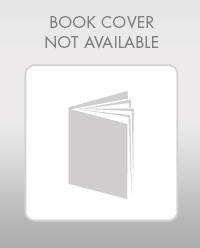
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 18, Problem 5PC
Program Plan Intro
Error Testing
Dynamic Stack:
Program Plan:
Main.cpp:
- Include required header files.
- Inside the “main ()” function,
- Create an object named “dstack” for stack.
- Declare a variable named “popElem”.
- Push 3 elements inside the stack using the function “push ()”.
- Pop 3 elements from the stack using the function “pop ()”.
DynIntStack.h:
- Include required header files.
- Create a template.
- Declare a class named “DynIntStack”. Inside the class
- Inside the “private” access specifier,
- Give structure declaration for the stack.
- Create an object for the template.
- Create a stack pointer name “next”.
- Create a stack pointer name “top”.
- Give structure declaration for the stack.
- Inside the “public” access specifier,
- Give a declaration for a constructor.
- Assign null to the top node.
- Give function declaration for “push ()”, “pop ()”,and “isEmpty()”.
- Give a declaration for a constructor.
- Inside the “private” access specifier,
- Give the class template.
- Define function definition for “push ()”.
- Assign null to the new node.
- Dynamically allocate memory for new node,
- Assign “num” to the value of new node.
- Check if the stack is empty using the function “isEmpty ()”
- If the condition is true then assign new node as the top and make the next node as null.
- If the condition is not true then, assign top node to the next of new node and assign new node as the top.
- Give the class template.
- Define function definition for “pop ()”.
- Assign null to the temp node.
- Check if the stack is empty using the function “is_Empty ()”
- If the condition is true then print “The stack is empty”.
- If the condition is not true then,
- Assign top value to the variable “num”.
- Link top of next node to temp node.
- Delete the top most node and make temp as the top node.
- Define function definition for “isEmpty ()”.
- Assign Boolean value to the variable.
- Check if the top node is null.
- Assign true to “status”.
- Return the status.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
CLASSES, DYNAMIC ARRAYS AND POINTERS
Define a class called textLines that will be used to store a list of lines of text (each line can be specified as a string).
Use a dynamic array to store the list. In addition, you should have a private data member that specifies the length of the list.
Create a constructor that takes a file name as parameter, and fills up the list with lines from the file. Make sure that you set the dynamic array to expand large enough to hold all the lines from the file. Also, create a constructor that takes an integer parameter that sets the size of an empty list.
in C++
Write member functions to:
remove and return the last line from the list
add a new line onto the end of the list, if there is room for it, otherwise print a message and expand the array
empty the entire list
return the number of lines still on the list
take two lists and return one combined list (with no duplicates)
copy constructor to support deep copying
remember the destructor!
C++ Programming
Requirments:
Please submit just one file for the classes and main to test the classes. Just create the classes before main and submit only one cpp file. Do not create separate header files.
Please note: the deleteNode needs to initialize the *nodePtr and the *previousNode. The code to do this can be copied from here:ListNode *nodePtr, *previousNode = nullptr;
Part 1: Your own Linked ListDesign your own linked list class to hold a series of integers. The class should have member functions for appending, inserting, and deleting nodes. Don't forget to add a destructor that destroys the list. Demonstrate the class with a driver program.Part 2: List PrintModify the linked list class you created in part 1 to add a print member function. The function should display all the values in the linked list. Test the class by starting with an empty list, adding some elements, and then printing the resulting list out.
c++
Chapter 18 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 18.3 - Describe what LIFO means.Ch. 18.3 - What is the difference between static and dynamic...Ch. 18.3 - What are the two primary stack operations?...Ch. 18.3 - What STL types does the STL stack container adapt?Ch. 18 - Prob. 1RQECh. 18 - Prob. 2RQECh. 18 - What is the difference between a static stack and...Ch. 18 - Prob. 4RQECh. 18 - The STL stack is considered a container adapter....Ch. 18 - What types may the STL stack be based on? By...
Ch. 18 - Prob. 7RQECh. 18 - Prob. 8RQECh. 18 - Prob. 9RQECh. 18 - Prob. 10RQECh. 18 - Prob. 11RQECh. 18 - Prob. 12RQECh. 18 - Prob. 13RQECh. 18 - Prob. 14RQECh. 18 - Prob. 15RQECh. 18 - Prob. 16RQECh. 18 - Prob. 17RQECh. 18 - Prob. 18RQECh. 18 - Prob. 1PCCh. 18 - Prob. 2PCCh. 18 - Prob. 3PCCh. 18 - Prob. 4PCCh. 18 - Prob. 5PCCh. 18 - Prob. 6PCCh. 18 - Prob. 7PCCh. 18 - Prob. 8PCCh. 18 - Prob. 14PCCh. 18 - Prob. 9PCCh. 18 - Prob. 10PCCh. 18 - Prob. 11PCCh. 18 - Prob. 12PCCh. 18 - Prob. 13PCCh. 18 - Prob. 15PC
Knowledge Booster
Similar questions
- C# Programing Language Given the code below 1.Change all of the low-level arrays in your program to use the appropriate C# collection class instead. Be sure to implement Deck as a Stack 2. Make sure that you use the correct type-safe generic designations throughout your program. 3.Write code that watches for that incorrect data and throws an appropriate exception if bad data is found.4. Write Properties instead of Getter and Setter methods. no lamdas, no delegates, no LINQ, no eventsGo Fish using System; namespace GoFish{public enum Suit { Clubs, Diamonds, Hearts, Spades };public enum Rank { Ace, Two, Three, Four, Five, Six, Seven, Eight, Nine, Ten, Jack, Queen, King }; // ----------------------------------------------------------------------------------------------------- public class Card{public Rank Rank { get; private set; }public Suit Suit { get; private set; } public Card(Suit suit, Rank rank){this.Suit = suit;this.Rank = rank;} public override string ToString(){return ("[" +…arrow_forwardIn C++ Pleasearrow_forwardCourse: Data Structure and Algorithims Language: Java Kindly make the program in 2 hours. Task is well explained. You have to make the proogram properly in Java: Restriction: Prototype cannot be change you have to make program by using given prototype. TAsk: Create a class Node having two data members int data; Node next; Write the parametrized constructor of the class Node which contain one parameter int value assign this value to data and assign next to null Create class LinkList having one data members of type Node. Node head Write the following function in the LinkList class publicvoidinsertAtLast(int data);//this function add node at the end of the list publicvoid insertAthead(int data);//this function add node at the head of the list publicvoid deleteNode(int key);//this function find a node containing "key" and delete it publicvoid printLinkList();//this function print all the values in the Linklist public LinkListmergeList(LinkList l1,LinkList l2);// this function…arrow_forward
- CPU Scheduling Algorithm Simulator in C++Instructions:Create a .zip file consisting of code, output files, readme file .The readme file should explain briefly your code. The simulator will consists of following classes:(i) Process: The data members of this class should store process id, arrival time in the ready queue,CPU burst time, completion time, turn around time, waiting time, and response time. The memberfunctions of this class should assign values to the data members and print them. A constructorshould also be used.(ii) Process_Creator: This class will create an array of processes and assign a random arrival timeand burst time to each process. Data members, constructor and member functions can be writtenaccordingly.(iii) Scheduler: This class will implement the scheduling algorithm. The class will maintain a readyqueue of infinite capacity (i.e., any number of processes can be accommodated in the queue). Theready queue should be implemented using the min-Heap data structure…arrow_forwardFor C++ please just need cpp filearrow_forwardX1222: Double Ended Queue: Deque A double ended queue, known as deque, is a queue data structure that allows adding and removing elements from both ends of the queue. Instead of enqueue and dequeue, it has insert, delete, and get for both front and last of the queue as shown below. The data stored internally is stored in a ListNodesPlus object. The basic class definition is shown below: public class Deque { private ListNodePlus elements; // code ommitted for space public void clear() {...}; public int numElements () {...}; public boolean isEmpty() {...}; ● // Implement the following four methods public void insertFront (E it) { } public E deleteFront () { } public void insertLast (E it) { } public E deleteLast() { } Write the following four methods: • insertFront (E it) takes it and adds it to the front of the queue. stored internally in elements. The front of the queue is defined as position 0 in the queue. ● deleteFront () removes the element at the front of the queue and returns it.…arrow_forward
- struct nodeType { int infoData; nodeType * next; }; nodeType *first; … and containing the values(see image) Using a loop to reach the end of the list, write a code segment that deletes all the nodes in the list. Ensure the code performs all memory ‘cleanup’ functions.arrow_forwardcode: public class PasswordGeneratorAndStorage {/*** Adds an application to the applications ArrayList. If index is -1, add application* to the end of applications. Else is index is within the size of applications, add* application at that index in applications. Otherwise, return. However, if applications * or application is null, return.* * @param applications* @param application* @param index*/public static void addApplication(ArrayList<String> applications, String application, int index) {// TODO: FILL IN BODY}/*** Generates a random password of length passwordLength and adds it to the end of* passwords if index is -1, or adds it to the index of passwords if index is within the* size of passwords (similar to how the addApplication adds an application). To generate* the random password, use rand to generate a random int within the size of characters. You* can then use this int to grab the char at that index in characters and concatenate that* to your String password variable.…arrow_forwardJAVA programming language DescriptionYour job is to write your own array list (growable array) that stores elements that are objects of a parentclass or any of its child classes but does not allow any other type of objects to be stored. You will createa general data class and two specific data classes that inherit from that class. You will then write thearray list to hold any objects of the general data class. Finally, you will test your array list using JUnittests to prove that it does what it should.DetailsYour array list must be named DataList. Start with an array with room for 10 elements. Keep all elementscontiguous (no blank places) at the low end of the indices. When the array is full, grow it by doubling thesize. (Do not use any built-in methods to copy the array elements. Write your own code to do this.) Youdo not have to shrink the array when elements are removed. The array list must have the followingpublic methods (listed in UML style where GenClass is your general data…arrow_forward
- Smart Pointer: Write a smart pointer class. A smart pointer is a data type, usually implemented with templates, that simulates a pointer while also providing automatic garbage collection. It automatically counts the number of references to a SmartPointer<?> object and frees the object of type T when the reference count hits zero.arrow_forwardUse the template below: def createList(n): #Base Case/s #ToDo: Add conditions here for base case/s #if <condition> : #return <value> #Recursive Case/s #ToDo: Add conditions here for your recursive case/s #else: #return <operation and recursive call> #remove the line after this once all ToDo is completed return [] def removeMultiples(x, arr): #Base Case/s #TODO: Add conditions here for your base case/s #if <condition> : #return <value> #Recursive Case/s #TODO: Add conditions here for your recursive case/s #else: #return <operation and recursive call> #remove the line after this once you've completed all ToDo return [] def Sieve_of_Eratosthenes(list): #Base Case/s if len(list) < 1 : return list #Recursive Case/s else: return [list[0]] + Sieve_of_Eratosthenes(removeMultiples(list[0], list[1:])) if __name__ == "__main__": n = int(input("Enter n: "))…arrow_forwardC++ The People class holds an unsorted list of humans. Initially, this list is empty. Upon inserting a Human object, it is added to the array at the next available position and the position value in incremented. If the position value is equal to the size value, do not insert the Human. Instead, throw an exception that your driver can catch to print an error that the People object is full. Create a People class with the following features: Each People object has a statically allocated array of Human objects. Size attribute representing the maximum size of the array. Position attribute representing the next free position in the array. Default constructor with no arguments. Search method that takes a string argument and returns a Human object. This method returns the first Human object with a name matching the string argument. In other words, pass the name of a Human as a string, search the list of humans for one with that name. If found, return this human. Else throw an…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
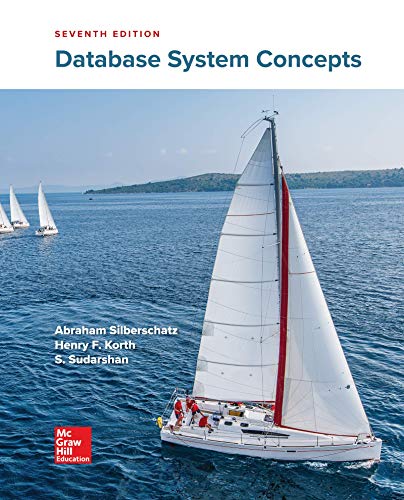
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
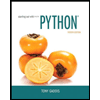
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
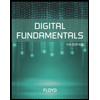
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
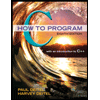
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
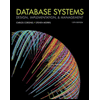
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
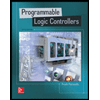
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education