pragma once #includ
complex.h
#pragma once
#include <iostream>
#include "imaginary.h"
using namespace std;
class Complex {
private:
int real;
Imaginary imagine;
public:
//YOU: Implement all these functions
Complex(); //Default constructor
Complex(int new_real, Imaginary new_imagine); //Two parameter constructor
Complex operator+(const Complex &rhs) const;
Complex operator-(const Complex &rhs) const;
Complex operator*(const Complex &rhs) const;
bool operator==(const Complex &rhs) const;
Complex operator^(const int &exponent) const;
friend ostream& operator<<(ostream &lhs,const Complex& rhs);
friend istream& operator>>(istream &lhs,Complex& rhs);
};
complex.cc
#include <iostream>
#include "complex.h"
using namespace std;
//Class definition file for Complex
//YOU: Fill in all of these functions
//There are stubs (fake functions) in there now so that it will compile
//The stubs should be removed and replaced with your own code.
Complex::Complex() {
real = 0;
imagine = 0;
}
Complex::Complex(int new_real, Imaginary new_imagine) {
real = new_real;
image = new_image;
}
Complex Complex::operator+(const Complex &rhs) const {
Complex num;
num.real = real + rhs.real;
num.imagine = imagine + rhs.imagine;
return num;
}
Complex Complex::operator-(const Complex &rhs) const {
Complex num;
num.real = real - rhs.real;
num.imagine = imagine - rhs.imagine;
return num;
}
Complex Complex::operator*(const Complex &rhs) const {
Complex num;
num.real = (real * rhs.real) - (imagine * rhs.imagine);
num.imagine = (real * rhs.imagine) + (imagine * rhs.real);
return num;
}
bool Complex::operator==(const Complex &rhs) const {
if (real == rhs.real && imagine == rhs.imagine) {
return true;
}
else{
return false;
}
}
//Complex exponent
Complex Complex::operator^(const int &exponent) const {
CODE HERE
}
//This function should output 3+5i for Complex(3,5), etc.
ostream& operator<<(ostream &lhs,const Complex& rhs) {
//Output a Complex here
CODE HERE
}
//This function should read in two ints, and construct a
// new Complex with those two ints
istream& operator>>(istream &lhs,Complex& rhs) {
//Read in a Complex here
CODE HERE
}
imaginary.h
#pragma once
#include <iostream>
using namespace std;
class Imaginary {
private:
int coeff; //If 5, then means 5i
public:
Imaginary();
Imaginary(int new_coeff);
int get_coeff() const;
Imaginary operator+(const Imaginary& rhs) const; //This is a "constant method"
Imaginary operator-(const Imaginary& rhs) const;
int operator*(const Imaginary& rhs) const;
Imaginary operator*(const int& rhs) const;
Imaginary operator=(const int& rhs);
Imaginary operator=(const Imaginary& rhs);
bool operator==(const Imaginary& rhs) const;
friend ostream& operator<<(ostream& lhs, const Imaginary& rhs);
friend istream& operator>>(istream& lhs, Imaginary& rhs);
};
main.cc
#include <iostream>
#include <iomanip>
#include <cstdlib>
#include "imaginary.h"
//YOU: include the header file for complex numbers
CODE HERE
using namespace std;
int main() {
cout << boolalpha; //Print "true" instead of "1" when outputting bools
Imaginary i,j; //These three lines are test code, delete them later
cin >> i >> j; //Read two Imaginaries in - won't work till cstors done
cout << i+j << endl; //Output the sum of them
while (true) {
//YOU: Read in a complex number
CODE HERE
//YOU: If it is 0 0, then break or exit
CODE HERE
exit(EXIT_SUCCESS);
//YOU: Read in an operator (+,-,*,==,or ^)
CODE HERE
//YOU: Read in the second operand (another complex or an int)
CODE HERE
//YOU: Output the result
CODE HERE
}
}
HELP ME THE HOMEWORK TYPE CODE. THANK YOU SO MUCH c++

main.cpp
#include <iostream>
#include "derComplex.h"
using namespace std;
int main()
{
Complex a(4.0, 6.0), b(3.0, 5.0), *c = new Complex;
Complex d(a);
d.print();
a.add(b).print();
derComplex res(7.0,9.0);
cout<<"\nComplex Number res is: ";
res.print();
cout<<"\nNegative of Complex number res is: ";
res.negative().print();
cout<<"\nConjugate of Complex number res is: ";
res.conjugate().print();
cout<<"\nSwaping of Complex number res is: ";
res.swap().print();
return 0;
}
Complex.h
#pragma once
#ifndef COMPLEX_H
#define COMPLEX_H
class Complex
{
public:
double real;
double imag;
public:
Complex() : real(0.0), imag(0.0) {}
Complex(double r, double i) : real(r), imag(i) {}
Complex(const Complex &c) : real(c.real), imag(c.imag) {}
// above statement must be pass-by-reference so that the default copy constructor is not used.
// if it is pass-by-value, the above statement is not a copy constructor and function
// overloading occurs the compiler cannot resolve
void setReal(double r) { real = r; }
void setImag(double i) { imag = i; }
void setComplex(Complex c) { real = c.real; imag = c.imag; }
double getReal() { return real; }
double getImag() { return imag; }
Complex add(const Complex &) const;
Complex sub(const Complex &) const;
Complex mul(const Complex &) const;
Complex div(const Complex &) const;
void print() const;
};
#endif
Complex.cpp
#include <iostream>
#include "Complex.h"
using namespace std;
Complex Complex::add(const Complex &c) const {
Complex result;
result.real = real + c.real;
result.imag = imag + c.imag;
return result;
}
Complex Complex::sub(const Complex &c) const {
Complex result;
result.real = real - c.real;
result.imag = imag - c.imag;
return result;
}
Complex Complex::mul(const Complex &c) const {
Complex result;
result.real = real c.real - imag c.imag;
result.imag = imag c.real + real c.imag;
return result;
}
Complex Complex::div(const Complex &c) const {
double t = c.real c.real + c.imag c.imag;
Complex result;
result.real = (real c.real + imag c.imag) / t;
result.imag = (imag c.real - real c.imag) / t;
return result;
}
void Complex::print() const {
cout << "( " << real << ", " << imag << " )\n";
}
derComplex.h
#include "Complex.h"
class derComplex : public Complex
{
public:
derComplex(){}
derComplex(double r, double i)
{
real=r;
imag=i;
}
derComplex negative() ;
derComplex conjugate() ;
derComplex swap() ;
};
derComplex.cpp
#include <iostream>
#include "derComplex.h"
using namespace std;
derComplex derComplex::negative()
{
derComplex temp;
temp.real = -1*this->real;
temp.imag = -1*this->imag;
return temp;
}
derComplex derComplex::conjugate()
{
derComplex temp;
temp.real = this->real;
temp.imag = -1*this->imag;
return temp;
}
derComplex derComplex::swap()
{
derComplex temp;
temp.real = this->imag;
temp.imag = this->real;
return temp;
}
OUTPUT
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

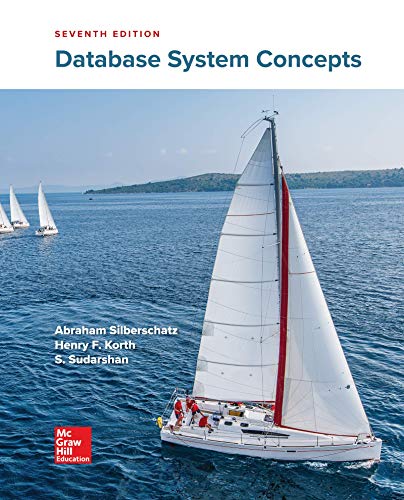
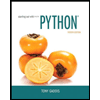
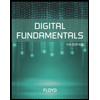
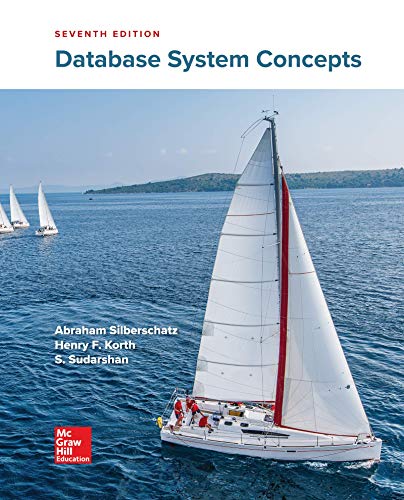
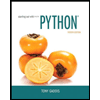
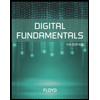
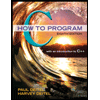
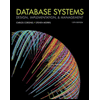
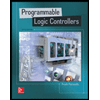