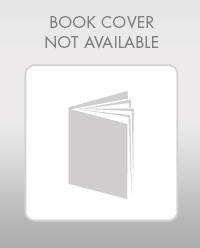
Concept explainers
Sequence Sum
A sequence of integers such as 1, 3, 5, 7,... can be represented by a function that takes a non-negative integer as parameter and returns the corresponding term of the sequence. For example, the sequence of odd numbers just cited can be represented by the function
int odd(int k) {return 2 * k + 1;}
Write an abstract class AbstractSeq that has a pure virtual member function
virtual int fun(int k) = 0;
as a stand-in for an actual sequence, and two member functions
void printSeq(int k, int m);
int sumSeq(int k, int m)
that are passed two integer parameters k and m, where k < m. The function printSeq will print all the terms fun(k) through fun(m) of the sequence, and likewise, the function sumSeq will return the sum of those terms. Demonstrate your AbstractSeq class by creating subclasses that you use to sum the terms of at least two different sequences. Determine what kind of output best shows off the operation of these classes, and write a

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Starting Out With C++: Early Objects (10th Edition)
Additional Engineering Textbook Solutions
Database Concepts (8th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Absolute Java (6th Edition)
Starting Out with Java: Early Objects (6th Edition)
Starting out with Visual C# (4th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
- Data Structuresarrow_forwardOne use of the this pointer is to access a data member when there is a local variable with the same name. For example, if a member function has a local variable x and a data member x, then you can refer to the data member as this->x. Use this fact to complete the constructor implementation of this Point class: class Point { public: Point(int x, int y); int get_x() const; int get_y() const; private: int x; int y; }; Not all lines are useful.arrow_forwardAll virtual functions in an abstract base class must be declared as pure virtual functions T/Farrow_forward
- CONSTRUCTOR// IntSet()// Pre: (none)// Post: The invoking IntSet is initialized to an empty// IntSet (i.e., one containing no relevant elements).// CONSTANT MEMBER FUNCTIONS (ACCESSORS)// int size() const// Pre: (none)// Post: Number of elements in the invoking IntSet is returned.// bool isEmpty() const// Pre: (none)// Post: True is returned if the invoking IntSet has no relevant// relevant elements, otherwise false is returned.// bool contains(int anInt) const// Pre: (none)// Post: true is returned if the invoking IntSet has anInt as an// element, otherwise false is returned.// bool isSubsetOf(const IntSet& otherIntSet) const// Pre: (none)// Post: True is returned if all elements of the invoking IntSet// are also elements of otherIntSet, otherwise false is// returned.// By definition, true is returned if the invoking IntSet// is empty (i.e., an empty IntSet…arrow_forwardAn abstract class a. Cannot be used to declare pointers and references b. Has at least one pure virtual function c. Can be instantiated d. Cannot have any constructorsarrow_forwardIn number theory, a value can be categorized as a natural number (a whole number >0, often denoted ℕ), an integer (zero or a positive or negative whole number, including the natural numbers, often denoted ℤ), or a real number (which includes the natural numbers and integers, along with all other positive and negative numbers that are not integers, often denoted ℝ). a) write a definition for a number class that contains: (i) A single field suitable for storing either a natural number, or an integer, or a real number; (ii) Setter and getter methods for manipulating this field; (iii) A constructor that initializes new objects of number to have the value 1 (unity); (iv) a method that determines which kind of number is currently stored (returning 0 if the number is real and an integer and a natural number, 1 if the number is real and integer but not a natural number, and 2 if the number is real but neither an integer nor a natural number) Java code neededarrow_forward
- make the flowchart for this code plz abstract class StudentCalculation /ABSTRACTION/{abstract int totalStrength();abstract int fees();}class College{private String collegeName;private int rank;private String address;College(String name,int r,String add){collegeName = name;rank = r;address = add;}public void setName(String n){collegeName = n;}public String getName(){return(collegeName);}public void setRank(int r){rank = r;}public int getRank(){return(rank);}public void setAddress(String a){address = a;}public String getAddress(){return address;}}class Deptcse extends StudentCalculation /INHERITENCE/{private int numberOfTeachers, numberOfMale, numberOfFeamle;Deptcse(int t,int m, int f){numberOfTeachers=t;numberOfMale = m;numberOfFeamle = f;}public String getName(){return("Computer Science and Engineering");}public void setTeacher(int t){numberOfTeachers=t;}public int getTeacher(){return(numberOfTeachers);}public void setMalestd(int m){numberOfMale = m;}public int…arrow_forwardarray of Payroll ObjectsDesign a PayRoll class that has data members for an employee’shourly pay rate and number of hours worked. Write a program withan array of seven PayRoll objects. The program should read thenumber of hours each employee worked and their hourly pay ratefrom a file and call class functions to store this information in theappropriate objects. It should then call a class function, once foreach object, to return the employee’s gross pay, so this informationcan be displayed.arrow_forwardDeclaring a function that is not a member of a class C as a(n) to C allows that function to access all of C's private and protected members.arrow_forward
- Course Title: Modern Programming Language Please Java Language Code and Correct Code Please Question : Design an abstract class GeometricObject. GeometricObject must ensure that its children must implement calcArea() method. Design Rectangle18ARID2891 and CircleMJibranAkram Classes as children of GeometricObject class with overridden toString() method to return “Rectangle18ARID2891 with w Width and h Height is drawn” OR “CircleMJibranAkram with r Radius is drawn”. The attributes of Rectangle18ARID2891 are length, width. The attribute of CircleMJibranAkram is radius Hint: Area of circle=πr2 , Area of rectangle= width*lengtharrow_forwardPoker card in PythonLet the CarteBase class present itself in the context of this exercise. It encapsulates a game card characterized by numerical values for its face and its kind. The possible faces are integers from 1 to 13, with face 1 corresponding to the ace, and faces 11, 12 and 13 corresponding respectively to the jack, the queen and the king. The other faces are simply identified by their number from 2 to 10. The possible grades are integers from 1 to 4 corresponding respectively to the cards of spade, heart, tile and clover. Analyze this class to understand how it works. You are asked to create a class called CarteDePoker that inherits CarteBase and defines or redefines the following methods: force(self) which returns the strength of the card in the poker game. In Poker, cards have the strength of their digital face from 2 to 13, except for the ace which is stronger than the other cards. So we give it a strength of 14.__eq__(self, other) to define operator behavior…arrow_forwardCircular Queue: A circular queue is the extended version of a regular queue where the last element is connected to the first element. Thus forming a circle-like structure. Create a C++ generic abstract class named as CircularQueue with the following: Attributes: Type*arr; int front; int rear; int maxSize; Functions: virtual void enqueue(Type) = 0; Adds the element of type Type at the end of the circular queue. virtual Type dequeue() = 0; Deletes the first most element of the circular queue and returns it. PLEASE USE C++arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
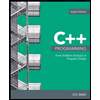
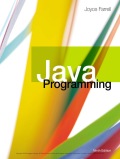
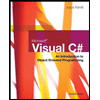
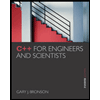