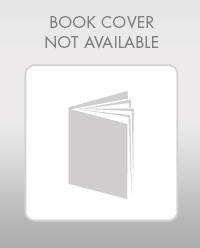
Concept explainers
What will the following
#include <iostream>
#include <memory>
using namespace std;
class First
{
protected:
int a;
public:
First(int x = 1) { a = x; }
int getVal() const { return a; }
};
class Second : public First
{
private:
int b;
public:
Second(int y = 5) { b = y; }
int getVal() const { return b; }
};
int main()
{
Shared_ptr<First> object1 = make_shared<First>();
shared_ptr<Second> object2 = make_shared<Second>():
cout << object1->getVal() << endl;
cout << object2−>getVal() << endl:
return 0;
}

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Starting Out With C++: Early Objects (10th Edition)
Additional Engineering Textbook Solutions
Modern Database Management (12th Edition)
Starting Out with Programming Logic and Design (4th Edition)
Software Engineering (10th Edition)
Starting out with Visual C# (4th Edition)
Java: An Introduction to Problem Solving and Programming (7th Edition)
Web Development and Design Foundations with HTML5 (9th Edition) (What's New in Computer Science)
- Class Implementation Exercises EX1: Write a complete C++ program to implement a car class. The class has three attributes: Id, speed and color. Also, the class include the following methods: (setter) function, (getter) function, (default and parameterized constructor), and( print) to print the attributes values ➜ Create three objects in the main function then call the method. EX2: Write a complete C++ program to implement a student class. ➜ The class has three attributes:std-Id, Name and marks [3]. Also, the class include the following methods: (setter) function,(getter) functions (default and parameterized constructor), (average) and(print) to print the attributes values ➜ Create array of objects in the main function then call the method.arrow_forwardoop using c++ please give me full answer thanks Write a Program that implements Base class calculator and a Derived class scientific calculator, The Derived class should take the basic operations i.e. add, divide, subtract from calculator. However it should have its own methods such as square root.arrow_forwardC++ #include <string>#include <cmath> class DollarAmount {public:// initialize amount from an int64_t valueexplicit DollarAmount(int64_t value) : amount{value} { } // add right's amount to this object's amountvoid add(DollarAmount right) {// can access private data of other objects of the same classamount += right.amount; } // subtract right's amount from this object's amountvoid subtract(DollarAmount right) {// can access private data of other objects of the same classamount -= right.amount;} // uses integer arithmetic to calculate interest amount, // then calls add with the interest amountvoid addInterest(int rate, int divisor) {// create DollarAmount representing the interestDollarAmount interest{(amount * rate + divisor / 2) / divisor}; add(interest); // add interest to this object's amount} // return a string representation of a DollarAmount objectstd::string toString() const {std::string dollars{std::to_string(amount / 100)};std::string…arrow_forward
- a) Given the following code segments: class MyClass { String name; double value; MyClass (String n, double v) { name = n; value =v; String getName () { return name; } double getValue () { return value; } }arrow_forwardC++ Define and implement a class named Movie with the following members: std::string name; float cost; bool signedByDirector; Movie(); Movie(std::string movietitle); std::string getName (); float getCost(); void setCost (float newCost); // Price is modified by signing (see description) // Change cost to newCost // Returns signedByDirector bool issigned (); void signMovie(); Ensure each variable and function has been set an appropriate access modifier and your header file includes a header guard. Your methodgetCost() should return cost, but if the Movie is signed, it should return the cost plus a markup of 20%. For example, if a movie was worth $100, getCost () should return 100. If the same movie was signed by the author, then getCost() should return 120. Note: This function should not modify the class member cost, instead it should use cost and signedByDirector to determine the actual cost/value of the Movie. Your default constructor should set the cost to 0, the title to "NO TITLE".…arrow_forwardclockType.h file provided //clockType.h, the specification file for the class clockType#ifndef H_ClockType#define H_ClockType class clockType {public: void setTime(int hours, int minutes, int seconds); //Function to set the time. //The time is set according to the parameters. //Postcondition: hr = hours; min = minutes; // sec = seconds // The function checks whether the values of // hours, minutes, and seconds are valid. If a // value is invalid, the default value 0 is // assigned. void getTime(int& hours, int& minutes, int& seconds) const; //Function to return the time. //Postcondition: hours = hr; minutes = min; // seconds = sec void printTime() const; //Function to print the time. //Postcondition: The time is printed in the form // hh:mm:ss. void incrementSeconds(); //Function to increment the time by one…arrow_forward
- complex.h#pragma once#include <iostream>#include "imaginary.h"using namespace std; class Complex { private: int real; Imaginary imagine; public: //YOU: Implement all these functions Complex(); //Default constructor Complex(int new_real, Imaginary new_imagine); //Two parameter constructor Complex operator+(const Complex &rhs) const; Complex operator-(const Complex &rhs) const; Complex operator*(const Complex &rhs) const; bool operator==(const Complex &rhs) const; Complex operator^(const int &exponent) const; friend ostream& operator<<(ostream &lhs,const Complex& rhs); friend istream& operator>>(istream &lhs,Complex& rhs);}; complex.cc#include <iostream>#include "complex.h"using namespace std; //Class definition file for Complex //YOU: Fill in all of these functions//There are stubs (fake functions) in there now so that it will…arrow_forwardcomplex.h #pragma once #include <iostream> #include "imaginary.h" using namespace std; class Complex { private: int real; Imaginary imagine; public: //YOU: Implement all these functions Complex(); //Default constructor Complex(int new_real, Imaginary new_imagine); //Two parameter constructor Complex operator+(const Complex &rhs) const; Complex operator-(const Complex &rhs) const; Complex operator*(const Complex &rhs) const; bool operator==(const Complex &rhs) const; Complex operator^(const int &exponent) const; friend ostream& operator<<(ostream &lhs,const Complex& rhs); friend istream& operator>>(istream &lhs,Complex& rhs); }; complex.cc #include <iostream> #include "complex.h" using namespace std; //Class definition file for Complex //YOU: Fill in all of these functions //There are stubs (fake functions)…arrow_forwardC++ #include <iostream>using namespace std; //creating struct to hold //student name,age and letter gradestruct student{ //data members string name; int age; char grade;};int main(){ //declaring object for the struct student *k = new student; //filling it with data k->name = "Kate"; k->age=24; k->grade='B'; ///then printing student data cout<<"Name:"<<k->name<<endl; cout<<"Age:"<<k->age<<endl; cout<<"Grade:"<<k->grade<<endl; return 0;}arrow_forward
- **please see question attached** (previous program below) #include <iostream>using namespace std;class House{//instance variablesprivate:string location;int price; public://constructorsHouse(){location = "TBD";price = 0;}House(string location,int price){this->location = location;this->price = price;}//Get and set methodsvoid setLocation(string location){this->location = location;}string getLocation(){return location;}void setPrice(int price){this->price = price;}int getPrice(){return price;} };//member functionvoid output(House a){cout <<"Location: " << a.getLocation()<<endl;cout <<"Price: "<<a.getPrice()<<endl;}int main(){House a("1234 qcc st, Bayside, NY",1000000);output(a);House b;output(b); return 0;}arrow_forwardRemove the errors from the given code. #include <iostream>using namespace std; class age{ public: void print(int y){ cout<<2023-y; }} int main(){ age:: print(2000.0); return 0;}arrow_forwarddo part 4 import java.util.*; // Car classclass Car{ private String name; // Variable to hold car name private String model; // Variable to hold car model // Default constructor Car(){ this.name = null; this.model = null; } // Parametrised constructor Car(String name, String model){ this.name = name; this.model = model; } // Function to get car name public String getName(){ return this.name; }} // Dealer classclass Dealer{ private Car[] arr; // Array holding car objects for a dealer private int count; // Variable to hold number of cars under a dealer // Default constructor Dealer(){ arr = new Car[50]; count=0; } // Function to add a car under a dealer public void addCar(Car obj){ this.arr[this.count] = obj; this.count++; } // Function to check if a car exists under a dealer or not public boolean contains(String name){…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
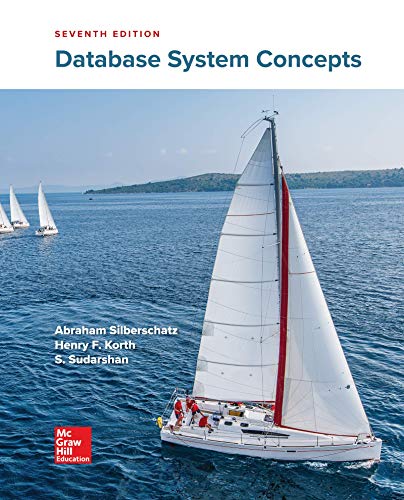
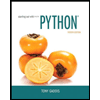
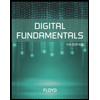
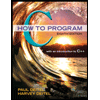
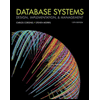
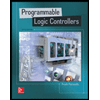