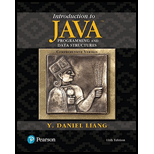
Concept explainers
Explanation of Solution
UnsupportedOperationException:
“UnsupportedOpertationException” is to indicate that the operation indicated in the class or subclass is not supported.
It is a runtime exception and the exception thrown being thrown by Java Virtual Machine(JVM).
Sample Program:
import java.util.Collections;
import java.util.HashSet;
import java.util.Random;
import java.util.Set;
public class UnsupportedOperationExceptionExample
{
// creating variable
private final static int Elements = 10;
// creating instance for the Random class
private final static Random random = new Random();
//Main method
public static void main(String[] args)
{
// creating set
Set integers = new HashSet(Elements);
// adding elements to the set

Want to see the full answer?
Check out a sample textbook solution
Chapter 20 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
- Java Programming: Write a static method named urgentAndIncomplete. Write the method as if it is in Main.java (not part of the ToDoItem class). The method should: Accept three ToDoItem references as parameters, and Return an int. Count how many of the ToDoItems meet two criteria: A priority greater than 3 Not completed The method should return how many of the parameters meet these criteria. That will be either 0, 1, 2, or 3.arrow_forward//Todo write test cases for SimpleCalculator Class // No need to implement the actual Calculator class just write Test cases as per TDD. // you need to just write test cases no mocking // test should cover all methods from calculator and all scenarios, so a minimum of 5 test // 1 for add, 1 for subtract, 1 for multiply, 2 for divide (1 for normal division, 1 for division by 0) // make sure all these test cases fail public class CalculatorTest { //Declare variable here private Calculator calculator; //Add before each here //write test cases here }arrow_forwardIN JAVA When is is necessary to create an object to call a method? A. When the method is an instance method. B. When the method has a return value. C. When the method is void. D. When the method is a static method.arrow_forward
- private int b; public int method3() { return b; } // in another class an object of the first class was introduced and inside the second class : float y = tester.method3(); are there any errors ? and explain pleasearrow_forwardFinish the TestPlane class that contains a main method that instantiates at least two Planes. Add instructions to instantiate your favorite plane and invoke each of the methods with a variety of parameter values to test each option within each method. To be able to test the functionality of each phase, you will add instructions to the main method in each phase.arrow_forwardhttps://youtu.be/4hAoK54Pfdc Here is a video regarding the kit component.arrow_forward
- Overriding is useful when a method has the same signature and type as a method in the parent class. O a local variable has the same name as an instance variable. O you want to change the return type of a method. O a method has the same signature as another method in the same class.arrow_forwardMethod overloading is a word that refers to the practise of using an excessive number of methods.arrow_forward1. Modify the method beginsWithVowel. Use a different way to implement the method (try method indexOf() from the String class). 2. Modify the driver class to iteratively ask the user to enter a sentence, and then translate the sentence, until the user decides to quit the program. import java.util.Scanner;public class Translator { //instance variables //constructors //methods public static String translate(String sentence)// My name is Patrick Casseus { String result = ""; sentence = sentence.toLowerCase(); Scanner scan = new Scanner(sentence); while(scan.hasNext()) { result = result += translateWord(scan.next()) + " "; } return result; } private static String translateWord(String word) { String result = ""; if(startWithVowel(word)) result = word + "yay"; else if(startWithBlend(word)) // chair -> air + ch + ay result = word.substring(2) +…arrow_forward
- The LocalDate class includes an instance method named lengthOfMonth() that returns the number of days in the month. Write an application that uses methods in the LocalDate class to calculate how many days are left until the first day of next month. Display the result in accordance with this example: There are 16 days until MAY starts. import java.time.*; import java.util.Scanner; public class DaysTilNextMonth { publicstaticvoidmain(String[] args) { // Write your code here } }arrow_forwardTrue or False: When numerous parameters are supplied to a method, the order in which they are passed is irrelevant.arrow_forwardpublic float method1(int z) { return (float) z; } // in another class we created an object of the first class and within in it: int y = tester.method1(); are there any errors ? with explanation pleasearrow_forward
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
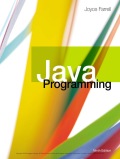
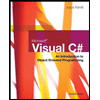