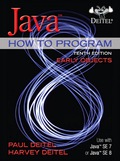
Java How To Program (Early Objects)
10th Edition
ISBN: 9780133807943
Author: Deitel, Paul
Publisher: Pearson Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 6, Problem 14.1E
Program Plan Intro
Circle Area
Program Plan:
- Import required packages.
- Declare the class “Circle”.
- Define the main method.
- Create an object “in” for the scanner class.
- Get the radius from the user.
- Validate the radius using “while” loop.
- Call the method “circleArea()”.
- Get the radius from the user.
- Definition of method “circleArea()”..
- Calculate and print the area of the circle.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
(Calculating the Product of Odd Integers) Write an application that calculates the product of the odd integers from 1 to 15.
(In Java) In the game Clacker, the numbers 1 through 12 are initially displayed. The player throws two dice and may cover the number representing the total or the two numbers on the dice. For example, for a throw of 3 and 5, the player may cover the 3 and the 5 or just the 8. Play continues until all the numbers are covered. The goal is to cover all numbers in the fewest rolls.
Create a Clacker application that displays 12 buttons numbered 1 through 12. Each of these buttons can be clicked to ‘cover’ it. A covered button displays nothing. Another button labelled Roll can be clicked to roll the dice. Include labels to display the appropriate die images for each roll. Another label displays the number of rolls taken. A New Game button can be clicked to clear the labels and uncover the buttons for a new game.
(PLEASE USE JAVA AND JFRAME GUI)
Game rules:The game consists of a two-dimensional field of size m × n (game field). Each element ofthe game field is a button with a number assigned to it.Initialization of the game:• The numbers on the buttons of the game field are set to a random digit between 0and 9• The target value is displayed above the game field• The current sum of the numbers displayed on the buttons of the game field is printedbelow the game field• The number of moves to completion is displayed in the upper right corner above thegame field.Playing:The first move is determined by the player by selecting any button (button A) on thegame field.1. The player is allowed to choose a second button (button B) located in the columnor row of the previously selected button (A).2. Upon selecting the second button (B), the value of button (A) is updated accordingto the following formula: A = (AoperationB)mod10.3. The operation for the basic game will be +.4. Decrement the number of moves…
Chapter 6 Solutions
Java How To Program (Early Objects)
Ch. 6 - A method is invoked with a(n)Ch. 6 - Prob. 1.2SRECh. 6 - Prob. 1.3SRECh. 6 - The keywordindicates that a method does not return...Ch. 6 - Prob. 1.5SRECh. 6 - Prob. 1.6SRECh. 6 - Prob. 1.7SRECh. 6 - An object of classproduces truly random numbers.Ch. 6 - Prob. 1.9SRECh. 6 - Prob. 1.10SRE
Ch. 6 - Prob. 1.11SRECh. 6 - Prob. 1.12SRECh. 6 - For the class Craps in Fig. 6.8, state the scope...Ch. 6 - the variable die1.Ch. 6 - the method rollDice.Ch. 6 - Prob. 2.4SRECh. 6 - Prob. 2.5SRECh. 6 - Write an application that tests whether the...Ch. 6 - Prob. 4.1SRECh. 6 - Method smallest, which takes three integers x, y...Ch. 6 - Prob. 4.3SRECh. 6 - Method intToFloat, which takes integer argument...Ch. 6 - Find the error in each of the following program...Ch. 6 - 1 int sum(int x, int y) { 2 int result; 3 result =...Ch. 6 - 1 void f(float a); { 2 float a; 3...Ch. 6 - 1 void product() { 2int, a = 6; 3int b = 5; 4int c...Ch. 6 - Declare method sphereVolume to calculate and...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - What is the value of x after each of the following...Ch. 6 - (Parking Charges) A parking garage charges a 2.00...Ch. 6 - (Rounding Numbers) Math.floor can be used to round...Ch. 6 - To round numbers to specific decimal places, use a...Ch. 6 - Prob. 5.1ECh. 6 - Write statements that assign random integers to...Ch. 6 - Write statements that will display a random number...Ch. 6 - (Exponentiation) Write a method integer Power...Ch. 6 - Define a method hypotenuse that calculates the...Ch. 6 - Prob. 10.1ECh. 6 - Prob. 11.1ECh. 6 - (Displaying a Square of Asterisks) Write a method...Ch. 6 - (Displaying a Square of Any Character) Modify the...Ch. 6 - Prob. 14.1ECh. 6 - (Separating Digits) Write methods that accomplish...Ch. 6 - (Separating Digits) Write methods that accomplish...Ch. 6 - Prob. 15.3ECh. 6 - (Temperature Conversions) Implement the following...Ch. 6 - Method fahrenheit returns the Fahrenheit...Ch. 6 - Prob. 16.3ECh. 6 - (Find the Minimum) Write a method minimum3 that...Ch. 6 - Prob. 18.1ECh. 6 - (Prime Numbers) A positive integer is prime if its...Ch. 6 - Prob. 20.1ECh. 6 - (Greatest Common Divisor) The greatest common...Ch. 6 - Write a method qualityPoints that inputs a...Ch. 6 - Write an application that simulates coin tossing....Ch. 6 - (Guess the Number) Write an application that plays...Ch. 6 - Prob. 25.1ECh. 6 - Prob. 26.1ECh. 6 - (Craps Game Modification) Modify the craps program...Ch. 6 - (Table of Binary, Octal and Hexadecimal Numbers)...Ch. 6 - (Computer-Assisted Instruction) The use of...Ch. 6 - Prob. 2MDCh. 6 - Prob. 3MDCh. 6 - (Computer-Assisted Instruction: Difficulty Levels)...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- JAVA:arrow_forward# given a radius, find the area of a circle # use value of pi as 3.1415 def get_area_circle(r): """ what it takes radius of a circle (any positive real number) what it does: computes the area of a circle given a radius, find the area of a circle area = pi*r2 (pi times r squared) what it returns area of a circle. Any positive real number (float) """ # your code goes in herearrow_forwardThank you so much for your help!arrow_forward
- (In Java) The SalesCenter application stores information about three employees. There is one manager (Diego Martin, salary $55,000), and two associates (Kylie Walter earning $18.50 per hour and Michael Rose earning $16.75 per hour). SalesCenter should be able to display the name and title for a specified employee. Additionally, the SalesCenter application should calculate and display the pay for a specified employee based on the pay argument entered by the user. The pay argument should correlate to hours worked if the pay for an associate is to be calculated. The pay argument for a manager should correlate to the number of weeks the manager is to be paid for. The SalesCenter interface should provide a menu of options. Depending on the option selected, additional input may be needed. Create client code that will generate the following SalesCenter output sketch: Employee/Pay/Quit Enter choice: E Enter employee number(1, 2, or 3): 2 Kylie Walter, associate Employee\Pay\Quit Enter…arrow_forwardPlease add commentsarrow_forward(Check password) Some websites impose certain rules for passwords. Write a method that checks whether a string is a valid password. Suppose the password rules are as follows: A password must have at least eight characters. A password consists of only letters and digits. A password must contain at least two digits. Write a program that prompts the user to enter a password and displays Valid Password if the rules are followed or Invalid Password otherwise.arrow_forward
- (Check the speed) Write a program that prompts the user to enter the speed of a vehicle. If speed is less than 20, display too slow; if speed is greater than 80, display too fast; otherwise, display just right.arrow_forward• FormulaEvaluator.java Requirements: Evaluate the following expression for any value of the variable x, and save the result in a variable of the type double. You must use the abs(), pow(), and sqrt() methods of the Math class to perform the calculation. You may use a single assignment statement with a somewhat large expression, or you may break the expression into multiple assignment statements. The latter may be easier to debug if you are not getting the correct result. 5x +3} ( √8x* — 6x²³ + 4x² + |20x+1) (9x³+7x² +5x+3) Next, determine the number of digits to the left and to the right of the decimal point in the result. [Hint: You can convert the double variable into a String variable using the static method Double.toString(result). Then, on this String variable use the indexOf() method from the String class to find the position of the decimal point (".") and use the length() method to find the length. Knowing the location of the decimal point and the length of the String, you…arrow_forwardThis needs to be done in Java! (Calculating Sales) An online retailer sells five products whose retail prices are as follows: Product 1, $2.98; product 2, $4.50; product 3, $9.98; product 4, $4.49 and product 5, $6.87. Write an application that reads a series of pairs of numbers as follows: product number quantity sold Your program should use a switch statement to determine the retail price for each product. It should calculate and display the total retail value of all products sold. Use a sentinel-controlled loop to determine when the program should stop looping and display the final results.arrow_forward
- Guessing game: Read a number until it's equal to a chosen number (first it can be a hard-coded constant or #define, or you can check rand() later). In case of wrong guessing help the user by printing whether the guessed number is to small or too big. ( in C language)arrow_forwardFinancial Application:• Write a program that computes future investment value at a given interest rate for aspecified number of months and prints the report shown in the sample output. • Given the annual interest rate, the interest amount earned each month is computedusing the formula: Interest earned = investment amount * annual interest rate /1200 (=months * 100) • Write a method, computeFutureValue, which receives the investment amount, annualinterest rate and number of months as parameters and does the following: o Prints the interest amount earned each month and the new value of theinvestment (hint: use a loop). o Returns the total interest amount earned after the number of months specified bythe user. The main method will:o Ask the user for all input needed to call the computeFutureValue method.o Call computeFutureValueo Print the total interest amount earned by the investment at the end of thenumber of months entered by the user. Sample Program runningEnter the investment…arrow_forwardFinancial Application:• Write a program that computes future investment value at a given interest rate for aspecified number of months and prints the report shown in the sample output. • Given the annual interest rate, the interest amount earned each month is computedusing the formula: Interest earned = investment amount * annual interest rate /1200 (=months * 100) • Write a method, computeFutureValue, which receives the investment amount, annualinterest rate and number of months as parameters and does the following: o Prints the interest amount earned each month and the new value of theinvestment (hint: use a loop). o Returns the total interest amount earned after the number of months specified bythe user. The main method will:o Ask the user for all input needed to call the computeFutureValue method.o Call computeFutureValueo Print the total interest amount earned by the investment at the end of thenumber of months entered by the user. Sample Program runningEnter the investment…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
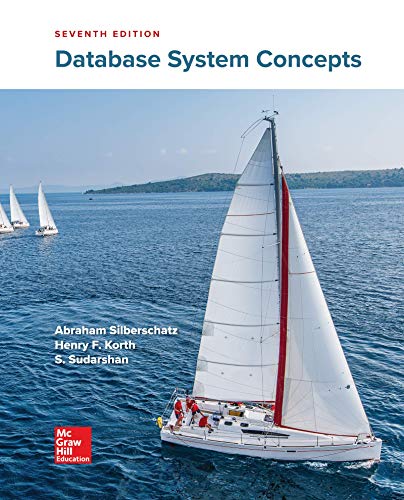
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
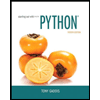
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
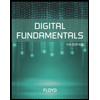
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
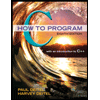
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
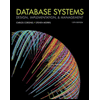
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
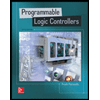
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education