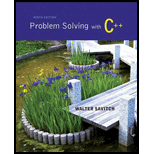
Concept explainers
Solution to Practice
Redefine CDAccount from Display 10.1 so that it is a class rather than a structure. Use the same member variables as in Display 10.1 but make them private. Include member functions for each of the following: one to return the initial balance, one to return the balance at maturity, one to return the interest rate, and one to return the term. Include a constructor that sets all of the member variables to any specified values, as well as a default constructor. Embed your class definition in a test program.

Redefine CDAccount
Program Plan:
- Include the necessary libraries.
- Use namespace.
- Define a class.
- Declare the member functions as public.
- Declare the variables as private.
- Define the main method.
- Declare the variables that are required for the program.
- Call the function.
- Display the messages.
- Add member functions
- Default constructor.
- Constructor to set specified values.
- To return interest rate.
- To return initial balance.
- To return balance at maturity.
- To return the term.
- input function (istream&);
- output function (ostream&);
Program Description:
The following C++ program redefines CDAccount from Display 10.1 to be a class rather than struct.
Explanation of Solution
Program:
//Include libraries
#include <iostream>
//Use namespace
using namespace std;
//Define class
class CDAccount
{
//Public specifiers
public:
//Declare member functions
CDAccount();
CDAccount(double bal, double intRate, int T);
double InterestRate();
double InitialBalance();
double BalanceAtMaturity();
int Term();
void input(istream&);
void output(ostream&);
//Private specifiers
private:
//Declare variables
double balance;
double interestRate; // in PER CENT
int term; // months to maturity;
};
//Main function
int main()
{
//Declare variables
double balance; double intRate;
int term;
//Constructor
CDAccount account = CDAccount(100.0, 10.0, 6);
//Display message
cout << "CD Account interest rate: "
<< account.InterestRate() << endl;
//Display message
cout << "CD Account initial balance: "
<< account.InitialBalance() << endl;
//Display message
cout << "CD Account balance at maturity is: "
<< account.BalanceAtMaturity() << endl;
//Display message
cout << "CD Account term is: " << account.Term()
<< " months" << endl;
account.output(cout);
//Display message
cout << "Enter CD initial balance, interest rate, "
<< " and term: " << endl;
account.input(cin);
//Display message
cout << "CD Account interest rate: "
<< account.InterestRate() << endl;
//Display message
cout << "CD Account initial balance: "
<< account.InitialBalance() << endl;
//Display message
cout << "CD Account balance at maturity is: "
<< account.BalanceAtMaturity() << endl;
//Display message
cout << "CD Account term is: " << account.Term()
<< " months" << endl;
account.output(cout);
//Newline character
cout << endl;
//Pause console window
system("pause");
}
//Default constructor
CDAccount::CDAccount() { /* do nothing */ }
//Constructor to set specified values
CDAccount::CDAccount(double bal, double intRate, int T)
{
balance = bal;
interestRate = intRate;
term = T;
}
//Function to return interest rate
double CDAccount::InterestRate()
{
//Return value
return interestRate;
}
//Function to return initial balance
double CDAccount::InitialBalance()
{
//Return value
return balance;
}
//Function to return the balance at maturity
double CDAccount::BalanceAtMaturity()
{
//Return value
return balance * (1 + (interestRate / 100)*(term / 12.0));
}
//Function to return the term
int CDAccount::Term()
{
//Return value
return term;
}
//Input Function
void CDAccount::input(istream& inStream)
{
//Store value
inStream >> balance;
inStream >> interestRate;
inStream >> term;
}
//Output function
void CDAccount::output(ostream& outStream)
{
//Display values
outStream.setf(ios::fixed);
outStream.setf(ios::showpoint);
outStream.precision(2);
//Display message
outStream << "when your CD matures in " << term
<< " months" << endl
<< "it will have a balance of "
<< BalanceAtMaturity() << endl;
}
Output:
CD Account interest rate: 10
CD Account initial balance: 100
CD Account balance at maturity is: 105
CD Account term is: 6 months
when your CD matures in 6 months
it will have a balance of 105.00
Enter CD initial balance, interest rate, and term:
100
10
12
CD Account interest rate: 10.00
CD Account initial balance: 100.00
CD Account balance at maturity is: 110.00
CD Account term is: 12 months
when your CD matures in 12 months
it will have a balance of 110.00
Press any key to continue . . .
Want to see more full solutions like this?
Chapter 10 Solutions
Problem Solving with C++ (9th Edition)
Additional Engineering Textbook Solutions
Mechanics of Materials (10th Edition)
Database Concepts (8th Edition)
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Starting Out with Python (4th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Electric Circuits. (11th Edition)
- Exercise docID document text docID document text 1 hot chocolate cocoa beans 7 sweet sugar 2345 9 cocoa ghana africa 8 sugar cane brazil beans harvest ghana 9 sweet sugar beet cocoa butter butter truffles sweet chocolate 10 sweet cake icing 11 cake black forest Clustering by k-means, with preprocessing tokenization, term weighting TFIDF. Manhattan Distance. Number of cluster is 2. Centroid docID 2 and docID 9.arrow_forwardChange the following code so that there is always at least one way to get from the left corner to the top right, but the labyrinth is still randomized. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. Take care that the player and the dragon cannot start off on walls. Also the dragon starts off from a randomly chosen position public class Labyrinth { private final int size; private final Cell[][] grid; public Labyrinth(int size) { this.size = size; this.grid = new Cell[size][size]; generateLabyrinth(); } private void generateLabyrinth() { Random rand = new Random(); for (int i = 0; i < size; i++) { for (int j = 0; j < size; j++) { // Randomly create walls and paths grid[i][j] = new Cell(rand.nextBoolean()); } } // Ensure start and end are…arrow_forwardChange the following code so that it checks the following 3 conditions: 1. there is no space between each cells (imgs) 2. even if it is resized, the components wouldn't disappear 3. The GameGUI JPanel takes all the JFrame space, so that there shouldn't be extra space appearing in the frame other than the game. Main(): Labyrinth labyrinth = new Labyrinth(10); Player player = new Player(9, 0); Dragon dragon = new Dragon(9, 9); JFrame frame = new JFrame("Labyrinth Game"); GameGUI gui = new GameGUI(labyrinth, player, dragon); frame.add(gui); frame.setSize(600, 600); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); public class GameGUI extends JPanel { private final Labyrinth labyrinth; private final Player player; private final Dragon dragon; //labyrinth, player, dragon are just public classes private final ImageIcon playerIcon = new ImageIcon("data/images/player.png");…arrow_forward
- Make the following game user friendly with GUI, with some simple graphics. The GUI should be in another seperate class, with some ImageIcon, and Game class should be added into the pane. The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return…arrow_forwardDiscuss the negative and positive impacts or information technology in the context of your society. Provide two references along with with your answerarrow_forwardA cylinder of diameter 10 cm rotates concentrically inside another hollow cylinder of inner diameter 10.1 cm. Both cylinders are 20 cm long and stand with their axis vertical. The annular space is filled with oil. If a torque of 100 kg cm is required to rotate the inner cylinder at 100 rpm, determine the viscosity of oil. Ans. μ= 29.82poisearrow_forward
- Make the following game user friendly with GUI, with some simple graphics The following code works as this: The objective of the player is to escape from this labyrinth. The player starts at the bottom left corner of the labyrinth. He has to get to the top right corner of the labyrinth as fast he can, avoiding a meeting with the evil dragon. The player can move only in four directions: left, right, up or down. There are several escape paths in all labyrinths. The player’s character should be able to moved with the well known WASD keyboard buttons. If the dragon gets to a neighboring field of the player, then the player dies. Because it is dark in the labyrinth, the player can see only the neighboring fields at a distance of 3 units. Cell Class: public class Cell { private boolean isWall; public Cell(boolean isWall) { this.isWall = isWall; } public boolean isWall() { return isWall; } public void setWall(boolean isWall) { this.isWall = isWall; } @Override public String toString() {…arrow_forwardPlease original work What are four of the goals of information lifecycle management think they are most important to data warehousing, Why do you feel this way, how dashboards can be used in the process, and provide a real life example for each. Please cite in text references and add weblinksarrow_forwardThe following is code for a disc golf program written in C++: // player.h #ifndef PLAYER_H #define PLAYER_H #include <string> #include <iostream> class Player { private: std::string courses[20]; // Array of course names int scores[20]; // Array of scores int gameCount; // Number of games played public: Player(); // Constructor void CheckGame(int playerId, const std::string& courseName, int gameScore); void ReportPlayer(int playerId) const; }; #endif // PLAYER_H // player.cpp #include "player.h" #include <iomanip> Player::Player() : gameCount(0) {} void Player::CheckGame(int playerId, const std::string& courseName, int gameScore) { for (int i = 0; i < gameCount; ++i) { if (courses[i] == courseName) { // If course has been played, then check for minimum score if (gameScore < scores[i]) { scores[i] = gameScore; // Update to new minimum…arrow_forward
- In this assignment, you will implement a multi-threaded program (using C/C++) that will check for Prime Numbers and Palindrome Numbers in a range of numbers. Palindrome numbers are numbers that their decimal representation can be read from left to right and from right to left (e.g. 12321, 5995, 1234321). The program will create T worker threads to check for prime and palindrome numbers in the given range (T will be passed to the program with the Linux command line). Each of the threads works on a part of the numbers within the range. Your program should have some global shared variables: • numOfPrimes: which will track the total number of prime numbers found by all threads. numOfPalindroms: which will track the total number of palindrome numbers found by all threads. numOfPalindromic Primes: which will count the numbers that are BOTH prime and palindrome found by all threads. TotalNums: which will count all the processed numbers in the range. In addition, you need to have arrays…arrow_forwardHow do you distinguish between hardware and a software problem? Discuss theprocedure for troubleshooting any hardware or software problem. give one reference with your answer.arrow_forwardYou are asked to explain what a computer virus is and if it can affect computer’shardware or software. How do you protect your computer against virus? give one reference with your answer.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
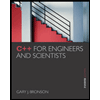
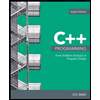