Please examine the C++ code and make corrections please. ##include using namespace std; //employee class class Employee { private: //fields string name, address, phone; double payRate; public: //cosntructors Employee() {} Employee(string n, string a, double p, string ph) { name = n; address = a; payRate = p; phone = p; } //accessors string getName() { return name; } string getAddress() { return address; } string getPhone() { return phone; } double getPayRate() { return payRate; } //setters void setName(string n) { name = n; } void setAddress(string a) { address = a; } void setPhone(string ph) { phone = ph; } void setPayRate(double pr) { payRate = pr; } }; //employee directory class class EmployeeDirectory { private: //all employees are added into this vector vector employees; public: EmployeeDirectory() {} //this function adds a new employee void addEmployee(string n, string a, double p, string ph) { //make employee object and add in vector Employee e(n, a, p, ph); employees.push_back(e); } //this function returns Employee object for the name searched Employee searchByName(string n) { //iterate all employees for (Employee e : employees) { //if name matches with given name then return the employee object if (e.getName() == n) return e; } //if employee not found, make an empty employee object and send Employee e; return e; } //prints all the employees, only names as given in question void displayAll() { cout << "All employees are : " << endl; for (Employee e : employees) { cout << e.getName() << endl; } cout << endl; } }; int main() { cout << "Everest Law This is CSC CSC 102 Final Exam - Employee Directory\n"; cout << "\n"; EmployeeDirectory directory; directory.addEmployee("Sarah Paulson", "1769 Polly Fork, West Nyasia, South Dakota", 1920, "(138) 705 9405"); directory.addEmployee("Tom Holland", "1769 Polly Fork, West Nyasia, South Dakota", 2500, "(991) 201 9415"); directory.addEmployee("Emma Watson", "1769 Polly Fork, West Nyasia, South Dakota", 2000, "(532) 427 2850"); directory.addEmployee("Leonardo Dicaprio", "1769 Polly Fork, West Nyasia, South Dakota", 4580, "(904) 188 3811"); directory.addEmployee("Maggie Smith", "1769 Polly Fork, West Nyasia, South Dakota", 1900, "(049) 737 8587"); directory.displayAll(); }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
Please examine the C++ code and make corrections please.
##include<bits / stdc++.h>
using namespace std;
//employee class
class Employee
{
private:
//fields
string name, address, phone;
double payRate;
public:
//cosntructors
Employee() {}
Employee(string n, string a, double p, string ph)
{
name = n;
address = a;
payRate = p;
phone = p;
}
//accessors
string getName() { return name; }
string getAddress() { return address; }
string getPhone() { return phone; }
double getPayRate() { return payRate; }
//setters
void setName(string n)
{
name = n;
}
void setAddress(string a) { address = a; }
void setPhone(string ph) { phone = ph; }
void setPayRate(double pr) { payRate = pr; }
};
//employee directory class
class EmployeeDirectory
{
private:
//all employees are added into this
vector<Employee> employees;
public:
EmployeeDirectory() {}
//this function adds a new employee
void addEmployee(string n, string a, double p, string ph)
{
//make employee object and add in vector
Employee e(n, a, p, ph);
employees.push_back(e);
}
//this function returns Employee object for the name searched
Employee searchByName(string n)
{
//iterate all employees
for (Employee e : employees)
{
//if name matches with given name then return the employee object
if (e.getName() == n)
return e;
}
//if employee not found, make an empty employee object and send
Employee e;
return e;
}
//prints all the employees, only names as given in question
void displayAll()
{
cout << "All employees are : " << endl;
for (Employee e : employees)
{
cout << e.getName() << endl;
}
cout << endl;
}
};
int main()
{
cout << "Everest Law This is CSC CSC 102 Final Exam - Employee Directory\n";
cout << "\n";
EmployeeDirectory directory;
directory.addEmployee("Sarah Paulson", "1769 Polly Fork, West Nyasia, South Dakota", 1920, "(138) 705 9405");
directory.addEmployee("Tom Holland", "1769 Polly Fork, West Nyasia, South Dakota", 2500, "(991) 201 9415");
directory.addEmployee("Emma Watson", "1769 Polly Fork, West Nyasia, South Dakota", 2000, "(532) 427 2850");
directory.addEmployee("Leonardo Dicaprio", "1769 Polly Fork, West Nyasia, South Dakota", 4580, "(904) 188 3811");
directory.addEmployee("Maggie Smith", "1769 Polly Fork, West Nyasia, South Dakota", 1900, "(049) 737 8587");
directory.displayAll();
}

Step by step
Solved in 2 steps with 4 images

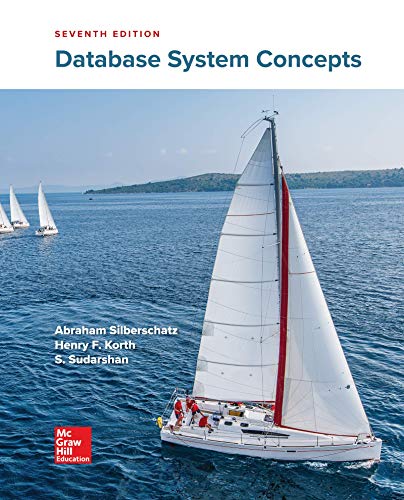
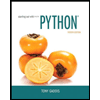
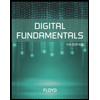
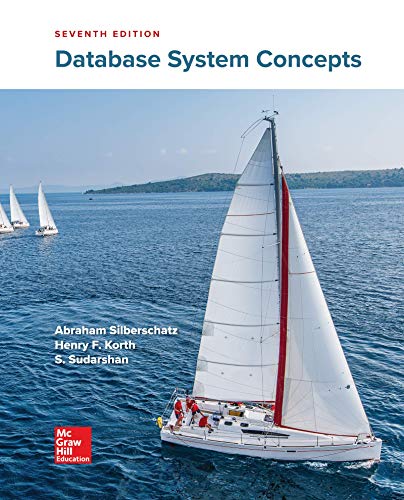
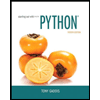
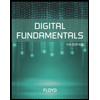
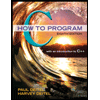
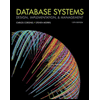
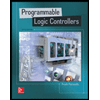