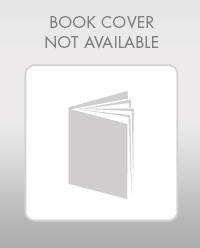
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 2PC
Program Plan Intro
Dynamic Stack Template
Program Plan:
Main.cpp:
- Include required header files.
- Inside the “main ()” function,
- Create an object named “dstack” for stack.
- Declare a variable named “popElem”.
- Push 3 elements inside the stack using the function “push ()”.
- Pop 3 elements from the stack using the function “pop ()”.
DynStack.h:
- Include required header files.
- Create a template.
- Declare a class named “DynStack”. Inside the class
- Inside the “private” access specifier,
- Give structure declaration for the stack
- Create an object for the template
- Create a stack pointer name “next”.
- Create a stack pointer name “top”
- Give structure declaration for the stack
- Inside the “public” access specifier,
- Give a declaration for a constructor.
- Assign null to the top node.
- Give function declaration for “push ()”, “pop ()”,and “isEmpty ()”.
- Give a declaration for a constructor.
- Inside the “private” access specifier,
- Give the class template.
- Give function definition for “push ()”.
- Assign null to the new node.
- Dynamically allocate memory for new node
- Assign “num” to the value of new node.
- Check if the stack is empty using the function “isEmpty ()”
- If the condition is true then assign new node as the top and make the next node as null.
- If the condition is not true then, assign top node to the next of new node and assign new node as the top.
- Give the class template.
- Give function definition for “pop ()”.
- Assign null to the temp node.
- Check if the stack is empty using the function “is_Empty ()”
- If the condition is true then print “The stack is empty”.
- If the condition is not true then,
- Assign top value to the variable “num”.
- Link top of next node to temp node.
- Delete the top most node and make temp as the top node.
- Give function definition for “isEmpty ()”.
- Assign Boolean value to the variable
- Check if the top node is null
- Assign true to “status”.
- Return the status
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Describe three (3) Multiplexing techniques common for fiber optic links
Could you help me to know features of the following concepts:
- commercial CA
- memory integrity
- WMI filter
Briefly describe the issues involved in using ATM technology in Local Area Networks
Chapter 18 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - The STL stack is considered a container adapter....Ch. 18 - What types may the STL stack be based on? By...
Ch. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 14PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
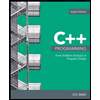
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
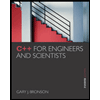
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
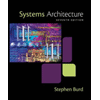
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
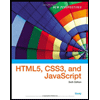
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
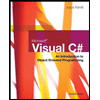
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,