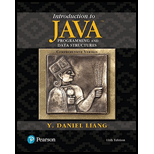
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 20, Problem 20.9PE
Program Plan Intro
Program to remove the first largest ball
Program plan:
- Import the required packages into the program.
- In the Exercise20_09 class,
- In start() method,
- Create an object for buttons and HBox.
- Call addAll() method to add all buttons.
- Set the action adds or remove a ball.
- Pause and restart animation.
- Scroll bar used to control speed of animation.
- Set the maximum speed.
- Create a scene, put it into pane in stage, and display the stage.
- Define the MultipleBallPane class,
- Define the add() method to add the ball.
- Define the subtract() method to subtract the ball.
- Define the play() method to play the ball.
- Define the pause() method to pause the ball.
- Define the increaseSpeed() method to increase the speed.
- Define the decreaseSpeed() method to decrease the speed.
- Define the DoubleProperty() method to return the rate property.
- Define the moveBall() method to adjust the ball position and identify the collision.
- In start() method,
- In the Ball class extends the Circle class.
- Declare the required variables.
- Define compareTo() method to return the value.
- In the main() method,
- Launch the project.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Find the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714V
Resolver por superposicion
Describe three (3) Multiplexing techniques common for fiber optic links
Chapter 20 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 20.2 - Prob. 20.2.1CPCh. 20.2 - Prob. 20.2.2CPCh. 20.2 - Prob. 20.2.3CPCh. 20.2 - Prob. 20.2.4CPCh. 20.2 - Prob. 20.2.5CPCh. 20.3 - Prob. 20.3.1CPCh. 20.3 - Prob. 20.3.2CPCh. 20.3 - Prob. 20.3.3CPCh. 20.3 - Prob. 20.3.4CPCh. 20.4 - Prob. 20.4.1CP
Ch. 20.4 - Prob. 20.4.2CPCh. 20.5 - Prob. 20.5.1CPCh. 20.5 - Suppose list1 is a list that contains the strings...Ch. 20.5 - Prob. 20.5.3CPCh. 20.5 - Prob. 20.5.4CPCh. 20.5 - Prob. 20.5.5CPCh. 20.6 - Prob. 20.6.1CPCh. 20.6 - Prob. 20.6.2CPCh. 20.6 - Write a lambda expression to create a comparator...Ch. 20.6 - Prob. 20.6.4CPCh. 20.6 - Write a statement that sorts an array of Point2D...Ch. 20.6 - Write a statement that sorts an ArrayList of...Ch. 20.6 - Write a statement that sorts a two-dimensional...Ch. 20.6 - Write a statement that sorts a two-dimensional...Ch. 20.7 - Are all the methods in the Collections class...Ch. 20.7 - Prob. 20.7.2CPCh. 20.7 - Show the output of the following code: import...Ch. 20.7 - Prob. 20.7.4CPCh. 20.7 - Prob. 20.7.5CPCh. 20.7 - Prob. 20.7.6CPCh. 20.8 - Prob. 20.8.1CPCh. 20.8 - Prob. 20.8.2CPCh. 20.8 - Prob. 20.8.3CPCh. 20.9 - How do you create an instance of Vector? How do...Ch. 20.9 - How do you create an instance of Stack? How do you...Ch. 20.9 - Prob. 20.9.3CPCh. 20.10 - Prob. 20.10.1CPCh. 20.10 - Prob. 20.10.2CPCh. 20.10 - Prob. 20.10.3CPCh. 20.11 - Can the EvaluateExpression program evaluate the...Ch. 20.11 - Prob. 20.11.2CPCh. 20.11 - If you enter an expression "4 + 5 5 5", the...Ch. 20 - (Display words in ascending alphabetical order)...Ch. 20 - (Store numbers in a linked list) Write a program...Ch. 20 - (Guessing the capitals) Rewrite Programming...Ch. 20 - (Sort points in a plane) Write a program that...Ch. 20 - (Combine colliding bouncing balls) The example in...Ch. 20 - (Game: lottery) Revise Programming Exercise 3.15...Ch. 20 - Prob. 20.9PECh. 20 - Prob. 20.10PECh. 20 - (Match grouping symbols) A Java program contains...Ch. 20 - Prob. 20.12PECh. 20 - Prob. 20.14PECh. 20 - Prob. 20.16PECh. 20 - (Directory size) Listing 18.10,...Ch. 20 - Prob. 20.20PECh. 20 - (Nonrecursive Tower of Hanoi) Implement the...Ch. 20 - Evaluate expression Modify Listing 20.12,...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Could you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forwardFor this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward
- 1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forwarda) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forward
- using r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardusing r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
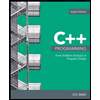
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
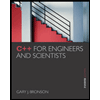
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
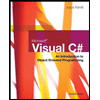
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
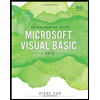
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning