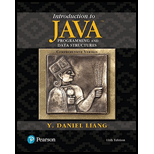
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 20, Problem 20.12PE
Program Plan Intro
Clone PriorityQueue
- Import the required packages into the program.
- In the Exercise20_12 class,
- In the main() method,
- Create two objects for Priority Queue.
- Add the items into the queue.
- Create another object for Priority queue by clone() method.
- Display the result.
- In the main() method,
- In the MyPriorityQueue() class,
- Define the clone() method.
- Create an object for MyPriorityQueue.
- Loop execute until the item. If yes,
- Call the offer() method.
- Return the queue.
- Define the clone() method.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
JAVA CODE
Learning Objectives: Detailed understanding of the linked list and its implementation. Practice with inorder sorting. Practice with use of Java exceptions. Practice use of generics.
You have been provided with java code for SomeList<T> class. This code is for a general linked list implementation where the elements are not ordered. For this assignment you will modify the code provided to create a SortedList<T> class that will maintain elements in a linked list in ascending order and allow the removal of objects from both the front and back. You will be required to add methods for inserting an object in order (InsertInorder) and removing an object from the front or back. You will write a test program, ListTest, that inserts 25 random integers, between 0 and 100, into the linked list resulting in an in-order list. Your code to remove an object must include the exception NoSuchElementException. Demonstrate your code by displaying the ordered linked list and…
3. Define a Pair class using Java Generics framework
a. Properties: first, second
b. Constructors: Create 2 different constructor with different initialization parameters
c. Methods: setFirst(), setSecond(), getFirst(), getSecond();
d. Test Pair class print out the value of Pair instance:
i. Define a Pair instance and assign (10,10.1) to the pair;
ii.
Define a Pair instance and assign (8.2, "ABC") to the pair;
iii. Define an array holding[] of Pair class.
1. holding has 100 elements;
2.
Assign (int, double) to array by using loop -> (0, 100.0), (1, 99.0), (2, 98.0), ...,
(99, 1.0).
Use Python for this question. Also please comment what each line of code means for this question as well:
Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using Note that this means you may be able to inherit some of the methods below. Which ones? (Try not writing those and see if it works!)
Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top.
push() – take an item as input and push it on the top of the stack
pop() – remove and return the item at the top of the stack
isEmpty() – returns True if the stack is empty,…
Chapter 20 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 20.2 - Prob. 20.2.1CPCh. 20.2 - Prob. 20.2.2CPCh. 20.2 - Prob. 20.2.3CPCh. 20.2 - Prob. 20.2.4CPCh. 20.2 - Prob. 20.2.5CPCh. 20.3 - Prob. 20.3.1CPCh. 20.3 - Prob. 20.3.2CPCh. 20.3 - Prob. 20.3.3CPCh. 20.3 - Prob. 20.3.4CPCh. 20.4 - Prob. 20.4.1CP
Ch. 20.4 - Prob. 20.4.2CPCh. 20.5 - Prob. 20.5.1CPCh. 20.5 - Suppose list1 is a list that contains the strings...Ch. 20.5 - Prob. 20.5.3CPCh. 20.5 - Prob. 20.5.4CPCh. 20.5 - Prob. 20.5.5CPCh. 20.6 - Prob. 20.6.1CPCh. 20.6 - Prob. 20.6.2CPCh. 20.6 - Write a lambda expression to create a comparator...Ch. 20.6 - Prob. 20.6.4CPCh. 20.6 - Write a statement that sorts an array of Point2D...Ch. 20.6 - Write a statement that sorts an ArrayList of...Ch. 20.6 - Write a statement that sorts a two-dimensional...Ch. 20.6 - Write a statement that sorts a two-dimensional...Ch. 20.7 - Are all the methods in the Collections class...Ch. 20.7 - Prob. 20.7.2CPCh. 20.7 - Show the output of the following code: import...Ch. 20.7 - Prob. 20.7.4CPCh. 20.7 - Prob. 20.7.5CPCh. 20.7 - Prob. 20.7.6CPCh. 20.8 - Prob. 20.8.1CPCh. 20.8 - Prob. 20.8.2CPCh. 20.8 - Prob. 20.8.3CPCh. 20.9 - How do you create an instance of Vector? How do...Ch. 20.9 - How do you create an instance of Stack? How do you...Ch. 20.9 - Prob. 20.9.3CPCh. 20.10 - Prob. 20.10.1CPCh. 20.10 - Prob. 20.10.2CPCh. 20.10 - Prob. 20.10.3CPCh. 20.11 - Can the EvaluateExpression program evaluate the...Ch. 20.11 - Prob. 20.11.2CPCh. 20.11 - If you enter an expression "4 + 5 5 5", the...Ch. 20 - (Display words in ascending alphabetical order)...Ch. 20 - (Store numbers in a linked list) Write a program...Ch. 20 - (Guessing the capitals) Rewrite Programming...Ch. 20 - (Sort points in a plane) Write a program that...Ch. 20 - (Combine colliding bouncing balls) The example in...Ch. 20 - (Game: lottery) Revise Programming Exercise 3.15...Ch. 20 - Prob. 20.9PECh. 20 - Prob. 20.10PECh. 20 - (Match grouping symbols) A Java program contains...Ch. 20 - Prob. 20.12PECh. 20 - Prob. 20.14PECh. 20 - Prob. 20.16PECh. 20 - (Directory size) Listing 18.10,...Ch. 20 - Prob. 20.20PECh. 20 - (Nonrecursive Tower of Hanoi) Implement the...Ch. 20 - Evaluate expression Modify Listing 20.12,...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- - the constructor needs to initialize tailPtr to nullptr - insert(): modify it to update prev pointers as well as next pointers. - remove(): modify to update prev pointers as well as next pointers. Add a new public member function to the LinkedList class named reverse() which reverses the items in the list. swap each node’s prev/next pointers, and finally swap headPtr/tailPtr. Demonstrate your function works by creating a sample list of a few entries in main(), printing out the contents of the list, reversing the list, and then printing out the contents of the list again to show that the list has been reversed. Note: your function must actually reverse the items in the doubly-linked list, not just print them out in reverse order! we won't use the copy constructor in this assignment, and as such you aren't required to update the copy constructor to work with a doubly-linked list. @file LinkedList.cpp */ #include "LinkedList.h" // Header file#include <cassert>#include…arrow_forwardPlease use Python for this question: 1. Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using inheritance. Note that this means you may be able to inherit some of the methods below: a. Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top. b. push() – take an item as input and push it on the top of the stack c. pop() – remove and return the item at the top of the stack d. isEmpty() – returns True if the stack is empty, False otherwise e. [] – return the item at a given location, [0] is at the bottom of the stack f.…arrow_forward(Please Help. My professor did not teach us any of this and will not answer my emails) Design a class named Queue for storing integers. Like a stack, a queue holds elements. In a stack, the elements are retreived in a last-in-first-out fashion. In a queue, the elements are retrieved in a first-in-first-out fashion. The class contains: An int[] data field named elements that stores the int values in the queue A data field named size that stores the number of elements in the queue A constructor that creates a Queue object with defult capacity 8 The method enqueue(int v) that adds v into the queue The method empty () that returns true if the queue is empty The method getSize() that returns the size of the queuearrow_forward
- Simple queue implementation please help (looks like a lot but actually isnt, everything is already set up for you to make it even easier) help in any part you can, please be clear, thank you Given an interface for Queue- Without using the java collections interface (ie do not import java.util.List,LinkedList, Stack, Queue...)- Create an implementation of Queue interface provided- For the implementation create a tester to verify the implementation of thatdata structure performs as expected Wait in line – Queue (fifo)- Implement the provided Queue interface ( fill out the implementation shell)- Put your implementation through its paces by exercising each of the methods ina test harness- Add to your ‘BusClient’ the following functionality using your Queue-o Create (enqueue) 6 riders by name§ Iterate over the queue, print all riderso Peek at the queue / print the resulto Remove (dequeue) the head of the queue§ Iterate over the queue, print all riderso Add two more riders to the queueo Peek…arrow_forwardProject 4: Maze Solver (JAVA) The purpose of this assignment is to assess your ability to: Implement stack and queue abstract data types in JAVA Utilize stack and queue structures in a computational problem. For this question, implement a stack and a queue data structure. After testing the class, complete the depth-first search method (provided). The method should indicate whether or not a path was found, and, in the case that a path is found, output the sequence of coordinates from start to end. The following code and related files are provided. Carefully ready the code and documentation before beginning. A MazeCell class that models a cell in the maze. Each MazeCell object contains data for the row and column position of the cell, the next direction to check, and a bool variable that indicates whether or not the cell has already been visited. A Source file that creates the maze and calls your depth-first search method. An input file, maze.in, that may be used for testing. You…arrow_forwardQuestion #7. SORTED LIST NOT UNSORTED C++ The specifications for the Sorted List ADT state that the item to be deleted is in the list. • Rewrite the specification for Deleteltem so that the list is unchanged if the item to be deleted is not in the list. • Implement Deleteltem as specified in (a) using an array-based. Implement Deleteltem as specified in (a) using a linked implementation. • Rewrite the specification for Deleteltem so that all copies of the item to be deleted are removed if they exist. • Implement Deleteltem as specified in (d) using an array-based. • Implement Deleteltem as specified in (d) using a linked implementation.arrow_forward
- Language: C++ Note: Write both parts separately. Part a: Complete the above Priority Queue class. Write a driver to test it. Part b: Modify the above class to handle ‘N’ different priority levelsarrow_forwardPlease be thorough with explanation (a) Consider implementing a stack as a class that relies on a singly linked list, maintaining pointers to both the start and end of the singly linked list in the main object. You consider two possibilities. The first is to push (insert) to the beginning and pop (remove) from the beginning of the linked list. The second is to push to the end and pop from the end of the linked list. Is one of these possibilities better than the other? Briefly explain your answer.arrow_forwardIn this project, you will develop algorithms that find road routes through the bridges to travel between islands. The input is a text file containing data about the given map. Each file begins with the number of rows and columns in the map considered as maximum latitudes and maximum longitudes respectively on the map. The character "X" in the file represents the water that means if a cell contains "X" then the traveler is not allowed to occupy that cell as this car is not drivable on water. The character "0" in the file represents the road connected island. That means if a cell contains "0" then the traveler is allowed to occupy that cell as this car can drive on roads. The traveler starts at the island located at latitude = 0 and longitude = 0 (i.e., (0,0)) in the upper left comer, and the goal is to drive to the island located at (MaxLattitude-1, MaxLongitudes-1) in the lower right corner. A legal move from an island is to move left, right, up, or down to an immediately adjacent cell…arrow_forward
- In this project, you will develop algorithms that find road routes through the bridges to travel between islands. The input is a text file containing data about the given map. Each file begins with the number of rows and columns in the map considered as maximum latitudes and maximum longitudes respectively on the map. The character "X" in the file represents the water that means if a cell contains "X" then the traveler is not allowed to occupy that cell as this car is not drivable on water. The character "0" in the file represents the road connected island. That means if a cell contains "O" then the traveler is allowed to occupy that cell as this car can drive on roads. The traveler starts at the island located at latitude = 0 and longitude = 0 (i.e., (0,0)) in the upper left corner, and the goal is to drive to the island located at (MaxLattitude-1, MaxLongitudes-1) in the lower right corner. A legal move from an island is to move left, right, up, or down to an immediately adjacent…arrow_forwardStackQueuePostfix A. Pointer_based queuea. Define the class PoiQueue with no implementation; i.e. declare the datamembers, and the function members only (Enqueue, Dequeue, IsEmpty,GetHead etc.).b. Implement the Enqueue method of the above classB. Array_based non-circular queue:a. Define the class Queue using one dimensional array representation with noimplementation; i.e. declare the data members, and the function membersonly (Enqueue, Dequeue, IsEmpty, GetHead etc.).b. Implement the Denqueue method of the above classarrow_forwardPlease answer the following question in Python code: Inheritance (based on 8.38) You MUST use inheritance for this problem. A stack is a sequence container type that, like a queue, supports very restrictive access methods: all insertions and removals are from one end of the stack, typically referred to as the top of the stack. A stack is often referred to as a last-in first-out (LIFO) container because the last item inserted is the first removed. Implement a Stack class using Note that this means you may be able to inherit some of the methods below. Which ones? (Try not writing those and see if it works!) Constructor/_init__ - Can construct either an empty stack, or initialized with a list of items, the first item is at the bottom, the last is at the top. push() – take an item as input and push it on the top of the stack pop() – remove and return the item at the top of the stack isEmpty() – returns True if the stack is empty, False otherwise [] – return the item at a given…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
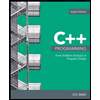
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning