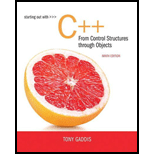
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 4PC
Program Plan Intro
Dynamic Queue Template
Program Plan:
Main.cpp:
- Include required header files.
- Inside “main ()” function,
- Declare a constant variable
- Declare a template
- Manipulate and insert elements into the queue using “enqueue ()” function.
- Delete the queue elements using “dequeue ()” function.
Dynqueue.h:
- Include required header files.
- Create template class
- Declare a class named “Dynqueue”. Inside the class,
- Inside the “private” access specifier,
- Create a structure named “QueueNode”.
- Create an object for the template
- Create a pointer named “next”.
- Create two pointers named “front” and “rear”.
- Declare a variable.
- Create a structure named “QueueNode”.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Declare the functions “enqueue ()”, “dequeue ()”, “isEmpty ()”, “isFull ()”, and “clear ()”.
- Inside the “private” access specifier,
- Declare template class.
- Give definition for the constructor.
- Assign the values.
- Declare template class.
- Give definition for the destructor.
- Call the function “clear ()”.
- Declare template class.
- Give function definition for “enqueue ()”.
- Make the pointer “newNode” as null.
- Assign “num” to newNode->value.
- Make newNode->next as null.
- Check whether the queue is empty using “isEmpty ()” function.
- If the condition is true then, assign newNode to “front” and “rear”.
- If the condition is not true then,
- Assign newNode to rear->next
- Assign newNode to “rear”.
- Increment the variable “numItems”.
- Declare template class.
- Give function definition for “dequeue ()”.
- Assign temp pointer as null.
- Check if the queue is empty using “isEmpty ()” function.
- If the condition is true then print “The queue is empty”.
- If the condition is not true then,
- Assign the value of front to the variable “num”.
- Make front->next as “temp”.
- Delete the front value
- Make temp as front.
- Decrement the variable “numItems”.
- Declare template class.
- Give function definition for “isEmpty ()”.
- Assign “true” to a Boolean variable
- Check if “numItems” is true.
- If the condition is true then assign “false” to the variable.
- Return the Boolean variable.
- Declare template class.
- Give function definition for “clear ()”.
- Create an object for template.
- Dequeue values from queue till the queue becomes empty using “while” condition.
- Create an object for template.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
c++
Write a client function that returns the back of a queue while leaving the queue unchanged. This function can call any of the methods of the ADT queue. It can also create new queues. The return type is ITemType, and it accepts a queue as a parameter.
C++ ProgrammingActivity: Queue Linked List
Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow.
#include "queue.h"
#include "linkedlist.h"
class SLLQueue : public Queue {
LinkedList* list;
public:
SLLQueue() {
list = new LinkedList();
}
void enqueue(int e) {
list->addTail(e);
return;
}
int dequeue() {
int elem;
elem = list->removeHead();
return elem;
}
int first() {
int elem;
elem = list->get(1);
return elem;;
}
int size() {
return list->size();
}
bool isEmpty() {
return list->isEmpty();
}
int collect(int max) {
int sum = 0;
while(first() != 0) {
if(sum + first() <= max) {
sum += first();
dequeue();
} else {…
C++ ProgrammingActivity: Queue Linked List
Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow.
SEE ATTACHED PHOTO FOR THE PROBLEM
#include "queue.h"
#include "linkedlist.h"
class SLLQueue : public Queue {
LinkedList* list;
public:
SLLQueue() {
list = new LinkedList();
}
void enqueue(int e) {
list->addTail(e);
return;
}
int dequeue() {
int elem;
elem = list->removeHead();
return elem;
}
int first() {
int elem;
elem = list->get(1);
return elem;;
}
int size() {
return list->size();
}
bool isEmpty() {
return list->isEmpty();
}
int collect(int max) {
int sum = 0;
while(first() != 0) {
if(sum + first() <= max) {
sum += first();…
Chapter 19 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 19.1 - Describe what LIFO means.Ch. 19.1 - What is the difference between static and dynamic...Ch. 19.1 - What are the two primary stack operations?...Ch. 19.1 - What STL types does the STL stack container adapt?Ch. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - What is the difference between a static stack and...Ch. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - The STL stack is considered a container adapter....
Ch. 19 - What types may the STL stack be based on? By...Ch. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - The STL stack container is an adapter for the...Ch. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 21RQECh. 19 - Prob. 22RQECh. 19 - Prob. 23RQECh. 19 - Prob. 24RQECh. 19 - Prob. 25RQECh. 19 - Prob. 26RQECh. 19 - Write two different code segments that may be used...Ch. 19 - Prob. 28RQECh. 19 - Prob. 29RQECh. 19 - Prob. 30RQECh. 19 - Prob. 31RQECh. 19 - Prob. 32RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Dynamic String Stack Design a class that stores...Ch. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PCCh. 19 - Prob. 11PCCh. 19 - Inventory Bin Stack Design an inventory class that...Ch. 19 - Prob. 13PCCh. 19 - Prob. 14PCCh. 19 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Computer science JAVA programming languagearrow_forwardCourse: Data Structure and Algorithms Language: C++ Question is well explained Question #2Implement a class for Circular Doubly Linked List (with a dummy header node) which stores integers in unsorted order. Your class definitions should look like as shown below: class CDLinkedList;class DNode {friend class CDLinkedList;private int data;private DNode next;private DNode prev;};class CDLinkedList {private:DNode head; // Dummy header nodepublic CDLinkedList(); // Default constructorpublic bool insert (int val); public bool removeSecondLastValue (); public void findMiddleValue(); public void display(); };arrow_forwardIn C++ Pleasearrow_forward
- 1 Implement a Queue Data Structure specifically to store integer data using a Singly Linked List. 2 The data members should be private. 3 You need to implement the following public functions: 4 1. Constructor: 5 It initialises the data members as required. 6 7 8 2. enqueue(data) : This function should take one argument of type integer. It enqueues the element into the queue and returns nothing. 3. dequeue(): It dequeues/removes the element from the front of the queue and in turn, returns the element being dequeued or removed. In case the queue is empty, it r 4. front (): 10 11 It returns the element being kept at the front of the queue. In case the queue is empty, it returns -1. 12 5. getSize(): 13 It returns the size of the queue at any given instance of time. 14 6. 1sEmpty(): 15 It returns a boolean value indicating whether the queue is empty or not. 16 Operations Performed on the Stack: 17 Query-1 (Denoted by an integer 1): Enqueues an integer data to the queue. 18 19 Query-2…arrow_forwardLINKED LIST IMPLEMENTATION Linked list Write a C++ program to implement insertion, deletion, and display operations in a Linked List Strictly adhere to the Object-Oriented specifications given in the problem statement. All class names, member variable names, and function names should be the same as specified in the problem statement.The class Node has the following member variable Datatype Variable Usage int data to store data Node* next to store the next node Define the following public member functions in the class LinkedList. Member function Function description void insertNode(int value) This function inserts the data into the linked list at the end void deleteNode(int value) This function deletes the node from the linked list void display() This function is used to display the nodes in the linked list In the main() function, read inputs and call the functions of the LinkedList class based on the inputs. Note: If the Linked list is empty while…arrow_forwardExplain the process of Linking with Static Libraries ?arrow_forward
- X1222: Double Ended Queue: Deque A double ended queue, known as deque, is a queue data structure that allows adding and removing elements from both ends of the queue. Instead of enqueue and dequeue, it has insert, delete, and get for both front and last of the queue as shown below. The data stored internally is stored in a ListNodesPlus object. The basic class definition is shown below: public class Deque { private ListNodePlus elements; // code ommitted for space public void clear() {...}; public int numElements () {...}; public boolean isEmpty() {...}; ● // Implement the following four methods public void insertFront (E it) { } public E deleteFront () { } public void insertLast (E it) { } public E deleteLast() { } Write the following four methods: • insertFront (E it) takes it and adds it to the front of the queue. stored internally in elements. The front of the queue is defined as position 0 in the queue. ● deleteFront () removes the element at the front of the queue and returns it.…arrow_forwardWrite JAVA code General Problem Description: It is desired to develop a directory application based on the use of a double-linked list data structure, in which students are kept in order according to their student number. In this context, write the Java codes that will meet the requirements given in detail below. Requirements: Create a class named Student to represent students. In the Student class; student number, name and surname and phone numbers for communication are kept. Student's multiple phones number (multiple mobile phones, home phones, etc.) so phone numbers information will be stored in an “ArrayList”. In the Student class; parameterless, taking all parameters and It is sufficient to have 3 constructor methods, including a copy constructor, get/set methods and toString.arrow_forwardC++ Queue LinkedList, Refer to the codes I've made as a guide and add functions where it can add/insert/remove function to get the output of the program, refer to the image as an example of input & output. Put a comment or explain how the program works/flow.arrow_forward
- # Create an object from the Queue class. # Queue (with a capital Q) is the class name # queue (with a small q) is the object # created from the "Queue" classarrow_forwardLanguage: C++ Note: Write both parts separately. Part a: Complete the above Priority Queue class. Write a driver to test it. Part b: Modify the above class to handle ‘N’ different priority levelsarrow_forwardComputer science JAVA programming question i need help with this entire question pleasearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
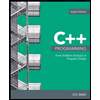
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning