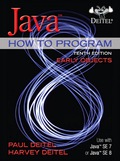
Write an application that simulates coin tossing. Let the

Want to see the full answer?
Check out a sample textbook solution
Chapter 6 Solutions
Java How To Program (Early Objects)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Experiencing MIS
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Starting Out with C++ from Control Structures to Objects (8th Edition)
Differential Equations: Computing and Modeling (5th Edition), Edwards, Penney & Calvis
- The Yukon Widget Company manufactures widgets that weigh 9.2 pounds each. Write a program that calculates how many widgets are stacked on a pallet, based on the total weight of the pallet. The program should ask the user how much the pallet weighs by itself and with the widgets stacked on it. It should then calculate and display the number of widgets stacked on the pallet.arrow_forwardScreen shot pleasearrow_forwardMethods with an empty parameter list and do not return a value: [Questions 1-8 required] You invoke a method by its name followed by a pair of brackets and the usual semi-colon Write a method called DisplayPersonalInfo(). This method will display your name, school, program and your favorite course. Call the DisplayPersonalInfo() method from your program Main() method Write a method called CalculateTuition(). This method will prompt the user for the number of courses that she is currently taking and then calculate and display the tuition cost. (cost = number of course * 569.99). Call the CalculateTuition() method two times from the same Main() method as in question 1. Write a method call CalculateAreaOfCircle(). This method will prompt the user for the radius of a circle and then calculate and display the area.[A = πr2].Call the CalculateAreaOfCircle() method twice from the same Main() method as in question 1. Use Math.Pi for the value of π Write a method call…arrow_forward
- Create a method that takes a value and indicate whether it is positive or negative by a return aBoolean value.Declared as: boolean isPositive (float a)arrow_forwardTrue or False? Arrays cannot be passed as parameters, but must be used only in the main method.arrow_forwardLook at the header of the method below, and then implement a sample call to it in your code.private static void ShowValue ()arrow_forward
- A video player plays a game in which the character competes in a hurdle race. Hurdles are of varying heights, and the characters have a maximum height they can jump. There is a magic potion they can take that will increase their maximum jump height by unit for each dose. How many doses of the potion must the character take to be able to jump all of the hurdles. If the character can already clear all of the hurdles, return . Example The character can jump unit high initially and must take doses of potion to be able to jump all of the hurdles. Function Description Complete the hurdleRace function in the editor below. hurdleRace has the following parameter(s): int k: the height the character can jump naturally int height[n]: the heights of each hurdle Returns int: the minimum number of doses required, always or more Input Format The first line contains two space-separated integers and , the number of hurdles and the maximum height the character can jump naturally.The…arrow_forward// Question 4: // Declare an integer variable named "variables with an initial value of 10. // Write a method named "SetToOne" that takes one out parameter. // The method should set the out parameter to 1. // Call the method SetToOne passing variables in the btngs click method. // Display the variable variables in the lblQ4. private void btn04_Click(object sender, EventArgs e) {arrow_forwardIn a C# Console App do the following Write a method that takes three integers and returns true if their sum is divisible by 3, false otherwise. Write a method that takes two strings and displays the string that has fewer characters. Write a method that takes two bool variables and returns true if they have the same value, false otherwise. Write a method that takes an int and a double and returns their product.arrow_forward
- Write a game of rock paper scissors, one of the choices will be from the user and the other from the computer (use random) Print results for each game.Play the games in a row until you exit the game. Explain each step as comment.Game rules:Rock crushes scissors, you win.Paper covers rock, you win.Scissors cut paper, you winarrow_forward// Question 4: // Declare an integer variable named "variables with an initial value of 10. // Write a method named "SetToOne" that takes one out parameter. // The method should set the out parameter to 1. // Call the method SetToOne passing variables in the btnQ4 click method. // Display the variable variables in the lblQ4. private void btn04_Click(object sender, EventArgs e) {arrow_forwardWrite a program that generates a random number in the range of 1 through 1000, and asks the user to guess what the number is. If the user guess is higher than the random number, the program should display Too high, try again. If the users guess is lower than the random number, the program should display Too low, try again. If the user guesses the number, the application should congratulate the user and generate a new random number so the game can start over. If the user doesn't want to continue the game the user should type an appropriate stop symbol to terminate the program (such stop conditions were discussed in the class). For each game iteration, count the number of guesses made by the user and display them. The program should contain the following functions: check_guess(): this function checks if the user's guess is lower, higher or equal to the random number. random_gen(): function that generates one random number for one game iteration main(): the function where the program…arrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
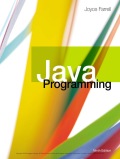
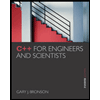
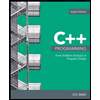