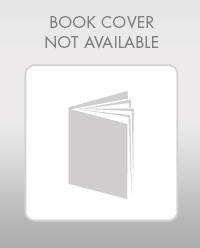
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 5PC
Program Plan Intro
Tree Width
Program Plan:
- Define a class BTreeNode to create nodes for binary search tree items.
- Include all the required header files.
- Initialize a value to the node, and set the left child of node to leftp and right child of node to rightp .
- Define a class to create a Binary Search Tree.
- Create a function int width that returns width of the tree.
- Create a function bool search to search a particular item in tree.
- Create a function leafCounter that counts no. of leaves present in a level.
- Create function void insert to insert nodes into the tree.
- Create a function void inorder to sort items in inorder traversal.
- Declare the main function.
- Prompt the user to enter 5 numbers to be inserted into tree.
- Sort the items present in the tree in inorder traversal and return the width of the Binary Search Tree.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
using r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.
using r language
using r language
Chapter 19 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 19.1 - Prob. 19.1CPCh. 19.1 - Prob. 19.2CPCh. 19.1 - Prob. 19.3CPCh. 19.1 - Prob. 19.4CPCh. 19.1 - Prob. 19.5CPCh. 19.1 - Prob. 19.6CPCh. 19.2 - Prob. 19.7CPCh. 19.2 - Prob. 19.8CPCh. 19.2 - Prob. 19.9CPCh. 19.2 - Prob. 19.10CP
Ch. 19.2 - Prob. 19.11CPCh. 19.2 - Prob. 19.12CPCh. 19 - Prob. 1RQECh. 19 - Prob. 2RQECh. 19 - Prob. 3RQECh. 19 - Prob. 4RQECh. 19 - Prob. 5RQECh. 19 - Prob. 6RQECh. 19 - Prob. 7RQECh. 19 - Prob. 8RQECh. 19 - Prob. 9RQECh. 19 - Prob. 10RQECh. 19 - Prob. 11RQECh. 19 - Prob. 12RQECh. 19 - Prob. 13RQECh. 19 - Prob. 14RQECh. 19 - Prob. 15RQECh. 19 - Prob. 16RQECh. 19 - Prob. 17RQECh. 19 - Prob. 18RQECh. 19 - Prob. 19RQECh. 19 - Prob. 20RQECh. 19 - Prob. 1PCCh. 19 - Prob. 2PCCh. 19 - Prob. 3PCCh. 19 - Prob. 4PCCh. 19 - Prob. 5PCCh. 19 - Prob. 6PCCh. 19 - Prob. 7PCCh. 19 - Prob. 8PCCh. 19 - Prob. 9PCCh. 19 - Prob. 10PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- using r language Obtain a bootstrap t confidence interval estimate for the correlation statistic in Example 8.2 (law data in bootstrap).arrow_forwardusing r language Compute a jackknife estimate of the bias and the standard error of the correlation statistic in Example 8.2.arrow_forwardusing r languagearrow_forward
- using r languagearrow_forwardThe assignment here is to write an app using a database named CIT321 with a collection named students; we will provide a CSV file of the data. You need to use Vue.js to display 2 pages. You should know that this assignment is similar, all too similar in fact, to the cars4sale2 example in the lecture notes for Vue.js 2. You should study that program first. If you figure out cars4sale2, then program 6 will be extremely straightforward. It is not my intent do drop a ton of new material here in the last few days of class. The database contains 51 documents. The first rows of the CSV file look like this: sid last_name 1 Astaire first_name Humphrey CIT major hrs_attempted gpa_points 10 34 2 Bacall Katharine EET 40 128 3 Bergman Bette EET 42 97 4 Bogart Cary CIT 11 33 5 Brando James WEB 59 183 6 Cagney Marlon CIT 13 40 GPA is calculated as gpa_points divided by hrs_attempted. GPA points would have been arrived at by adding 4 points for each credit hour of A, 3 points for each credit hour of…arrow_forwardI need help to solve the following case, thank youarrow_forward
- You will write a program that allows the user to keep track of college locations and details about each location. To begin you will create a College python class that keeps track of the csollege's unique id number, name, address, phone number, maximum students, and average tuition cost. Once you have built the College class, you will write a program that stores College objects in a dictionary while using the College's unique id number as the key. The program should display a menu in this order that lets the user: 1) Add a new College 2) Look up a College 4) Delete an existing College 5) Change an existing College's name, address, phone number, maximum guests, and average tuition cost. 6) Exit the programarrow_forwardShow all the workarrow_forwardConstruct a frequency polygon density estimate for the sample in Question 1, using bin width determined by Sturges’ Rule.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
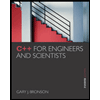
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
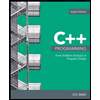
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
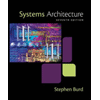
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
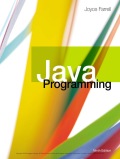
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
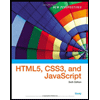
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning