omplex.h #pragma once #include #include "imaginary.h" using namespace std; class Complex { private: int real; Imaginary imagine; public: //YOU: Implement all these functions Complex(); //Default constructor Complex(int new_real, Imaginary new_imagine); //Two parameter constructor Complex operator+(const Complex &rhs) const; Complex operator-(const Complex &rhs) const; Complex operator*(const Complex &rhs) const; bool operator==(const Complex &rhs) const; Complex operator^(const int &exponent) const; friend ostream& operator<<(ostream &lhs,const Complex& rhs); friend istream& operator>>(istream &lhs,Complex& rhs); }; complex.cc #include #include "complex.h" using namespace std; //Class definition file for Complex //YOU: Fill in all of these functions //There are stubs (fake functions) in there now so that it will compile //The stubs should be removed and replaced with your own code. Complex::Complex() { } Complex::Complex(int new_real, Imaginary new_imagine) { } Complex Complex::operator+(const Complex &rhs) const { } Complex Complex::operator-(const Complex &rhs) const { } Complex Complex::operator*(const Complex &rhs) const { } bool Complex::operator==(const Complex &rhs) const { } Complex Complex::operator^(const int &exponent) const { } //This function should output 3+5i for Complex(3,5), etc. ostream& operator<<(ostream &lhs,const Complex& rhs) { //Output a Complex here } //This function should read in two ints, and construct a // new Complex with those two ints istream& operator>>(istream &lhs,Complex& rhs) { //Read in a Complex here } imaginary.h #pragma once #include using namespace std; class Imaginary { private: int coeff; //If 5, then means 5i public: Imaginary(); Imaginary(int new_coeff); int get_coeff() const; Imaginary operator+(const Imaginary& rhs) const; //This is a "constant method" Imaginary operator-(const Imaginary& rhs) const; int operator*(const Imaginary& rhs) const; Imaginary operator*(const int& rhs) const; Imaginary operator=(const int& rhs); Imaginary operator=(const Imaginary& rhs); bool operator==(const Imaginary& rhs) const; friend ostream& operator<<(ostream& lhs, const Imaginary& rhs); friend istream& operator>>(istream& lhs, Imaginary& rhs); }; imaginary.cc #include "imaginary.h" #include using namespace std; //Sample Code - I have done the addition operator for you so you can see //what a functioning operator should look like. Given this function below, //in main() you could write the following code: // Imaginary foo(3); //foo is 3i // Imaginary bar(5); //bar is 5i // foo = foo + bar; //foo will become 8i //In the above example, this function would get called on foo, with //bar being passed in as the parameter named rhs (right hand side). Imaginary Imaginary::operator+(const Imaginary& rhs) const { return Imaginary(coeff+rhs.coeff); //My coeff is 3; rhs.coeff is 5. So construct a new one with a coeff of 8. } //These you will need to implement yourself. //They currently are just stub functions Imaginary::Imaginary() { //Default cstor //coeff = ?? } Imaginary::Imaginary(int new_coeff) { //One parameter cstor //coeff = ?? } int Imaginary::get_coeff() const { //Get function return 0; //Change this } Imaginary Imaginary::operator-(const Imaginary& rhs) const { return Imaginary(coeff-rhs.coeff); } Imaginary Imaginary::operator*(const int& rhs) const { //5i * 2 = 10i } int Imaginary::operator*(const Imaginary& rhs) const { //i * i = -1 } //This function is functional Imaginary Imaginary::operator=(const Imaginary& rhs) { coeff = rhs.coeff; return rhs; } //This function is functional Imaginary Imaginary::operator=(const int& rhs) { coeff = rhs; return Imaginary(rhs); } bool Imaginary::operator==(const Imaginary& rhs) const { return (true); } //This function is done for you. It will allow you to cout variables of type Imaginary. //For example, in main you could write: // Imaginary foo(2); // cout << foo << endl; //And this would print out "2i" ostream& operator<<(ostream& lhs, const Imaginary& rhs) { lhs << showpos; lhs << rhs.coeff << "i"; //Will echo +4i or +0i or -3i or whatever lhs << noshowpos; return lhs; } istream& operator>>(istream& lhs, Imaginary& rhs) { int i; lhs >> i; rhs.coeff = i; return lhs; } HELP ME WITH HOMEWORK I CONFUSE. THANK YOU SO MUCH C++
complex.h
#pragma once
#include <iostream>
#include "imaginary.h"
using namespace std;
class Complex {
private:
int real;
Imaginary imagine;
public:
//YOU: Implement all these functions
Complex(); //Default constructor
Complex(int new_real, Imaginary new_imagine); //Two parameter constructor
Complex operator+(const Complex &rhs) const;
Complex operator-(const Complex &rhs) const;
Complex operator*(const Complex &rhs) const;
bool operator==(const Complex &rhs) const;
Complex operator^(const int &exponent) const;
friend ostream& operator<<(ostream &lhs,const Complex& rhs);
friend istream& operator>>(istream &lhs,Complex& rhs);
};
complex.cc
#include <iostream>
#include "complex.h"
using namespace std;
//Class definition file for Complex
//YOU: Fill in all of these functions
//There are stubs (fake functions) in there now so that it will compile
//The stubs should be removed and replaced with your own code.
Complex::Complex() {
}
Complex::Complex(int new_real, Imaginary new_imagine) {
}
Complex Complex::operator+(const Complex &rhs) const {
}
Complex Complex::operator-(const Complex &rhs) const {
}
Complex Complex::operator*(const Complex &rhs) const {
}
bool Complex::operator==(const Complex &rhs) const {
}
Complex Complex::operator^(const int &exponent) const {
}
//This function should output 3+5i for Complex(3,5), etc.
ostream& operator<<(ostream &lhs,const Complex& rhs) {
//Output a Complex here
}
//This function should read in two ints, and construct a
// new Complex with those two ints
istream& operator>>(istream &lhs,Complex& rhs) {
//Read in a Complex here
}
imaginary.h
#pragma once
#include <iostream>
using namespace std;
class Imaginary {
private:
int coeff; //If 5, then means 5i
public:
Imaginary();
Imaginary(int new_coeff);
int get_coeff() const;
Imaginary operator+(const Imaginary& rhs) const; //This is a "constant method"
Imaginary operator-(const Imaginary& rhs) const;
int operator*(const Imaginary& rhs) const;
Imaginary operator*(const int& rhs) const;
Imaginary operator=(const int& rhs);
Imaginary operator=(const Imaginary& rhs);
bool operator==(const Imaginary& rhs) const;
friend ostream& operator<<(ostream& lhs, const Imaginary& rhs);
friend istream& operator>>(istream& lhs, Imaginary& rhs);
};
imaginary.cc
#include "imaginary.h"
#include <iomanip>
using namespace std;
//Sample Code - I have done the addition operator for you so you can see
//what a functioning operator should look like. Given this function below,
//in main() you could write the following code:
// Imaginary foo(3); //foo is 3i
// Imaginary bar(5); //bar is 5i
// foo = foo + bar; //foo will become 8i
//In the above example, this function would get called on foo, with
//bar being passed in as the parameter named rhs (right hand side).
Imaginary Imaginary::operator+(const Imaginary& rhs) const {
return Imaginary(coeff+rhs.coeff); //My coeff is 3; rhs.coeff is 5. So construct a new one with a coeff of 8.
}
//These you will need to implement yourself.
//They currently are just stub functions
Imaginary::Imaginary() { //Default cstor
//coeff = ??
}
Imaginary::Imaginary(int new_coeff) { //One parameter cstor
//coeff = ??
}
int Imaginary::get_coeff() const { //Get function
return 0; //Change this
}
Imaginary Imaginary::operator-(const Imaginary& rhs) const {
return Imaginary(coeff-rhs.coeff);
}
Imaginary Imaginary::operator*(const int& rhs) const {
//5i * 2 = 10i
}
int Imaginary::operator*(const Imaginary& rhs) const {
//i * i = -1
}
//This function is functional
Imaginary Imaginary::operator=(const Imaginary& rhs) {
coeff = rhs.coeff;
return rhs;
}
//This function is functional
Imaginary Imaginary::operator=(const int& rhs) {
coeff = rhs;
return Imaginary(rhs);
}
bool Imaginary::operator==(const Imaginary& rhs) const {
return (true);
}
//This function is done for you. It will allow you to cout variables of type Imaginary.
//For example, in main you could write:
// Imaginary foo(2);
// cout << foo << endl;
//And this would print out "2i"
ostream& operator<<(ostream& lhs, const Imaginary& rhs) {
lhs << showpos;
lhs << rhs.coeff << "i"; //Will echo +4i or +0i or -3i or whatever
lhs << noshowpos;
return lhs;
}
istream& operator>>(istream& lhs, Imaginary& rhs) {
int i;
lhs >> i;
rhs.coeff = i;
return lhs;
}
HELP ME WITH HOMEWORK I CONFUSE. THANK YOU SO MUCH C++


Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 2 images

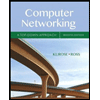
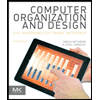
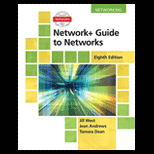
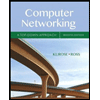
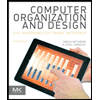
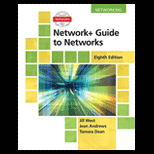
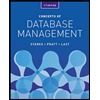
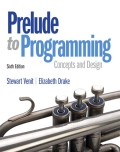
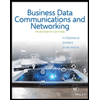