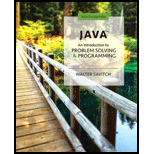
Concept explainers
Assume that nextWord is a string variable that has been given a value consisting entirely of letters. Write some Java code that displays the message First half of the alphabet, provided that nextWord precedes the letter N in alphabetic ordering. If nextword does not precede N in alphabetic ordering, your code should display Second half of the alphabet. Be careful when you represent the letter N. You will need a string value, as opposed to a
char value.

Want to see the full answer?
Check out a sample textbook solution
Chapter 3 Solutions
Java: An Introduction to Problem Solving and Programming (8th Edition)
Additional Engineering Textbook Solutions
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Mechanics of Materials (10th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
SURVEY OF OPERATING SYSTEMS
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
- Draw a context diagram for this scenario: You are developing a new customer relationship Management system for the BEC store, which rents out movies to customers. Customers will provide comments on new products, and request rental extensions and new products, each of which will be stored into the system and used by the manager for purchasing movies, extra copies, etc. Each month, one employee of BEC will select their favorite movie pick of that week, which will be stored in. the system. The actual inventory information will be stored in the Entertainment Tracker system, and would be retrieved by this new system as and when necessary.arrow_forwardWrite a complete Java program named FindSumAndAverage that performs the following tasks in 2-D array: Main Method: a. The main() method asks the user to provide the dimension n for a square matrix. A square matrix has an equal number of rows and columns. b. The main() method receives the value of n and calls the matrixSetUp() method that creates a square matrix of size n and populates it randomly with integers between 1 and 9. c. The main method then calls another method named printMatrix() to display the matrix in a matrix format. d. The main method also calls a method named findSumAndAverage() which: • Receives the generated matrix as input. • Calculates the sum of all elements in the matrix. • Calculates the average value of the elements in the matrix. • Stores these values (sum and average) in a single-dimensional array and returns this array • e. The main method prints the sum and average based on the result returned from findSumAndAverage()). Enter the dimension n for the square…arrow_forwardThe partial sums remain the same no matter what indexing we done to s artial sum of each series onverges, * + s of each series to the series or show 12. (1)+(0)+(0)+(+1)+ 17, " (F) + (F) + (F)(F)(- 18. 19. 1 #20. (三)+(三)-(三)+(3) 20 (9)-(0)-(0)-- 10 +1 2.1+(男)+(男)+(罰)+(鄂 9 T29 x222-끝+1-23 + -.... Repeating Decimals 64 Express each of the numbers in Exercises 23-30 as the m integers. 23. 0.23 = 0.23 23 23... 24. 0.234 = 0.234 234 234. 25. 0.7 = 0.7777... 26. 0.d = 0.dddd... where d is a digit natio of own s converges or * 27. 0.06 = 0.06666.. 28. 1.4141.414 414 414... 29. 1.24123 = 1.24 123 123 123... 30. 3.142857 = 3.142857 142857. Using the ath-Term Test In Exercises 31-38, use the ath-Term Test for divergence to show that the series is divergent, or state that the test is inconclusive 8arrow_forward
- CPS 2231 Computer Programming Homework #3 Due Date: Posted on Canvas 1. Provide answers to the following Check Point Questions from our textbook (5 points): a. How do you define a class? How do you define a class in Eclipse? b. How do you declare an object's reference variable (Hint: object's reference variable is the name of that object)? c. How do you create an object? d. What are the differences between constructors and regular methods? e. Explain why we need classes and objects in Java programming. 2. Write the Account class. The UML diagram of the class is represented below (10 points): Account id: int = 0 - balance: double = 0 - annualInterestRate: double = 0.02 - dateCreated: java.util.Date + Account() + Account(id: int, balance: double) + getId(): int + setId(newId: int): void + getBalance(): double + setBalance(newBalance: double): void + getAnnualInterestRate(): double + setAnnualInterest Rate (newRate: double): void + toString(): String + getDataCreated(): java.util.Date +…arrow_forwardTHIS IS NOT A GRADING ASSIGNMENT: Please only do lab 2.2 (bottom part of the first picture) For that Lab 2.2 do: *Part 1 (do the CODE, that's super important I need it) *Part 2 *Part 3 I also attached Section 2.5.2 which is part of the step 1 so you can read what is it about. Thank you!arrow_forwardTHIS IS NOT A GRADING ASSIGNMENT: Please only do lab 2.2 (bottom part of the first picture) For that Lab 2.2 do: *Part 1 *Part 2 *Part 3 I also attached Section 2.5.2 which is part of the step 1 so you can read what is it about. Thank you!arrow_forward
- can you please give me: * the code (step 3) *the list file (step 5) *and answer step 6 Thank youarrow_forward# Find the error# Why will the following code not print out a list of contact namesphoneBook = {'Doe, Jane' : '843-000-0000' ,'Doe, John' : '843-111-1111' ,'Smith, Adam' : '843-222-2222' ,'Jobs, Steve' : '999-333-3333' ,}for contact in phoneBook.values():print(contact)arrow_forward# Find the error:# The following code creates an empty dictionary and attempts to add a record# Why will the following code not create a new dictionary entry as intended?phoneBook = {}phoneBook{'Jobs, Steve'} = '999-111-1111'arrow_forward
- Select all the possible polar representations of the vector that is obtained from rotating where by Zrot Ź x = 3e² T= 3п 8 Hint: Consider the negative angle that is equivalent to the positive angle of the rotated vector. 0arrow_forwardCharacter Analysis If you have downloaded the source code you will find a file named text.txt on the Chapter 08 folder. Write a program that reads the file's contents and determines the following: The number of uppercase letters in the file The number of lowercase letter in the file The number of digits in the file The number of whitespace characters in the filearrow_forwardWrite a program that reads the text file's contents and calculates and outputs the following in this order: • The number of words in the file • The number of lines in the file • The number of uppercase letters in the file • The number of lowercase letters in the file • The number of digits in the file • The number of letter H's in the file • The number of whitespace characters in the file NOTE: Your program should include at least one try-except error handling statement block. Your program should also validate any input that could cause your program to crash. I'm Henery The Eighth, I Am! Henery The Eighth, I Am, I am!I got married to the widow next door,She's been married seven times before.And ev'ryone was a Henery,She wouldn't have Willie or a Sam.I'm her eighth old man named Henery,Henery the Eighth, I Am!Second verse same as the first!I'm Henery The Eighth, I Am! Henery The Eighth, I Am, I am!I got married to the widow next door,She's been married seven times before.And…arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
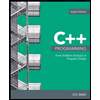
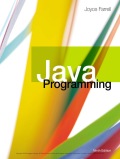
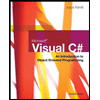
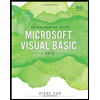
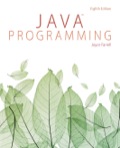
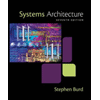