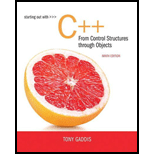
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 19, Problem 13PC
Program Plan Intro
Inventory Bin Queue
Program Plan:
InventoryItem.h:
- Include required header files
- Declare a class named “InventoryItem”. Inside the class,
- Inside “private” access specifier,
- Declare variables “serialNum”, “manufactDate”, and “lotNum”.
- Inside “public” access specifier,
- Give definition for default constructor.
- Give definition for overloaded constructor.
- Give mutator function “set_SerialNum ()” to set serial number.
- Give mutator function “set_ManufactDate ()” to set date.
- Give mutator function “set_LotNum ()” to set lot number.
- Give accessor function “get_SerialNum ()” that returns serial number.
- Give accessor function “get_ManufactDate ()” that returns date.
- Give accessor function “get_LotNum ()” that returns lot number.
- Inside “private” access specifier,
Dynqueue.h:
- Include required header files.
- Create template class
- Declare a class named “Dynqueue”. Inside the class,
- Inside the “private” access specifier,
- Create a structure named “QueueNode”.
- Create an object for the template
- Create a pointer named “next”.
- Create two pointers named “front” and “rear”.
- Create a structure named “QueueNode”.
- Inside “public” access specifier,
- Declare constructor and destructor.
- Declare the functions “enqueue ()”, “dequeue ()”, “isEmpty ()”, “isFull ()”, and “clear ()”.
- Inside the “private” access specifier,
- Declare template class.
- Give definition for the constructor.
- Assign the values.
- Declare template class.
- Give definition for the destructor.
- Call the function “clear ()”.
- Declare template class.
- Give function definition for “enqueue ()”.
- Make the pointer “newNode” as null.
- Assign “num” to newNode->value.
- Make newNode->next as null.
- Check whether the queue is empty using “isEmpty ()” function.
- If the condition is true then, assign newNode to “front” and “rear”.
- If the condition is not true then,
- Assign newNode to rear->next
- Assign newNode to “rear”.
- Increment the variable “numItems”.
- Declare template class.
- Give function definition for “dequeue ()”.
- Assign temp pointer as null.
- Check if the queue is empty using “isEmpty()” function.
- If the condition is true then print “The queue is empty”.
- If the condition is not true then,
- Assign the value of front to the variable “num”.
- Make front->next as “temp”.
- Delete the front value
- Make temp as front.
- Decrement the variable “numItems”.
- Declare template class.
- Give function definition for “isEmpty ()”.
- Assign “true” to a Boolean variable
- Check if “numItems” is true.
- If the condition is true then assign “false” to the variable.
- Return the Boolean variable.
- Declare template class.
- Give function definition for “clear ()”.
- Create an object for template.
- Dequeue values from queue till the queue becomes empty using “while” condition.
- Create an object for template.
Main.cpp:
- Include required header files
- Inside “main ()” function,
- Create a template for queue.
- Create an object for the class
- Declare variables “choice”, “serial”, and “mDate”.
- Print the menu to the user till the user enters corresponding menu number using “while” condition.
- Switch to case.
- Case1:
- Get the serial number and manufacturing date from the user.
- Push the object into the queue using the function “enqueue ()”.
- Case 2:
- Check if the queue is empty using “isEmpty ()” function.
- If the queue is not empty,
- Dequeue an element which is inserted first.
- Print the serial number and date that has been removed.
- Case 3:
- Exit
- Case1:
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Find the error, assume data is a string and all variables have been declared.
for ch in data:
if ch.isupper:
num_upper = num_upper + 1
if ch.islower:
num_lower = num_lower + 1
if ch.isdigit:
num_digits = num_digits + 1
if ch.isspace:
num_space = num_space + 1
Find the Error:
date_string = input('Enter a date in the format mm/dd/yyyy: ')
date_list = date_string.split('-')
month_num = int(date_list[0])
day = date_list[1]
year = date_list[2]
month_name = month_list[month_num - 1]
long_date = month_name + ' ' + day + ', ' + year
print(long_date)
Find the Error:
full_name = input ('Enter your full name: ')
name = split(full_name)
for string in name:
print(string[0].upper(), sep='', end='')
print('.', sep=' ', end='')
Chapter 19 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 19.1 - Describe what LIFO means.Ch. 19.1 - What is the difference between static and dynamic...Ch. 19.1 - What are the two primary stack operations?...Ch. 19.1 - What STL types does the STL stack container adapt?Ch. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - What is the difference between a static stack and...Ch. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - The STL stack is considered a container adapter....
Ch. 19 - What types may the STL stack be based on? By...Ch. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - The STL stack container is an adapter for the...Ch. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 21RQECh. 19 - Prob. 22RQECh. 19 - Prob. 23RQECh. 19 - Prob. 24RQECh. 19 - Prob. 25RQECh. 19 - Prob. 26RQECh. 19 - Write two different code segments that may be used...Ch. 19 - Prob. 28RQECh. 19 - Prob. 29RQECh. 19 - Prob. 30RQECh. 19 - Prob. 31RQECh. 19 - Prob. 32RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Dynamic String Stack Design a class that stores...Ch. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PCCh. 19 - Prob. 11PCCh. 19 - Inventory Bin Stack Design an inventory class that...Ch. 19 - Prob. 13PCCh. 19 - Prob. 14PCCh. 19 - Prob. 15PC
Knowledge Booster
Similar questions
- Please show the code for the Tikz figure of the complex plane and the curve C. Also, mark all singularities of the integrand.arrow_forward11. Go to the Webinars worksheet. DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: a. Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. b. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. c. Determine and enter the constraints based on the information provided in Table 3. d. Use Simplex LP as the solving method to find a global optimal solution. e. Save the Solver model below the Maximum weekly profit model label. f. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and…arrow_forwardGo to the Webinars DeShawn wants to determine the number of webinars the company can hold on Tuesdays and Thursdays to make the highest weekly profit without interfering with consultations, which are also scheduled for Tuesdays and Thursdays and use the same resources. Use Solver to find this information as follows: Use Total weekly profit as the objective cell in the Solver model, with the goal of determining the maximum value for that cell. Use the number of Tuesday and Thursday sessions for the five programs as the changing variable cells. Determine and enter the constraints based on the information provided in Table 3. Use Simplex LP as the solving method to find a global optimal solution. Save the Solver model below the Maximum weekly profit model label. Solve the model, keeping the Solver solution. Table 3: Solver Constraints Constraint Cell or Range Each webinar is scheduled at least once on Tuesday and once on Thursday B4:F5 Each Tuesday and Thursday…arrow_forward
- I want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)? When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene. Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency. To elaborate,…arrow_forwardI want to ask someone who has experiences in writing physics based simulation software. For context I am building a game engine, and want to implement physics simulation. There are a few approaches that I managed to find, but would like to know what are other approaches to doing physics simulation entry points from scenes, would you be able to visually draw me a few approaches (like 3 approaces)?When I say entry point to the actual physics simulation. An example of this is when the user presses the play button in the editor, it starts and initiates the physics system. Applying all of the global physics settings parameters that gets applied to that scene.Here is the use-case, I am looking for. If you have two scenes, and select scene 1. You press the play button. The physics simulation starts. When that physics simulation starts, you are also having to update the physics through some physics dedicated delta time because physics needs to happen faster update frequency.To elaborate, what…arrow_forwardMale comedians were typically the main/dominant star of television sitcoms made during the FCC licensing freeze. Question 19 options: True False In the episode of The Honeymooners that you watched this week, why did Alice decide to get a job outside of the home? Question 1 options: to earn enough money to buy a mink coat to have something to do while the kids were at school to pay the bills after her husband got laid offarrow_forward
- After the FCC licensing freeze was lifted, sitcoms featuring urban settings and working class characters became far less common. Question 14 options: True Falsearrow_forwardsolve this questions for me .arrow_forwarda) first player is the minimizing player. What move should be chosen?b) What nodes would not need to be examined using the alpha-beta pruning procedure?arrow_forward
- Consider the problem of finding a path in the grid shown below from the position S to theposition G. The agent can move on the grid horizontally and vertically, one square at atime (each step has a cost of one). No step may be made into a forbidden crossed area. Inthe case of ties, break it using up, left, right, and down.(a) Draw the search tree in a greedy search. Manhattan distance should be used as theheuristic function. That is, h(n) for any node n is the Manhattan distance from nto G. The Manhattan distance between two points is the distance in the x-directionplus the distance in the y-direction. It corresponds to the distance traveled along citystreets arranged in a grid. For example, the Manhattan distance between G and S is4. What is the path that is found by the greedy search?(b) Draw the search tree in an A∗search. Manhattan distance should be used as thearrow_forwardwhats for dinner? pleasearrow_forwardConsider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand. ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page) main()arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
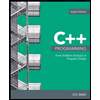
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
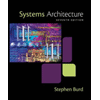
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
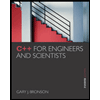
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
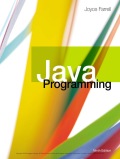
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT