Write a program in C++ that defines a class named StringOps. Declare data members of this class as described below: variable str of data type string. variable size of data type integer which represents the length of the string str. Define member functions in StringOps as given below: parameterless and parameterized constructors that initialize the string variable str. void input():This function accepts a string as input from the user and stores it in str. The length of this string is computed and stored in the variable size. Overload operator + to create a new string obtained by concatenation of two StringOps class objects. The size of this new string should be appropriately set. Overload operator[] to return the character present at the specific index of the string variable str. The index specified begins from 1 and can range upto size. Index can also receive a negative value in which case -1 means the last character of the string variable str. The range in the negative side would be from -1 to –size. For example, if str = “hello”; then index = 1 would correspond to character „h‟; index = -1 would correspond to character „o‟, index = -2 for character „l‟ and so on. Zero index would stand invalid. int findMatch(char set[]): This function searches the characters from the array set in the string str and returns the sum of frequency of matched characters in str. For example, if str = “C++ programming” and set = {„p‟, „m‟, „+‟, „h‟}, then the value returned is 1+2+2 = 5. void display(): This function should display the string object str and its size
Write a program in C++ that defines a class named StringOps. Declare data members of
this class as described below:
variable str of data type string.
variable size of data type integer which represents the length of the string str.
Define member functions in StringOps as given below:
parameterless and parameterized constructors that initialize the string variable str.
void input():This function accepts a string as input from the user and stores it in
str. The length of this string is computed and stored in the variable size.
Overload operator + to create a new string obtained by concatenation of two
StringOps class objects. The size of this new string should be appropriately set.
Overload operator[] to return the character present at the specific index of the string
variable str. The index specified begins from 1 and can range upto size. Index can
also receive a negative value in which case -1 means the last character of the string
variable str. The range in the negative side would be from -1 to –size. For example,
if str = “hello”; then index = 1 would correspond to character „h‟; index = -1
would correspond to character „o‟, index = -2 for character „l‟ and so on. Zero index
would stand invalid.
int findMatch(char set[]): This function searches the characters from the
array set in the string str and returns the sum of frequency of matched characters in
str. For example, if str = “C++ programming” and set = {„p‟, „m‟,
„+‟, „h‟}, then the value returned is 1+2+2 = 5.
void display(): This function should display the string object str and its size

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

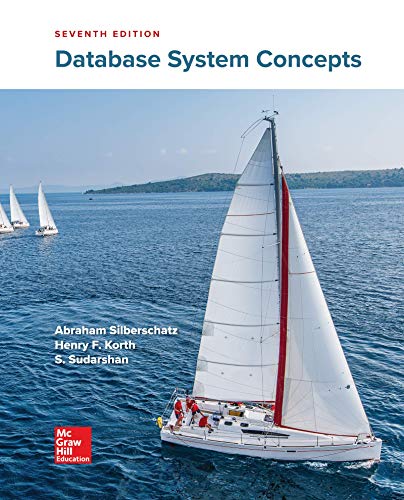
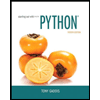
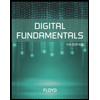
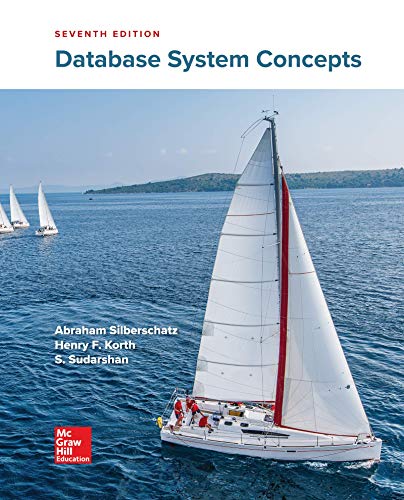
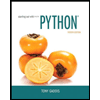
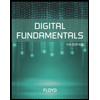
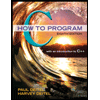
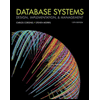
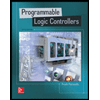