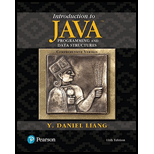
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Expert Solution & Answer
Chapter 20.7, Problem 20.7.2CP
Explanation of Solution
Collections class:
- The Collections class has static methods to do the common operations in collection and list.
- There are various static methods in Collections class.
- For collection, the static methods are max(), min(), disjoint(), and frequency().
- For list, the static methods are sort(), binarySearch(), reverse(), shuffle(), copy(), and fill().
- All the methods such as max(), min(), disjoint(), and frequency() in collections which is used for lists...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.
Draw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Chapter 20 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 20.2 - Prob. 20.2.1CPCh. 20.2 - Prob. 20.2.2CPCh. 20.2 - Prob. 20.2.3CPCh. 20.2 - Prob. 20.2.4CPCh. 20.2 - Prob. 20.2.5CPCh. 20.3 - Prob. 20.3.1CPCh. 20.3 - Prob. 20.3.2CPCh. 20.3 - Prob. 20.3.3CPCh. 20.3 - Prob. 20.3.4CPCh. 20.4 - Prob. 20.4.1CP
Ch. 20.4 - Prob. 20.4.2CPCh. 20.5 - Prob. 20.5.1CPCh. 20.5 - Suppose list1 is a list that contains the strings...Ch. 20.5 - Prob. 20.5.3CPCh. 20.5 - Prob. 20.5.4CPCh. 20.5 - Prob. 20.5.5CPCh. 20.6 - Prob. 20.6.1CPCh. 20.6 - Prob. 20.6.2CPCh. 20.6 - Write a lambda expression to create a comparator...Ch. 20.6 - Prob. 20.6.4CPCh. 20.6 - Write a statement that sorts an array of Point2D...Ch. 20.6 - Write a statement that sorts an ArrayList of...Ch. 20.6 - Write a statement that sorts a two-dimensional...Ch. 20.6 - Write a statement that sorts a two-dimensional...Ch. 20.7 - Are all the methods in the Collections class...Ch. 20.7 - Prob. 20.7.2CPCh. 20.7 - Show the output of the following code: import...Ch. 20.7 - Prob. 20.7.4CPCh. 20.7 - Prob. 20.7.5CPCh. 20.7 - Prob. 20.7.6CPCh. 20.8 - Prob. 20.8.1CPCh. 20.8 - Prob. 20.8.2CPCh. 20.8 - Prob. 20.8.3CPCh. 20.9 - How do you create an instance of Vector? How do...Ch. 20.9 - How do you create an instance of Stack? How do you...Ch. 20.9 - Prob. 20.9.3CPCh. 20.10 - Prob. 20.10.1CPCh. 20.10 - Prob. 20.10.2CPCh. 20.10 - Prob. 20.10.3CPCh. 20.11 - Can the EvaluateExpression program evaluate the...Ch. 20.11 - Prob. 20.11.2CPCh. 20.11 - If you enter an expression "4 + 5 5 5", the...Ch. 20 - (Display words in ascending alphabetical order)...Ch. 20 - (Store numbers in a linked list) Write a program...Ch. 20 - (Guessing the capitals) Rewrite Programming...Ch. 20 - (Sort points in a plane) Write a program that...Ch. 20 - (Combine colliding bouncing balls) The example in...Ch. 20 - (Game: lottery) Revise Programming Exercise 3.15...Ch. 20 - Prob. 20.9PECh. 20 - Prob. 20.10PECh. 20 - (Match grouping symbols) A Java program contains...Ch. 20 - Prob. 20.12PECh. 20 - Prob. 20.14PECh. 20 - Prob. 20.16PECh. 20 - (Directory size) Listing 18.10,...Ch. 20 - Prob. 20.20PECh. 20 - (Nonrecursive Tower of Hanoi) Implement the...Ch. 20 - Evaluate expression Modify Listing 20.12,...
Knowledge Booster
Similar questions
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
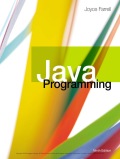
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
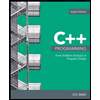
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
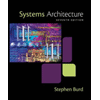
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
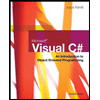
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
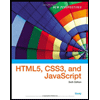
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning