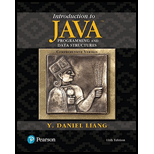
(Display words in ascending alphabetical order) Write a

Display words in ascending alphabetical order
Program plan:
- Import the required packages into the program.
- In the main() method,
- Check whether the argument length is not equal to 1. If yes,
- Display the error message.
- Assign the argument 0 as filename.
- Create a list to hold the words.
- In try block,
- Check the matches between the word from file and alphabets.
- Call the add() method to add the word into list.
- Catch the exception if error occurs.
- Call sort() method to sort the list in ascending order.
- Display the ascending order word.
- Check whether the argument length is not equal to 1. If yes,
The below program demonstrates to sort the word in ascending order from the file and display that list.
Explanation of Solution
Program:
//Import the java packages
import java.util.*;
import java.io.*;
//Class definition
public class Exercise20_01
{
//Main method
public static void main(String[] args)
{
/*Check whether the argument length is not equal to 1 */
if (args.length != 1) {
//Display the error message
System.out.println("Usage: java Exercise20_01 fullfilename");
System.exit(1);
}
//Assign the argument 0 as filename
String filename = args[0];
// Create a list to hold the words
List<String> list = new ArrayList<>();
//In try block
try {
//Create an object for Scanner class
Scanner in = new Scanner(new File(filename));
//Loop executes until the input from file
while (in.hasNext()) {
//Declare and assign the string //variable
String word = in.next();
//Check matches among the word from //file
if (word.matches("[a-z|A-Z].*"))
/*Call add() method to add the word into list */
list.add(word);
}
}
//Catch the exception
catch (Exception ex) {
//Display the error message
System.err.println(ex);
}
/*Call sort() method to sort the list in ascending order */
Collections.sort(list);
// Display the result
System.out.println("Display words in ascending order ");
//Loop executes until the list
for (String word : list) {
//Display the ascending order word
System.out.println(word);
}
}
}
Input file:
Screenshot of Test.txt file
Command to run the program:
java Exercise20_01 Test.txt
Display words in ascending order
hello
hi
hi
jhon
mercy
welcome
Want to see more full solutions like this?
Chapter 20 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Introduction To Programming Using Visual Basic (11th Edition)
Mechanics of Materials (10th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Degarmo's Materials And Processes In Manufacturing
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
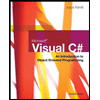
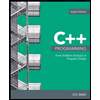
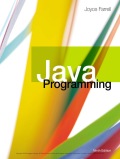
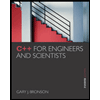
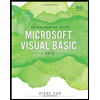