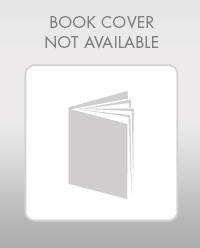
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 15, Problem 4PC
Program Plan Intro
Flexible Encryption
- Include the required header files to the program.
- Define “Encryption” class.
- In public, create a constructor, destructor and pure virtual function.
- In protected, declare the required variable.
- Define the constructor.
- Open the input file and output file.
- If the input file is not present, exit the program.
- If the output file is not present, exit the program.
- Define the destructor.
- Close the input and output file.
- Define the subclass “encrypt” function.
- Declare the required variables.
- Read the input file and write the encrypted form to the output file.
- Define the “Encryption” function.
- In public, define the “transform” function.
- Return the encrypted value.
- In public, define the “transform” function.
- Define the “main()” function.
- Declare the required variables.
- Get the input and output file name from the user.
- Create the objects for the classes.
- Get the encryption key from the user.
- Call the function “setKey” function with the key value.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Please do not use any AI tools to solve this question.
I need a fully manual, step-by-step solution with clear explanations, as if it were done by a human tutor.
No AI-generated responses, please.
Obtain the MUX design for the function F(X,Y,Z) = (0,3,4,7) using an off-the-shelf MUX with an active low strobe input (E).
I cannot program smart home automation rules from my device using a computer or phone, and I would like to know how to properly connect devices such as switches and sensors together ?
Cisco Packet Tracer
1. Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.
Chapter 15 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 15.3 - Prob. 15.1CPCh. 15.3 - Prob. 15.2CPCh. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - What will the following program display? #include...Ch. 15.3 - How can you tell from looking at a class...Ch. 15.3 - What makes an abstract class different from other...Ch. 15.3 - Examine the following classes. The table lists the...Ch. 15 - A class that cannot be instantiated is a(n) _____...
Ch. 15 - A member function of a class that is not...Ch. 15 - A class with at least one pure virtual member...Ch. 15 - In order to use dynamic binding, a member function...Ch. 15 - Static binding takes place at _____ time.Ch. 15 - Prob. 6RQECh. 15 - Prob. 7RQECh. 15 - Prob. 8RQECh. 15 - The is-a relation between classes is best...Ch. 15 - The has-a relation between classes is best...Ch. 15 - If every C1 class object can be used as a C2 class...Ch. 15 - A collection of abstract classes defining an...Ch. 15 - The keyword _____ prevents a virtual member...Ch. 15 - To have the compiler check that a virtual member...Ch. 15 - C++ Language Elements Suppose that the classes Dog...Ch. 15 - Will the statement pAnimal = new Cat; compile?Ch. 15 - Will the statement pCreature = new Dog ; compile?Ch. 15 - Will the statement pCat = new Animal; compile?Ch. 15 - Rewrite the following two statements to get them...Ch. 15 - Prob. 20RQECh. 15 - Find all errors in the following fragment of code,...Ch. 15 - Soft Skills 22. Suppose that you need to have a...Ch. 15 - Prob. 1PCCh. 15 - Prob. 2PCCh. 15 - Sequence Sum A sequence of integers such as 1, 3,...Ch. 15 - Prob. 4PCCh. 15 - File Filter A file filter reads an input file,...Ch. 15 - Prob. 6PCCh. 15 - Bumper Shapes Write a program that creates two...Ch. 15 - Bow Tie In Tying It All Together, we defined a...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- using r language for integration theta = integral 0 to infinity (x^4)*e^(-x^2)/2 dx (1) use the density function of standard normal distribution N(0,1) f(x) = 1/sqrt(2pi) * e^(-x^2)/2 -infinity <x<infinity as importance function and obtain an estimate theta 1 for theta set m=100 for the estimate whatt is the estimate theta 1? (2)use the density function of gamma (r=5 λ=1/2)distribution f(x)=λ^r/Γ(r) x^(r-1)e^(-λx) x>=0 as importance function and obtain an estimate theta 2 for theta set m=1000 fir the estimate what is the estimate theta2? (3) use simulation (repeat 1000 times) to estimate the variance of the estimates theta1 and theta 2 which one has smaller variance?arrow_forwardusing r language A continuous random variable X has density function f(x)=1/56(3x^2+4x^3+5x^4).0<=x<=2 (1) secify the density g of the random variable Y you find for the acceptance rejection method. (2) what is the value of c you choose to use for the acceptance rejection method (3) use the acceptance rejection method to generate a random sample of size 1000 from the distribution of X .graph the density histogram of the sample and compare it with the density function f(x)arrow_forwardusing r language a continuous random variable X has density function f(x)=1/4x^3e^-(pi/2)^4,x>=0 derive the probability inverse transformation F^(-1)x where F(x) is the cdf of the random variable Xarrow_forward
- using r language in an accelerated failure test, components are operated under extreme conditions so that a substantial number will fail in a rather short time. in such a test involving two types of microships 600 chips manufactured by an existing process were tested and 125 of them failed then 800 chips manufactured by a new process were tested and 130 of them failed what is the 90%confidence interval for the difference between the proportions of failure for chips manufactured by two processes? using r languagearrow_forwardI want a picture of the tools and the pictures used Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardA. What will be printed executing the code above?B. What is the simplest way to set a variable of the class Full_Date to January 26 2020?C. Are there any empty constructors in this class Full_Date?a. If there is(are) in which code line(s)?b. If there is not, how would an empty constructor be? (create the code lines for it)D. Can the command std::cout << d1.m << std::endl; be included after line 28 withoutcausing an error?a. If it can, what will be printed?b. If it cannot, how could this command be fixed?arrow_forward
- Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardTransform the TM below that accepts words over the alphabet Σ= {a, b} with an even number of a's and b's in order that the output tape head is positioned over the first letter of the input, if the word is accepted, and all letters a should be replaced by the letter x. For example, for the input aabbaa the tape and head at the end should be: [x]xbbxx z/z,R b/b,R F ① a/a,R b/b,R a/a, R a/a,R b/b.R K a/a,R L b/b,Rarrow_forwardGiven the C++ code below, create a TM that performs the same operation, i.e., given an input over the alphabet Σ= {a, b} it prints the number of letters b in binary. 1 #include 2 #include 3 4- int main() { std::cout > str; for (char c : str) { if (c == 'b') count++; 5 std::string str; 6 int count = 0; 7 char buffer [1000]; 8 9 10 11- 12 13 14 } 15 16- 17 18 19 } 20 21 22} std::string binary while (count > 0) { binary = std::to_string(count % 2) + binary; count /= 2; std::cout << binary << std::endl; return 0;arrow_forward
- Considering the CFG described below, answer the following questions. Σ = {a, b} • NT = {S} Productions: P1 S⇒aSa P2 P3 SbSb S⇒ a P4 S⇒ b A. List one sequence of productions that can accept the word abaaaba; B. Give three 5-letter words that can be accepted by this CFG; C. Create a Pushdown automaton capable of accepting the language accepted by this CFG.arrow_forwardGiven the FSA below, answer the following questions. b 1 3 a a b b с 2 A. Write a RegEx that is equivalent to this FSA as it is; B. Write a RegEx that is equivalent to this FSA removing the states and edges corresponding to the letter c. Note: To both items feel free to use any method you want, including analyzing which words are accepted by the FSA, to generate your RegEx.arrow_forward3) Finite State Automata Given the FSA below, answer the following questions. a b a b 0 1 2 b b 3 A. Give three 4-letter words that can be accepted by this FSA; B. Give three 4-letter words that cannot be accepted by this FSA; C. How could you describe the words accepted by this FSA? D. Is this FSA deterministic or non-deterministic?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
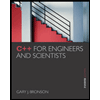
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
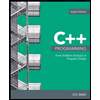
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
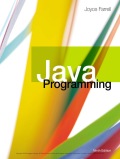
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
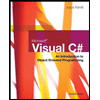
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
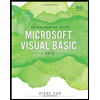
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage