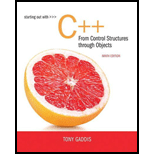
Starting Out with C++ from Control Structures to Objects (9th Edition)
9th Edition
ISBN: 9780134498379
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer
Chapter 19, Problem 32RQE
Explanation of Solution
STL Stack:
- STL stands for “Standard Template Library”.
- STL offers a stack template which can be implemented as a list, a deque or a
vector . - By default, STL stack is based on “deque” type.
- This is called as a container adapter because; the stack container is used to adapt these containers.
STL stack container adapts:
- List type:
- Example declaration: stack <int, vector<int>> iStack;
- Vector type:
- Example declaration: stack <int, list<int>> iStack;
- Deque type:
- ...
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
[5 marks] Give a recursive definition for the language anb2n where n = 1, 2, 3, ... over the alphabet Ó={a, b}.
2) [12 marks] Consider the following languages over the alphabet ={a ,b},
(i) The language of all words that begin and end an a
(ii) The language where every a in a word is immediately followed by at least one b.
(a) Express each as a Regular Expression
(b) Draw an FA for each language
(c) For Language (i), draw a TG using at most 3 states
(d) For Language (ii), construct a CFG.
Question 1 Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule. Question 2 Construct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.
Generate a random sample of standard lognormal data (rlnorm()) for sample size n = 100. Construct histogram estimates of density for this sample using Sturges’ Rule, Scott’s Normal Reference Rule, and the FD Rule.
Chapter 19 Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Ch. 19.1 - Describe what LIFO means.Ch. 19.1 - What is the difference between static and dynamic...Ch. 19.1 - What are the two primary stack operations?...Ch. 19.1 - What STL types does the STL stack container adapt?Ch. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - What is the difference between a static stack and...Ch. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - The STL stack is considered a container adapter....
Ch. 19 - What types may the STL stack be based on? By...Ch. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - The STL stack container is an adapter for the...Ch. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 21RQECh. 19 - Prob. 22RQECh. 19 - Prob. 23RQECh. 19 - Prob. 24RQECh. 19 - Prob. 25RQECh. 19 - Prob. 26RQECh. 19 - Write two different code segments that may be used...Ch. 19 - Prob. 28RQECh. 19 - Prob. 29RQECh. 19 - Prob. 30RQECh. 19 - Prob. 31RQECh. 19 - Prob. 32RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Dynamic String Stack Design a class that stores...Ch. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PCCh. 19 - Prob. 11PCCh. 19 - Inventory Bin Stack Design an inventory class that...Ch. 19 - Prob. 13PCCh. 19 - Prob. 14PCCh. 19 - Prob. 15PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Can I get help with this case please, thank youarrow_forwardI need help to solve the following, thank youarrow_forwardreminder it an exercice not a grading work GETTING STARTED Open the file SC_EX19_EOM2-1_FirstLastNamexlsx, available for download from the SAM website. Save the file as SC_EX19_EOM2-1_FirstLastNamexlsx by changing the “1” to a “2”. If you do not see the .xlsx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically. With the file SC_EX19_EOM2-1_FirstLastNamexlsx still open, ensure that your first and last name is displayed in cell B6 of the Documentation sheet. If cell B6 does not display your name, delete the file and download a new copy from the SAM website. Brad Kauffman is the senior director of projects for Rivera Engineering in Miami, Florida. The company performs engineering projects for public utilities and energy companies. Brad has started to create an Excel workbook to track estimated and actual hours and billing amounts for each project. He asks you to format the workbook to make the…arrow_forward
- Need help with coding in this in python!arrow_forwardIn the diagram, there is a green arrow pointing from Input C (complete data) to Transformer Encoder S_B, which I don’t understand. The teacher model is trained on full data, but S_B should instead receive missing data—this arrow should not point there. Please verify and recreate the diagram to fix this issue. Additionally, the newly created diagram should meet the same clarity standards as the second diagram (Proposed MSCATN). Finally provide the output image of the diagram in image format .arrow_forwardPlease provide me with the output image of both of them . below are the diagrams code make sure to update the code and mentionned clearly each section also the digram should be clearly describe like in the attached image. please do not provide the same answer like in other question . I repost this question because it does not satisfy the requirment I need in terms of clarifty the output of both code are not very well details I have two diagram : first diagram code graph LR subgraph Teacher Model (Pretrained) Input_Teacher[Input C (Complete Data)] --> Teacher_Encoder[Transformer Encoder T] Teacher_Encoder --> Teacher_Prediction[Teacher Prediction y_T] Teacher_Encoder --> Teacher_Features[Internal Features F_T] end subgraph Student_A_Model[Student Model A (Handles Missing Values)] Input_Student_A[Input M (Data with Missing Values)] --> Student_A_Encoder[Transformer Encoder E_A] Student_A_Encoder --> Student_A_Prediction[Student A Prediction y_A] Student_A_Encoder…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
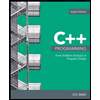
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
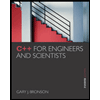
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
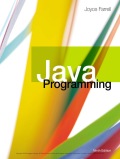
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
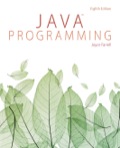
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
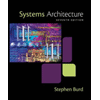
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning