we want our circuit to output a solution to this sudoku. Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the conversion of classical decision problems into oracles for Grover's algorithm. Turning the Problem into a Circuit We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit that…arrow_forwardNeed help with this in python!arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
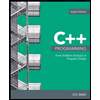
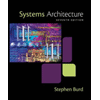
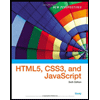
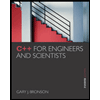
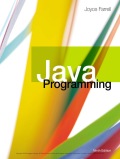