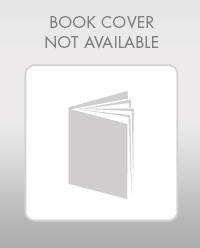
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 8PC
Program Plan Intro
Stack Copy Operations
Program Plan:
- Declare the main function.
- Prompt the user to enter a postfix expression.
- Convert an input stream into a string stream.
- Evaluate the postfix expression by calling the postfixExpr function and print the result.
- Skip whitespace in an input stream while evaluating a postfix expression.
- Declare a Function postfixExpr that evaluates the postfix expression by considering if the next token in the input stream is an integer, read the integer and push it onto the stack using the push() operation of the stack .
- However, if the input stream is an operator, pop the last two values from the stack using the pop operation and apply the operator, and push the result onto the stack and the lone value is the result and look for next iteration.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Stack:
Stacks are a type of container with LIFO (Last In First Out) type of working, where a new element is added at one end and (top) an element is removed from that end only. Your Stack should not be of the fixed sized. It should be able to grow itself. So using the class made in task 1, make a class named as Stack, having following additional functionalities:
bool empty() : Returns whether the Stack is empty or not. Time Complexity should be: O(1)
bool full() : Returns whether the Stack is full or not. Time Complexity should be: O(1)int size() : Returns the current size of the Stack. Time Complexity should be: O(1)Type top () : Returns the last element of the Stack. Time Complexity should be: O(1)
void push(Type) : Adds the element of type Type at the top of the stack. Time Complexity should be: O(1)
Type pop() : Deletes the top most element of the stack and returns it. Time Complexity should be: O(1)
Write non-parameterized constructor for the above class.
Write Copy…
C#
Reverse the stack - This procedure will reverse the order of items in the stack. This one may NOT break the rules of the stack. HINTS: Make use of more stacks. Arrays passed as parameters are NOT copies. Remember, this is a procedure, not a function.
This would occur in the NumberStack class, not the main class. These are the provided variables:
private int [] stack;private int size;
Create a method that will reverse the stack when put into the main class.
1. Stack Implementation
Write a method called insert for the class Stack. The method shall be
implemented using an array-based structure. You can assume that String[]
stack is defined in the class, which is responsible to store the elements and
responsible to enforce the order of first-in last-out, a.k.a., FIFO. Additionally, you
can assume there is a pointer called top, that indicates the position of the top of
the stack, pointing to the next available position to insert.
The method shall:
• take a String s as a parameter, and shall add it at the top of the stack.
shall return true if the element s was added successfully at the top of the
stack, false otherwise.
.
. The method must check boundaries of capacity and limitation of the Stack. In
case the method is invoked to insert an element of the top of the stack that
exceeds its current capacity, the method shall handle the situation properly.
Do not provide the entire Stack implementation, only the code solution of the
method.
Chapter 18 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - The STL stack is considered a container adapter....Ch. 18 - What types may the STL stack be based on? By...
Ch. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 14PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- C++ ProgrammingActivity: Linked List Stack and BracketsExplain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow #include "stack.h" #include "linkedlist.h" // SLLStack means Singly Linked List (SLL) Stack class SLLStack : public Stack { LinkedList* list; public: SLLStack() { list = new LinkedList(); } void push(char e) { list->add(e); return; } char pop() { char elem; elem = list->removeTail(); return elem; } char top() { char elem; elem = list->get(size()); return elem; } int size() { return list->size(); } bool isEmpty() { return list->isEmpty(); } };arrow_forwarddata structure-JAVAarrow_forwardReference-based Linked Lists: Select all of the following statements that are true. As a singly linked list's node references both its predecessor and its successor, it is easily possible to traverse such a list in both directions. According to the terminology introduced in class, the head reference variable in a singly linked list object references the list's first node. According to the terminology introduced in class, in a doubly linked list, each node references both the head and tail node. In a double-ended singly linked list, the tail reference variable provides access to the entire list. In a circular linked list, the last node references the first node.arrow_forward
- Object Oriented Programing: Create a class template for a class named GeneralStackthat holds • A single data member as an array named stack of size 50 to store certain elements • Three member functions i.e. push(type) to add elements in the Stack, pop() to remove elements from the stack, and currentStatus() to check whether the array is filled or not. (A filled array is an array that has non-zero value at all of its indexes). In the main() function, create three objects with different data types of class General Stack and test the functionality of member functions for various values of data members for these objects.arrow_forwardComputer Science //iterator() creates a new Iterator over this list. It will//initially be referring to the first value in the list, unless the//list is empty, in which case it will be considered both "past start"//and "past end". template <typename ValueType>typename DoublyLinkedList<ValueType>::Iterator DoublyLinkedList<ValueType>::iterator(){//return iterator(head);} //constIterator() creates a new ConstIterator over this list. It will//initially be referring to the first value in the list, unless the//list is empty, in which case it will be considered both "past start"//and "past end". template <typename ValueType>typename DoublyLinkedList<ValueType>::ConstIterator DoublyLinkedList<ValueType>::constIterator() const{//return constIterator(head);} //Initializes a newly-constructed IteratorBase to operate on//the given list. It will initially be referring to the first//value in the list, unless the list is empty, in which case//it will be…arrow_forwardIn c++ Also add comments explaining each linearrow_forward
- Assume the function: void F(stack<T> &S){ } and we send a stack S to the function F, as a result of it Select one: a. Both (copy constructor and destructor) should not be called b. Destructor should be called c. Copy constructor should be called d. Both (copy constructor and destructor) should be called Clear my choicearrow_forwardAssume the function: void F(stack<T> &S){ } and we send a stack S to the function F, as a result of it إختر أحد الخيارات: a. Both (copy constructor and destructor) should be called b. Both (copy constructor and destructor) should not be called c. Destructor should be called d. Copy constructor should be calledarrow_forwardFor C++ please just need cpp filearrow_forward
- X1222: Double Ended Queue: Deque A double ended queue, known as deque, is a queue data structure that allows adding and removing elements from both ends of the queue. Instead of enqueue and dequeue, it has insert, delete, and get for both front and last of the queue as shown below. The data stored internally is stored in a ListNodesPlus object. The basic class definition is shown below: public class Deque { private ListNodePlus elements; // code ommitted for space public void clear() {...}; public int numElements () {...}; public boolean isEmpty() {...}; ● // Implement the following four methods public void insertFront (E it) { } public E deleteFront () { } public void insertLast (E it) { } public E deleteLast() { } Write the following four methods: • insertFront (E it) takes it and adds it to the front of the queue. stored internally in elements. The front of the queue is defined as position 0 in the queue. ● deleteFront () removes the element at the front of the queue and returns it.…arrow_forwardURGENT URGENT URGENT !!!! Write a void method swapStackwithQueue that takes MyStack and MyQueue Objects as parameters and exchanges the elements. a) The top element of the old stack becomes the rear element of the new queue. b b) and the rear element of the old queue becomes the top element of the new stack. The output should be similar to the image.arrow_forwardComputer science JAVA programming languagearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
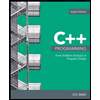
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning