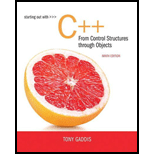
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 7PC
Program Plan Intro
Dynamic MathStack
Program Plan:
MathStack.h:
- Include required header files
- Declare a class named “MathStack”. Inside the class,
- Inside “public” access specifier,
- Declare functions “add ()”, “sub ()”, “mult ()”, “div ()”, “addAll ()”, and “multAll ()”.
- Inside “public” access specifier,
MathStack.cpp:
- Include required header files.
- Give function definition to add elements “add()”.
- Declare required variables “number”, and “sum_Value”.
- Call the function “pop()”.
- Add the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to subtract elements “sub()”.
- Declare required variables “number”, and “diff_Value”.
- Call the function “pop()”.
- Subtract the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to multiply elements “mult()”.
- Declare required variables “number”, and “prod_Value”.
- Call the function “pop()”.
- Multiply the elements.
- Push the value into the stack using the function “push ()”.
- Give function definition to divide elements “div()”.
- Declare required variables “number”, and “quo_Value”.
- Call the function “pop()”.
- Divide the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to add all the elements “addAll()”.
- Declare required variables “number”, and “sum_Value”.
- Call the function “pop()”.
- Add all the elements.
- Push the value into the stack using the function “push()”.
- Give function definition to multiply all the elements “multAll()”.
- Declare required variables “number”, and “prod_Value”.
- Call the function “pop()”.
- Multiply all the elements.
- Push the value into the stack using the function “push()”.
IntStack.h:
- Include required files.
- Declare a class named “IntStack”. Inside the class,
- Inside “protected” access specifier,
- Declare a pointer named “stackArray”.
- Declare variables “stackSize” and “top”.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Give function declarations.
- Inside “protected” access specifier,
IntStack.cpp:
- Declare required header files.
- Give definition for constructor,
- Create a stack array and assign the size
- Give definition for destructor,
- Delete the array and assign it to null
- Give function definition to push elements “push()”
- Check if the stack is full using the function “isFull()”,
- If the condition is true then, print “The stack is full”.
- If the condition is not true then,
- Increment the variable.
- Assign “num” to the top position.
- Check if the stack is full using the function “isFull()”,
- Give function definition to pop elements “pop ()”,
- Check if the stack is empty using the function “isEmpty()”.
- If the condition is true then, print “The stack is empty”.
- Assign top element to the variable.
- Decrement the variable.
- If the condition is true then, print “The stack is empty”.
- Check if the stack is empty using the function “isEmpty()”.
- Give function definition to check if the stack is full “isFull()”.
- Assign “false” to the Boolean variable “status”.
- Check if the stack size if full.
- Assign true
- Return the Boolean variable “status”.
- Give function definition to check if the stack is empty “isEmpty()”.
- Assign “false” to the Boolean variable
- Check if top is empty
- Assign true.
- Return the variable
Main.cpp:
- Include required header files.
- Inside “main ()” function,
- Declare constant variables “STACKSIZE”, “ADDSIZE”, and “MULTSIZE”.
- Create three stacks “stack”, “addAllStack”, and “multAllStack”.
- Declare a variable “popVar”.
- Push two elements to perform add operation.
- Call the function “add()”.
- Display the element.
- Push two elements to perform multiplication operation.
- Call the function “mult()”.
- Display the element.
- Push two elements to perform division operation.
- Call the function “div()”.
- Display the element.
- Push two elements to perform subtraction operation.
- Call the function “sub()”.
- Display the element.
- Push four elements to perform addAll operation.
- Call the function “addAll()”.
- Display the element.
- Push six elements to perform multAll operation.
- Call the function “multAll()”.
- Display the element.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Problem Statement
You are working as a Devops Administrator.
Y
ou’ve been
t
asked to deploy a multi
-
tier application on Kubernetes Cluster. The application is a NodeJS application
available on Docker Hub with the following name:
d
evopsedu/emp
loyee
This Node
JS application works with a mongo database. MongoDB
image
is available
on
D
ockerHub with the following name:
m
ongo
You are required to deploy this application on Kubernetes:
•
NodeJS is available on port 8888 in the container
and will be reaching out to por
t
27017 for mongo database connection
•
MongoDB will be accepting connections on
port 27017
You must deploy this application using the CL
I
.
Once your application is up and running, ensure you can add an employee from the
NodeJS application and verify by
going to Get Employee page and retrieving your input.
Hint:
Name the Mongo DB Service and deployment, specifically as “mongo”.
I need help in server client project. It is around 1200 lines of code in both . I want to meet with the expert online because it is complicated. I want the server send a menu to the client and the client enters his choice and keep on this until the client chooses to exit . the problem is not in the connection itself as far as I know.I tried while loops but did not work. please help its emergent
I need help in my server client in C language
Chapter 19 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 19.1 - Describe what LIFO means.Ch. 19.1 - What is the difference between static and dynamic...Ch. 19.1 - What are the two primary stack operations?...Ch. 19.1 - What STL types does the STL stack container adapt?Ch. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - What is the difference between a static stack and...Ch. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - The STL stack is considered a container adapter....
Ch. 19 - What types may the STL stack be based on? By...Ch. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - The STL stack container is an adapter for the...Ch. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 21RQECh. 19 - Prob. 22RQECh. 19 - Prob. 23RQECh. 19 - Prob. 24RQECh. 19 - Prob. 25RQECh. 19 - Prob. 26RQECh. 19 - Write two different code segments that may be used...Ch. 19 - Prob. 28RQECh. 19 - Prob. 29RQECh. 19 - Prob. 30RQECh. 19 - Prob. 31RQECh. 19 - Prob. 32RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Dynamic String Stack Design a class that stores...Ch. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PCCh. 19 - Prob. 11PCCh. 19 - Inventory Bin Stack Design an inventory class that...Ch. 19 - Prob. 13PCCh. 19 - Prob. 14PCCh. 19 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Exercise docID document text docID document text 1 hot chocolate cocoa beans 7 sweet sugar 2345 9 cocoa ghana africa 8 sugar cane brazil beans harvest ghana 9 sweet sugar beet cocoa butter butter truffles sweet chocolate 10 sweet cake icing 11 cake black forest Clustering by k-means, with preprocessing tokenization, term weighting TFIDF. Manhattan Distance. Number of cluster is 2. Centroid docID 2 and docID 9.arrow_forwardChange the following code so that there is always at least one way to get from the left corner to the top right, but the labyrinth is still randomized. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. Take care that the player and the dragon cannot start off on walls. Also the dragon starts off from a randomly chosen position public class Labyrinth { private final int size; private final Cell[][] grid; public Labyrinth(int size) { this.size = size; this.grid = new Cell[size][size]; generateLabyrinth(); } private void generateLabyrinth() { Random rand = new Random(); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { // Randomly create walls and paths grid[i][j] = new Cell(rand.nextBoolean()); } } // Ensure start and end are…arrow_forwardChange the following code so that it checks the following 3 conditions: 1. there is no space between each cells (imgs) 2. even if it is resized, the components wouldn't disappear 3. The GameGUI JPanel takes all the JFrame space, so that there shouldn't be extra space appearing in the frame other than the game. Main(): Labyrinth labyrinth = new Labyrinth(10); Player player = new Player(9, 0); Dragon dragon = new Dragon(9, 9); JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon); frame.add(gui); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; //labyrinth, player, dragon are just public classes private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");…arrow_forward
- Make the following game user friendly with GUI, with some simple graphics. The GUI should be in another seperate class, with some ImageIcon, and Game class should be added into the pane. The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return…arrow_forwardDiscuss the negative and positive impacts or information technology in the context of your society. Provide two references along with with your answerarrow_forwardA cylinder of diameter 10 cm rotates concentrically inside another hollow cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poisearrow_forward
- Make the following game user friendly with GUI, with some simple graphics The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…arrow_forwardPlease original work What are four of the goals of information lifecycle management think they are most important to data warehousing, Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each. Please cite in text references and add weblinksarrow_forwardThe following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forward
- In this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forwardYou are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
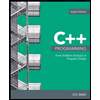
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
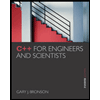
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
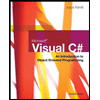
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
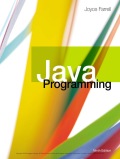
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT