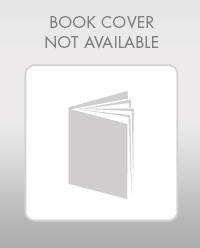
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 15, Problem 6PC
Program Plan Intro
Removal of Line Breaks
Program Plan:
- Include the required header files to the program.
- Definition for filter class.
- In public, declare the function for “do_Filter()”.
- In protected, declare the function for “transform(char ch)”.
- Include the required function prototypes.
- Function definition of the function “do_Filter()”.
- Declare the variable "letter" to get the character stored in the file.
- Loop to check the end of file.
- Output the characters to the file.
- Get the character from the input file.
- Derived class “Line_Break”.
- In protected, define the “transform ()” function.
- Inside the function return the value to the function.
- In public, declare the “do_Filter ()” function.
- In protected, define the “transform ()” function.
- Definition of “do_Filter ()” function.
- Check the input file contains the line breaks.
- If so, removal the line breaks and adds a space between the each word.
- Otherwise, call the “transform ()” function.
- Declare the “File_print()” function.
- Check the input file contains the line breaks.
- Define the main() function.
- Declare the require variable to hold the input and output file.
- Get the file name from the user.
- Create an object for the input file.
- Check the file open condition using “while” loop.
- Exit the program for invalid filename.
- Call the function “File_print()”.
- Get the output file name from the user.
- Create an object for the output file.
- Check the file open condition using “while” loop.
- Exit the program for invalid filename.
- Creating an object for “Line_Break ()”.
- Close output file.
- Re open the file to print the content.
- Close all files.
- Function definition for the function “File_print()”.
- Clear the content of the file.
- Seek arbitrary position in the file.
- Loop to check the end of file.
- Print the character.
- Clear the content of the file.
- Seek arbitrary position in the file
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Please original work
What topic would be related to architectures or infrastructures.
How you would implement your chosen topic in a data warehouse project.
Please cite in text references and add weblinks
What is cloud computing and why do we use it? Give one of your friends with your answer.
What are triggers and how do you invoke them on demand? Give one reference with your answer.
Chapter 15 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 15.3 - Prob. 15.1CPCh. 15.3 - Prob. 15.2CPCh. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - How can you tell from looking at a class...Ch. 15.3 - What makes an abstract class different from other...Ch. 15.3 - Examine the following classes. The table lists the...Ch. 15 - A class that cannot be instantiated is a(n) _____...
Ch. 15 - A member function of a class that is not...Ch. 15 - A class with at least one pure virtual member...Ch. 15 - In order to use dynamic binding, a member function...Ch. 15 - Static binding takes place at _____ time.Ch. 15 - Prob. 6RQECh. 15 - Prob. 7RQECh. 15 - Prob. 8RQECh. 15 - The is-a relation between classes is best...Ch. 15 - The has-a relation between classes is best...Ch. 15 - If every C1 class object can be used as a C2 class...Ch. 15 - A collection of abstract classes defining an...Ch. 15 - The keyword _____ prevents a virtual member...Ch. 15 - To have the compiler check that a virtual member...Ch. 15 - C++ Language Elements Suppose that the classes Dog...Ch. 15 - Will the statement pAnimal = new Cat; compile?Ch. 15 - Will the statement pCreature = new Dog ; compile?Ch. 15 - Will the statement pCat = new Animal; compile?Ch. 15 - Rewrite the following two statements to get them...Ch. 15 - Prob. 20RQECh. 15 - Find all errors in the following fragment of code,...Ch. 15 - Soft Skills 22. Suppose that you need to have a...Ch. 15 - Prob. 1PCCh. 15 - Prob. 2PCCh. 15 - Sequence Sum A sequence of integers such as 1, 3,...Ch. 15 - Prob. 4PCCh. 15 - File Filter A file filter reads an input file,...Ch. 15 - Prob. 6PCCh. 15 - Bumper Shapes Write a program that creates two...Ch. 15 - Bow Tie In Tying It All Together, we defined a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Discuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forwardDetermine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward• Solve the problem (pls refer to the inserted image)arrow_forward
- Write .php file that saves car booking and displays feedback. There are 2 buttons, which are <Book it> <Select a date>. <Select a date> button gets an input from the user, start date and an end date. Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, it books cars for specific dates, with bookings saved. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. In the booking.json file, if the Car ID and start date and end date matches, it fails Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert).arrow_forwardWrite .php file with the html that saves car booking and displays feedback. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. There are 2 buttons, which are <Book it> <Select a date> Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, book cars for specific dates, with bookings saved. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert). And then add an additional feature that only free dates are selectable (e.g., calendar view).arrow_forward• Solve the problem (pls refer to the inserted image) and create line graph.arrow_forward
- who started the world wide webarrow_forwardQuestion No 1: (Topic: Systems for collaboration and social business The information systems function in business) How does Porter's competitive forces model help companies develop competitive strategies using information systems? • List and describe four competitive strategies enabled by information systems that firms can pursue. • Describe how information systems can support each of these competitive strategies and give examples.arrow_forwardData communıcatıon digital data is transmitted via analog ASK and PSK are used together to increase the number of bits transmitted a)For m=8,suggest a solution and define signal elements , and then draw signals for the following sent data data = 0 1 0 1 1 0 0 0 1 0 1 1arrow_forward
- DatacommunicationData = 1 1 0 0 1 0 0 1 0 1 1 1 1 0 0a) how many bıts can be detected and corrected by this coding why prove?b)what wıll be the decision of the reciever if it recieve the following codewords why?arrow_forwardpattern recognitionPCA algor'thmarrow_forwardConsider the following program: LOAD AC, IMMEDIATE(30) ADD AC, REGISTER(R1) STORE AC, MEMORY(20) Given that the value of R1 is 50, determine the value stored at memory address 20 after the program is executed. Provide an explanation to support your answer.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
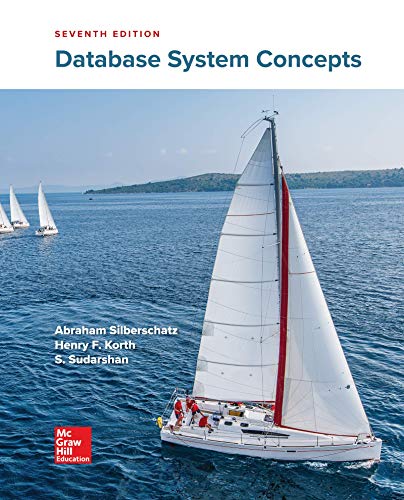
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
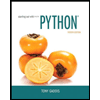
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
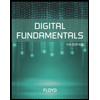
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
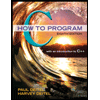
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
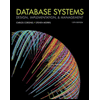
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
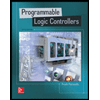
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education