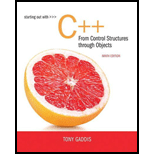
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 9PC
Program Plan Intro
File Reverser
Program Plan:
Main.cpp:
- Include required header files.
- Inside “main ()” function,
- Create an object “file1” for input file stream.
- Create an object “file2” for output file stream.
- Declare a class template
- Declare character variables named “popChar”, and “ch”.
- Till the end of file,
- Push each character into the stack using the function “push ()”.
- Close “file1”.
- Until the stack gets empty,
- Pop the character stack and write it on “file2” using the function “pop ()”.
- Close “file2”.
- Open “file2”.
- Till the end of file,
- Get a character and print it on the console screen.
DynStack.h:
- Include required header files.
- Create a template.
- Declare a class named “DynStack”. Inside the class
- Inside the “private” access specifier,
- Give structure declaration for the stack
- Create an object for the template
- Create a stack pointer name “next”.
- Create a stack pointer name “top”
- Give structure declaration for the stack
- Inside the “public” access specifier,
- Give a declaration for a constructor.
- Assign null to the top node.
- Give function declaration for “push ()”, “pop ()”,and “isEmpty ()”.
- Give a declaration for a constructor.
- Inside the “private” access specifier,
- Give the class template.
- Give function definition for “push ()”.
- Assign null to the new node.
- Dynamically allocate memory for new node
- Assign “num” to the value of new node.
- Check if the stack is empty using the function “isEmpty ()”
- If the condition is true then assign new node as the top and make the next node as null.
- If the condition is not true then, assign top node to the next of new node and assign new node as the top.
- Give the class template.
- Give function definition for “pop ()”.
- Assign null to the temp node.
- Check if the stack is empty using the function “is_Empty ()”
- If the condition is true then print “The stack is empty”.
- If the condition is not true then,
- Assign top value to the variable “num”.
- Link top of next node to temp node.
- Delete the top node and make temp as the top node.
- Give function definition for “isEmpty ()”.
- Assign Boolean value to the variable
- Check if the top node is null
- Assign true to “status”.
- Return the status
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Word List File Reader
This exercise assumes you have completed the Programming Exercise 7, Word List FileWriter. Write another program that reads the words from the file and displays the following data:• The number of words in the file.• The longest word in the file.• The average length of all of the words in the file
PYTHON coding
# Method: Load levelsList using the data in levelsFile
def readLevelsFromFile(self):
try:
# Set levelsList to an empty list
pass
# Open the file
pass
# Use a loop to read through the file line by line
pass
# This code is inside the loop:
# Convert the line to a float and then append it to levelsList
pass
# Close the file
pass
except:
return
Chapter 19 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 19.1 - Describe what LIFO means.Ch. 19.1 - What is the difference between static and dynamic...Ch. 19.1 - What are the two primary stack operations?...Ch. 19.1 - What STL types does the STL stack container adapt?Ch. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - What is the difference between a static stack and...Ch. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - The STL stack is considered a container adapter....
Ch. 19 - What types may the STL stack be based on? By...Ch. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - The STL stack container is an adapter for the...Ch. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 21RQECh. 19 - Prob. 22RQECh. 19 - Prob. 23RQECh. 19 - Prob. 24RQECh. 19 - Prob. 25RQECh. 19 - Prob. 26RQECh. 19 - Write two different code segments that may be used...Ch. 19 - Prob. 28RQECh. 19 - Prob. 29RQECh. 19 - Prob. 30RQECh. 19 - Prob. 31RQECh. 19 - Prob. 32RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Dynamic String Stack Design a class that stores...Ch. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PCCh. 19 - Prob. 11PCCh. 19 - Inventory Bin Stack Design an inventory class that...Ch. 19 - Prob. 13PCCh. 19 - Prob. 14PCCh. 19 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- A-Language- Python Write a fully functioning program that correctly uses a list, reads, and writes from/to an external file. Megan owns a small neighborhood coffee shop, and she has fifteen employees who work as baristas. All of the employees have the same hourly pay rate. Megan has asked you to design a program that will allow her to enter the number of hours worked by each employee and then display the amounts of all the employees’ gross pay. You determine that the program should perform the following steps: The text that is in the file is stored as one sentence. Incorporate the code that reads the file’s contents and calculates the following: total number of words in the file total average number of words per sentence total number of characters in the file total average number of characters per sentence Use function(s) to accommodate this word and character analysis of the file B For each employee: Get the employee name from the file named employee_names.txt (attached in…arrow_forwardIn c language,using pointer and for loop Code level: Beginnersarrow_forwardC++ Programming. Associative and unordered associative containers. Associative containers (set, map, multiset, multimap). • When executing a given task, the input values must be read from a text file. Task : Create a program that deletes a set of elements from a single word of a given string type and creates a second set of elements that consist of a single word, and also displays it on the screen.arrow_forward
- In python please! I am strugglingarrow_forwardCENT 110 Homework 6 This assignment involves writing a program that takes reads from a text file containing multiple lines of floating numbers separated by commas. The program will ask for the user to enter file name of the text file. The program will read each line of numbers, use the split() function to put the numbers in lists. For each line of numbers, the program will calculate the total of the numbers, the average value, the smallest number, and the largest number. For each line of numbers, it will then display each number, the total, average, smallest number, and the largest number. The numbers are in a text file named numbers.txt and contains the following: 1.0, 2.0, 3.0 4.0, 3.0, 2.0, 1.0 5.0, 5.0, 5.0, 5.0, 5.0arrow_forwardphyton Topics: list and list processing You will write a program to read grade data from a text file, displays the grades as a 5-column table, and then print the statistics (min, max, median, and median). You can assume that the input file only one number per line. You can assume the user always enter a file that exists. The median is the value in the middle of a sorted list. To sort a list, use list.sort() function. It’s computed as below. For a list of odd length, the middle number is just the length divide by 2. For the list, [1,2,3], the median is 2 since 2 is in the middle of the list. The middle index is 3//2, which is 1. Remember that // is the integer division operator. When the length of the list is even, there are two middle numbers. The median is the average of the two middle numbers. For example, [1,2,3,4], the median is (2+3)/2 = 2.5. The two middle indexes for this example are (4//2)-1 = 1 and (4//2) = 2. Functions you need to write: read_grades() Prompts…arrow_forward
- File System: It is highly useful in file system handling where for example the file allocation table contains a sequential list of locations where the files is split up and stored on a disk. Remember that overtime it is hard for an OS to find disk space to cover the entire file so it usually splits these up into chunks across the physical hard drive and stores a sequential list of links together as a linked list. Write an algorithm for the above problem and analyse the efficiency of the algorithm.arrow_forwardA file object’s writelines method automatically writes a newline ('\n') after writing each list item to the file.True or Falsearrow_forwardC++ Add a search command that asks the user for a string and finds the first line that contains that string. The search starts at the first line currently displayed and stops at the end of the file. If the string is found, the line that contains it is displayed at the top. In case the user enters a string X that does not occur in the file, the program should print the error message: ERROR: string "X" was not found.arrow_forward
- C++ Create a program that reads a file containing a list of songs and prints the songs to the screen one at a time. After each song is printed, except for the last song, the program asks the user to press enter for more. After the last song, the program should say that this was the last song and quit. If there were no songs in the file to begin with, the program should say that there are no songs to show and quit. The program should begin by asking the user for the name of the input file. Each song consists of a title, artist, and year. In the file, each song is given on three consecutive lines. Create this program using a class Song.arrow_forwardC++ Add a search command that asks the user for a string and finds the first line that contains that string. The search starts at the first line currently displayed and stops at the end of the file. If the string is found, the line that contains it is displayed at the top. In case the user enters a string X that does not occur in the file, the program should print the error message: ERROR: string "X" was not found. Attached is the first lines of the .txt filearrow_forwardreads an external character file and passes it to a list variable. Returns a list containing the words in the file as the return value of the function python functions _read_file_(): text inside:vejOnVacnesdsavWurndasorsdadsadaGhahidBejAcRenfacimJsadsadaiOnsOcsGebposAfAtOcFipswoukVuvaygdyswotarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
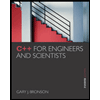
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
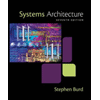
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage