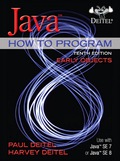
Java How To Program (Early Objects)
10th Edition
ISBN: 9780133807943
Author: Deitel, Paul
Publisher: Pearson Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 6, Problem 3MD
Program Plan Intro
Multiplication
Program Plan:
Multiply.java:
- Import required packages.
- Declare the class “Multiply”.
- Create an object for “random()”.
- Declare the required variables.
- Define the main method.
- Create an object for scanner class.
- Declare the variable “guessVal”.
- Call the method “Question()”.
- Get the guess number from the user.
- Check whether the “guessVal” is not equal to “-1”.
- Call the method “Response()”.
- Get the guess value from the user.
- Definition of method “Question()”.
- Get the two random numbers between 0 and 10.
- Multiply the two numbers.
- Print the two numbers.
- Definition of method “printResponse()”.
- Check the condition is “true”.
- For correct guess, give the corresponding case option.
- For incorrect guess, give the corresponding case option.
- Definition of method “Percentage()”.
- Calculate and return the result.
- Definition of method “Response()”.
- Increment the “iter++”.
- Check whether the “guess” is not equal to “crtAns”
- Call the method “printResponse()”.
- Check whether “iter” is less than “10”.
- Call the method “Question()”.
- Check whether “iter” is greater than “10”.
- Call the method “Percentage()”.
- Check whether the returned value from the method “Percentage()” is less than “0.75”.
- Assign the variables to “0”.
- Call the method “Question()”.
SampleTest.java:
- Definition of class “SampleTest”.
- Definition of main method.
- Create an object for “MultiplyVal” class.
- Call the method “quiz()”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Can I get help with this case please, thank you
I need help to solve the following, thank you
reminder it an exercice not a grading work
GETTING STARTED
Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website.
Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”.
If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically.
With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet.
If cell B6 does not display your name, delete the file and download a new copy from the SAM website.
Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…
Chapter 6 Solutions
Java How To Program (Early Objects)
Ch. 6 - A method is invoked with a(n)Ch. 6 - Prob. 1.2SRECh. 6 - Prob. 1.3SRECh. 6 - The keywordindicates that a method does not return...Ch. 6 - Prob. 1.5SRECh. 6 - Prob. 1.6SRECh. 6 - Prob. 1.7SRECh. 6 - An object of classproduces truly random numbers.Ch. 6 - Prob. 1.9SRECh. 6 - Prob. 1.10SRE
Ch. 6 - Prob. 1.11SRECh. 6 - Prob. 1.12SRECh. 6 - For the class Craps in Fig. 6.8, state the scope...Ch. 6 - the variable die1.Ch. 6 - the method rollDice.Ch. 6 - Prob. 2.4SRECh. 6 - Prob. 2.5SRECh. 6 - Write an application that tests whether the...Ch. 6 - Prob. 4.1SRECh. 6 - Method smallest, which takes three integers x, y...Ch. 6 - Prob. 4.3SRECh. 6 - Method intToFloat, which takes integer argument...Ch. 6 - Find the error in each of the following program...Ch. 6 - 1 int sum(int x, int y) { 2 int result; 3 result =...Ch. 6 - 1 void f(float a); { 2 float a; 3...Ch. 6 - 1 void product() { 2int, a = 6; 3int b = 5; 4int c...Ch. 6 - Declare method sphereVolume to calculate and...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - (Parking Charges) A parking garage charges a 2.00...Ch. 6 - (Rounding Numbers) Math.floor can be used to round...Ch. 6 - To round numbers to specific decimal places, use a...Ch. 6 - Prob. 5.1ECh. 6 - Write statements that assign random integers to...Ch. 6 - Write statements that will display a random number...Ch. 6 - (Exponentiation) Write a method integer Power...Ch. 6 - Define a method hypotenuse that calculates the...Ch. 6 - Prob. 10.1ECh. 6 - Prob. 11.1ECh. 6 - (Displaying a Square of Asterisks) Write a method...Ch. 6 - (Displaying a Square of Any Character) Modify the...Ch. 6 - Prob. 14.1ECh. 6 - (Separating Digits) Write methods that accomplish...Ch. 6 - (Separating Digits) Write methods that accomplish...Ch. 6 - Prob. 15.3ECh. 6 - (Temperature Conversions) Implement the following...Ch. 6 - Method fahrenheit returns the Fahrenheit...Ch. 6 - Prob. 16.3ECh. 6 - (Find the Minimum) Write a method minimum3 that...Ch. 6 - Prob. 18.1ECh. 6 - (Prime Numbers) A positive integer is prime if its...Ch. 6 - Prob. 20.1ECh. 6 - (Greatest Common Divisor) The greatest common...Ch. 6 - Write a method qualityPoints that inputs a...Ch. 6 - Write an application that simulates coin tossing....Ch. 6 - (Guess the Number) Write an application that plays...Ch. 6 - Prob. 25.1ECh. 6 - Prob. 26.1ECh. 6 - (Craps Game Modification) Modify the craps program...Ch. 6 - (Table of Binary, Octal and Hexadecimal Numbers)...Ch. 6 - (Computer-Assisted Instruction) The use of...Ch. 6 - Prob. 2MDCh. 6 - Prob. 3MDCh. 6 - (Computer-Assisted Instruction: Difficulty Levels)...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Need help with coding in this in python!arrow_forwardIn the diagram, there is a green arrow pointing from Input C (complete data) to Transformer Encoder S_B, which I don’t understand. The teacher model is trained on full data, but S_B should instead receive missing data—this arrow should not point there. Please verify and recreate the diagram to fix this issue. Additionally, the newly created diagram should meet the same clarity standards as the second diagram (Proposed MSCATN). Finally provide the output image of the diagram in image format .arrow_forwardPlease provide me with the output image of both of them . below are the diagrams code make sure to update the code and mentionned clearly each section also the digram should be clearly describe like in the attached image. please do not provide the same answer like in other question . I repost this question because it does not satisfy the requirment I need in terms of clarifty the output of both code are not very well details I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder…arrow_forward
- Why I need ?arrow_forwardHere are two diagrams. Make them very explicit, similar to Example Diagram 3 (the Architecture of MSCTNN). graph LR subgraph Teacher_Model_B [Teacher Model (Pretrained)] Input_Teacher_B[Input C (Complete Data)] --> Teacher_Encoder_B[Transformer Encoder T] Teacher_Encoder_B --> Teacher_Prediction_B[Teacher Prediction y_T] Teacher_Encoder_B --> Teacher_Features_B[Internal Features F_T] end subgraph Student_B_Model [Student Model B (Handles Missing Labels)] Input_Student_B[Input C (Complete Data)] --> Student_B_Encoder[Transformer Encoder E_B] Student_B_Encoder --> Student_B_Prediction[Student B Prediction y_B] end subgraph Knowledge_Distillation_B [Knowledge Distillation (Student B)] Teacher_Prediction_B -- Logits Distillation Loss (L_logits_B) --> Total_Loss_B Teacher_Features_B -- Feature Alignment Loss (L_feature_B) --> Total_Loss_B Partial_Labels_B[Partial Labels y_p] -- Prediction Loss (L_pred_B) --> Total_Loss_B Total_Loss_B -- Backpropagation -->…arrow_forwardPlease provide me with the output image of both of them . below are the diagrams code I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder --> Student_A_Features[Student A Features F_A] end subgraph Knowledge_Distillation_A [Knowledge Distillation (Student A)] Teacher_Prediction -- Logits Distillation Loss (L_logits_A) --> Total_Loss_A Teacher_Features -- Feature Alignment Loss (L_feature_A) --> Total_Loss_A Ground_Truth_A[Ground Truth y_gt] -- Prediction Loss (L_pred_A)…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
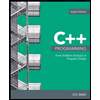
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
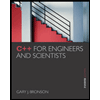
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
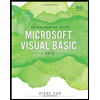
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
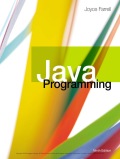
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
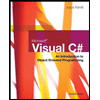
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Literals in Java Programming; Author: Sudhakar Atchala;https://www.youtube.com/watch?v=PuEU4S4B7JQ;License: Standard YouTube License, CC-BY
Type of literals in Python | Python Tutorial -6; Author: Lovejot Bhardwaj;https://www.youtube.com/watch?v=bwer3E9hj8Q;License: Standard Youtube License