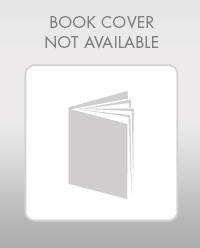
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 19, Problem 13RQE
Program Plan Intro
Binary tree:
- Binary tree is a non-linear data structure.
- Binary tree contains node such as root node that is pointed to two child nodes.
- A root node will have left reference node and right reference node.
- Binary tree will contain more than one self-referenced field.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
In Python
Given a singly linked list, print reverse of it using a recursive function
printLinkedList( node *first ) where first is the pointer pointing to the first data
node. For example, if the given linked list is 1->2->3->4, then output should be:
4 3 2 1
(note the whitespace in between each data value)
Create a function that takes an array that represent a
Binary Tree and a value and return true if the value is in
the tree and, false otherwise.
Examples
valueInTree (arr1, 5) true
valueInTree (arr1, 9) → false
valueInTree (arr2, 51) → false
Chapter 19 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 19.1 - Prob. 19.1CPCh. 19.1 - Prob. 19.2CPCh. 19.1 - Prob. 19.3CPCh. 19.1 - Prob. 19.4CPCh. 19.1 - Prob. 19.5CPCh. 19.1 - Prob. 19.6CPCh. 19.2 - Prob. 19.7CPCh. 19.2 - Prob. 19.8CPCh. 19.2 - Prob. 19.9CPCh. 19.2 - Prob. 19.10CP
Ch. 19.2 - Prob. 19.11CPCh. 19.2 - Prob. 19.12CPCh. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - Prob. 3RQECh. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - Prob. 6RQECh. 19 - Prob. 7RQECh. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - Prob. 17RQECh. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Prob. 6PCCh. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PC
Knowledge Booster
Similar questions
- Write a function called PtrToSuccessor that finds a node with the smallest key value in a tree, unlinks it from the tree, and returns a pointer to the unlinked node. **Please Do Not Use Any Pre-Implemented Code, And It Is Not A Binary Search Tree*l **I NEED C++ CODE, NOT C CODE** Thank you.arrow_forwardCreate a function that takes an array that represent a Binary Tree and a value and return true if the value is in the tree and, false otherwise. Examples valueInTree (arr1, 5) valueInTree (arr1, 9) valueInTree (arr2, 51) - true 1 false falsearrow_forwardI have an assignment that requires me to read strings from a text file and insert them into a binary search tree. I am having a hard time reading the data from the file and passing it through a function. This is the code I have right now: struct tree_node {char *data;tree_node *left;tree_node *right;}; char **Read();tree_node *create_node(char **val); int main(){tree_node *root= NULL, *temp_node;char **val = (char**)malloc(sizeof(char*)*17);for (int i = 0; i < 17; i++) {val[i] = (char*)malloc(sizeof(char)*strlen(*val));}temp_node = create_node(val);root = insert(root, temp_node);printf("In Order: ");inorder(root);printf("\n");return 0;} char **Read() {int size;FILE *fp = fopen("in.txt", "r");fscanf(fp, "%d", &size);char** value = (char**)malloc(sizeof(char*));for(int i = 0; i < size; i++) {fscanf(fp, "%s", &value[i]);} fclose(fp);return value;} tree_node *create_node(char **val) {tree_node* temp = (tree_node*)malloc(sizeof(tree_node));for(int i = 0; i < 17; i++)for(int…arrow_forward
- Given a reference to a binary tree t. I am trying to write a python function using recursion that will check if an integer called k is stored in a leaf node in the tree. if not stored it should return false but if it is I want it to return True. starting function: def in_leaf(t,k):arrow_forwardWrite a C++ program to implement binary search tree (BST). Your program should accept a list ofnumbers from the user (minimum 5) and then build a binary search tree from those numbers.Implement following functions: Serach function: Number is found or not leavesCount: Returns number of leaves in binary tree inorder: Print the contents of tree using inorder traversal Note: Define three files: bst.h, bst.cpp, main.cpp to solve this problemarrow_forwardThe level of a node in the tree is the number of nodes in the path from the root to the node (including itself). For example, the node 15 in the example tree above has a level of 2. Write a function prune-tree that will take a tree representation as well as an integer level. It will return a new tree containing only nodes that have level not exceeding level. The order of the nodes in the result list should be the same as the original order in the tree. You may assume that level is a non-negative integer. write the program in racketarrow_forward
- For a singly linked list pointed at by pointer Ptr, write the implementation of a function named CountPositive that counts and prints the nodes with positive value in the list. Take in consideration all special cases. (** The declaration of the Node is: typedef struct Node { int data; Node*next; }Node;arrow_forwardpython: In a binary search tree, write another way of function that takes in a root, p, and checks whether the tree rooted in p is a binary search tree or not. What is the time complexity of your function? def is_bst(self, p): root=p def helper(root, l, r): if not root: return True if not (left<root.val and root.val<right): return False return helper(root.l, l, root.val) and helper(root.r, root.val, r) return helper(root, float('-inf'), float('inf'))arrow_forwardWrite a C++ class called BSTArray with five basic functions for the BST: insert, search, findmin, findmax, and print: 1. int search(x): Find and return the index that stores element x using binary search tree mechanism. Print out all the elements in the search path. You must use the binary tree search algorithm. In other words, do NOT just do a linear search of the array. If the x value is not found, report an error and return -1. 2. int findmax( ): Find and return maximum value in BST. You must use the binary tree search algorithm. In other words, do NOT just perform a linear search of the array. If the tree is empty, return -1. 3. int findmin( ): Find and return minimum value in BST. In other words, do NOT just perform a linear search of the array. If the tree is empty, return -1. 4. void print( ): Print out the BST structure in the form of an array with index. Specifically, print the index of the array and the value stored at that index starting at zero and ending at the capacity of…arrow_forward
- Write a function that will print all of the elements in a tree in postprder recursively.arrow_forwardImplement a circular singly linked list in C programming language. Create functions for the ff: 1. Transversal 2. Insertion of element (at the beginning, in between nodes, and at the end) 3. Deletion of element 4. Search 5. Sortarrow_forwardwrite a function in python that recieves the reference to the root (t) of a binary search tree and returns a listcontaining the keys in the path from the root of (t) to the largest key in (t). The function must use recursion Starting code: def path_to_largest(t): return [t.key]arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
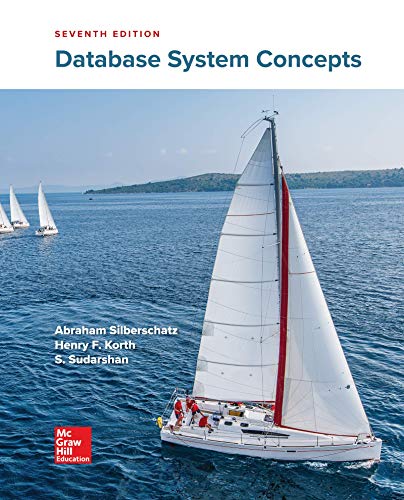
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
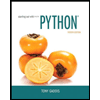
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
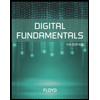
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
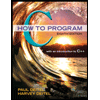
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
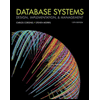
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
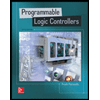
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education