Laboratory 2.1 - Multi-bytes Integer Addition using Subroutines Objective: The objective of this laboratory is to get students familiar with the way to extend the limited arithmetic capabilities of the MC8051 using software and structured coding by the utilization of appropriate subroutines. The purpose of this lab is to program the MC8051 to add multi bytes two signed integers. To simplify our laboratory, we will assume three bytes long (24 bits) signed integers. Each of the three bytes will be defined in three consecutive code memory bytes using the "DB" assembler directive and the little endian format. The program will store the answer in three consecutive internal data memory bytes starting at Ram address 40H. The program will also display the answer on PO, P1, and P2 staring with the LS Byte in P0. Port 3 pin 0 (P3.0) will be set if an overflow takes place. otherwise it will stay cleared. The implementation will employ two subroutines to make advantage of the repeated code blocks. Steps: 1. First, configure your code and data memory. The "MAIN:" program starts at code memory location 30H. The first integer uses code memory locations 100H, 101H, and 102H in the Intel MC8051 little endian format (least significant byte stored at the low address). The second integer uses code memory locations 105H, 106H, and 107H. 2. Second, configure parallel port 0, port 1, port 2, and P3.0 for output (write 0 in the port once during initialization). Write and debug the code to add the two integers and store the answer in internal data memory locations 40H, 41H, and 42H. Update P0, P1, and P2 to display the same addition result. Flag an overflow condition on P3.1 by setting it. 3. Third, rewrite the program utilizing two subroutines to make use of the repeated code blocks. Define the created two subroutines sequentially after the main program before the program "END" directive. 4. Check the program for different possible integer's cases; two positives, two negatives, negative and positive. Verify the overall operations. Make sure to test cases producing an overflow. Also make use of break points in the Keil debugger to enhance and speed up the checkout. 5. Document and submit your final report along with the well documented list file and adequate debugging screen shots taken during program execution. Also describe the steps and tools used in debugging your program. 6. Explain how the use of subroutines affect the execution time and the space (memory) requirements of your executable code.
Laboratory 2.1 - Multi-bytes Integer Addition using Subroutines Objective: The objective of this laboratory is to get students familiar with the way to extend the limited arithmetic capabilities of the MC8051 using software and structured coding by the utilization of appropriate subroutines. The purpose of this lab is to program the MC8051 to add multi bytes two signed integers. To simplify our laboratory, we will assume three bytes long (24 bits) signed integers. Each of the three bytes will be defined in three consecutive code memory bytes using the "DB" assembler directive and the little endian format. The program will store the answer in three consecutive internal data memory bytes starting at Ram address 40H. The program will also display the answer on PO, P1, and P2 staring with the LS Byte in P0. Port 3 pin 0 (P3.0) will be set if an overflow takes place. otherwise it will stay cleared. The implementation will employ two subroutines to make advantage of the repeated code blocks. Steps: 1. First, configure your code and data memory. The "MAIN:" program starts at code memory location 30H. The first integer uses code memory locations 100H, 101H, and 102H in the Intel MC8051 little endian format (least significant byte stored at the low address). The second integer uses code memory locations 105H, 106H, and 107H. 2. Second, configure parallel port 0, port 1, port 2, and P3.0 for output (write 0 in the port once during initialization). Write and debug the code to add the two integers and store the answer in internal data memory locations 40H, 41H, and 42H. Update P0, P1, and P2 to display the same addition result. Flag an overflow condition on P3.1 by setting it. 3. Third, rewrite the program utilizing two subroutines to make use of the repeated code blocks. Define the created two subroutines sequentially after the main program before the program "END" directive. 4. Check the program for different possible integer's cases; two positives, two negatives, negative and positive. Verify the overall operations. Make sure to test cases producing an overflow. Also make use of break points in the Keil debugger to enhance and speed up the checkout. 5. Document and submit your final report along with the well documented list file and adequate debugging screen shots taken during program execution. Also describe the steps and tools used in debugging your program. 6. Explain how the use of subroutines affect the execution time and the space (memory) requirements of your executable code.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter12: Points, Classes, Virtual Functions And Abstract Classes
Section: Chapter Questions
Problem 19SA
Related questions
Question
100%
can you please give me:
* the code (step 3)
*the list file (step 5)
*and answer step 6
Thank you

Transcribed Image Text:Laboratory 2.1 - Multi-bytes Integer Addition using Subroutines
Objective: The objective of this laboratory is to get students familiar with the way to extend the
limited arithmetic capabilities of the MC8051 using software and structured coding by the
utilization of appropriate subroutines.
The purpose of this lab is to program the MC8051 to add multi bytes two signed integers. To
simplify our laboratory, we will assume three bytes long (24 bits) signed integers. Each of the three
bytes will be defined in three consecutive code memory bytes using the "DB" assembler directive
and the little endian format. The program will store the answer in three consecutive internal data
memory bytes starting at Ram address 40H. The program will also display the answer on PO, P1,
and P2 staring with the LS Byte in P0. Port 3 pin 0 (P3.0) will be set if an overflow takes place.
otherwise it will stay cleared. The implementation will employ two subroutines to make advantage
of the repeated code blocks.
Steps:
1. First, configure your code and data memory. The "MAIN:" program starts at code memory
location 30H. The first integer uses code memory locations 100H, 101H, and 102H in the Intel
MC8051 little endian format (least significant byte stored at the low address). The second integer
uses code memory locations 105H, 106H, and 107H.
2. Second, configure parallel port 0, port 1, port 2, and P3.0 for output (write 0 in the port once
during initialization). Write and debug the code to add the two integers and store the answer in
internal data memory locations 40H, 41H, and 42H. Update P0, P1, and P2 to display the same
addition result. Flag an overflow condition on P3.1 by setting it.
3. Third, rewrite the program utilizing two subroutines to make use of the repeated code blocks.
Define the created two subroutines sequentially after the main program before the program "END"
directive.
4. Check the program for different possible integer's cases; two positives, two negatives, negative
and positive. Verify the overall operations. Make sure to test cases producing an overflow. Also
make use of break points in the Keil debugger to enhance and speed up the checkout.
5. Document and submit your final report along with the well documented list file and adequate
debugging screen shots taken during program execution. Also describe the steps and tools used in
debugging your program.
6. Explain how the use of subroutines affect the execution time and the space (memory)
requirements of your executable code.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
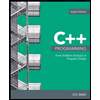
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
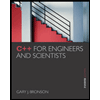
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
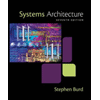
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
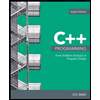
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
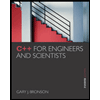
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
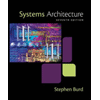
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
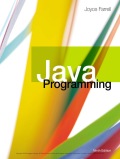
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
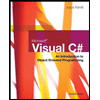
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
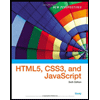
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:
9781305503922
Author:
Patrick M. Carey
Publisher:
Cengage Learning