write main for this code in which create manue where user will be able to add new course enroll student search student drop course display data of course all the function are written in code just call them in main and pass value from user #include using namespace std; class student { private: int id; string name; public: student(); student(int, string); int getID(); void setID(int); string getName(); void setName(string); // student class implementation student() { id = 0; name = ""; } student(int id, string name) { this->id = id; this->name = name; } int getID() { return id; } void setID(int id) { this->id = id; } string getName() { return name; } void setName(string name) { this->name = name; } }; class Course { private: int courseID; string courseName; int capacity; int currentlyEnrolled; student *students; public: Course(); Course(int, string, int, int); // getters int getcourseID(); string getcourseName(); int getcapacity(); int getcurrentlyEnrolled(); // Setters void setcourseID(int); void setcourseName(string); void setcapacity(int); void setcurrentlyEnrolled(int); //methods are here void enroll(int, string); void drop(int); void resize(); void shift(int); int search(int); bool isfull(); void printdata(); //implementation of class course Course() { courseID = 0; courseName = " "; capacity = 3; currentlyEnrolled = 0; students = new student[capacity]; } Course(int id, string name, int capacity, int enroll) { this->courseID = id; this->courseName = name; this->capacity = capacity; currentlyEnrolled = 0; students = new student[capacity]; } bool isfull() { if (capacity == currentlyEnrolled) { return true; } else { return false; } } //how to resize the course or how to increase capacity void resize() { capacity = capacity + 5; student*newstudents = new student[capacity]; //coppying data for (int i = 0; i < currentlyEnrolled; i++) { newstudents[i].setID(students[i].getID()); newstudents[i].setName(students[i].getName()); } delete[] students; students = newstudents; } int search(int studentID) { cout << "\n\nEnter student id to Search the list: " << endl; cin >> studentID; for (int i = 0; i < currentlyEnrolled; i++) { if (students[i].getID() == studentID) { return i; } } return -1; } void Course::enroll(int id, string name) { if (isfull()) { resize(); students[currentlyEnrolled].setID(id); students[currentlyEnrolled].setName(name); currentlyEnrolled = currentlyEnrolled + 1; } else { students[currentlyEnrolled].setID(id); students[currentlyEnrolled].setName(name); currentlyEnrolled = currentlyEnrolled + 1; } } // here is for droping course void drop(int studentID) { int index = search(studentID); if (index == -1) { cout << "not found" << endl; return; } else { students[index].setID(0); students[index].setName(""); shift(index); currentlyEnrolled = currentlyEnrolled - 1; cout << "Student Course Dropped Successfully!!!!" << endl; } } void shift(int index) { for (int i = index; i < currentlyEnrolled; i++) { students[i].setName(students[i + 1].getName()); students[i].setID(students[i + 1].getID()); } } void printdata() { cout << "courseID is =" << courseID << endl; cout << "course Name is =" << courseName << endl; for (int i = 0; i < currentlyEnrolled; i++) { cout << "ID is " << students[i].getID(); } } }; int main() { }
write main for this code in which create manue where user will be able to
- add new course
- enroll student
- search student
- drop course
- display data of course
all the function are written in code just call them in main and pass value from user
#include<string>
using namespace std;
class student
{
private:
int id;
string name;
public:
student();
student(int, string);
int getID();
void setID(int);
string getName();
void setName(string);
// student class implementation
student()
{
id = 0;
name = "";
}
student(int id, string name)
{
this->id = id;
this->name = name;
}
int getID() {
return id;
}
void setID(int id) {
this->id = id;
}
string getName() {
return name;
}
void setName(string name)
{
this->name = name;
}
};
class Course
{
private:
int courseID;
string courseName;
int capacity;
int currentlyEnrolled;
student *students;
public:
Course();
Course(int, string, int, int);
// getters
int getcourseID();
string getcourseName();
int getcapacity();
int getcurrentlyEnrolled();
// Setters
void setcourseID(int);
void setcourseName(string);
void setcapacity(int);
void setcurrentlyEnrolled(int);
//methods are here
void enroll(int, string);
void drop(int);
void resize();
void shift(int);
int search(int);
bool isfull();
void printdata();
//implementation of class course
Course()
{
courseID = 0;
courseName = " ";
capacity = 3;
currentlyEnrolled = 0;
students = new student[capacity];
}
Course(int id, string name, int capacity, int enroll) {
this->courseID = id;
this->courseName = name;
this->capacity = capacity;
currentlyEnrolled = 0;
students = new student[capacity];
}
bool isfull()
{
if (capacity == currentlyEnrolled)
{
return true;
}
else
{
return false;
}
}
//how to resize the course or how to increase capacity
void resize()
{
capacity = capacity + 5;
student*newstudents = new student[capacity];
//coppying data
for (int i = 0; i < currentlyEnrolled; i++)
{
newstudents[i].setID(students[i].getID());
newstudents[i].setName(students[i].getName());
}
delete[] students;
students = newstudents;
}
int search(int studentID)
{
cout << "\n\nEnter student id to Search the list: " << endl;
cin >> studentID;
for (int i = 0; i < currentlyEnrolled; i++)
{
if (students[i].getID() == studentID)
{
return i;
}
}
return -1;
}
void Course::enroll(int id, string name)
{
if (isfull()) {
resize();
students[currentlyEnrolled].setID(id);
students[currentlyEnrolled].setName(name);
currentlyEnrolled = currentlyEnrolled + 1;
}
else {
students[currentlyEnrolled].setID(id);
students[currentlyEnrolled].setName(name);
currentlyEnrolled = currentlyEnrolled + 1;
}
}
// here is for droping course
void drop(int studentID) {
int index = search(studentID);
if (index == -1) {
cout << "not found" << endl;
return;
}
else {
students[index].setID(0);
students[index].setName("");
shift(index);
currentlyEnrolled = currentlyEnrolled - 1;
cout << "Student Course Dropped Successfully!!!!" << endl;
}
}
void shift(int index) {
for (int i = index; i < currentlyEnrolled; i++) {
students[i].setName(students[i + 1].getName());
students[i].setID(students[i + 1].getID());
}
}
void printdata() {
cout << "courseID is =" << courseID << endl;
cout << "course Name is =" << courseName << endl;
for (int i = 0; i < currentlyEnrolled; i++) {
cout << "ID is " << students[i].getID();
}
}
};
int main()
{
}

Step by step
Solved in 2 steps

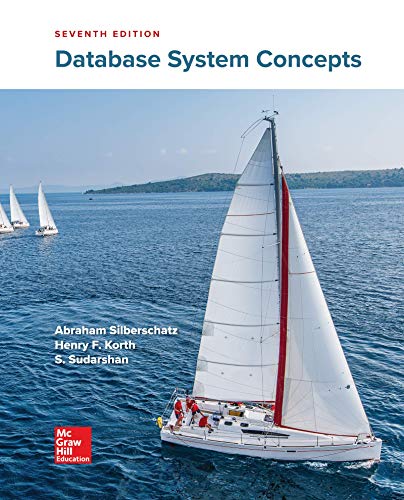
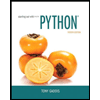
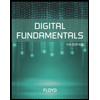
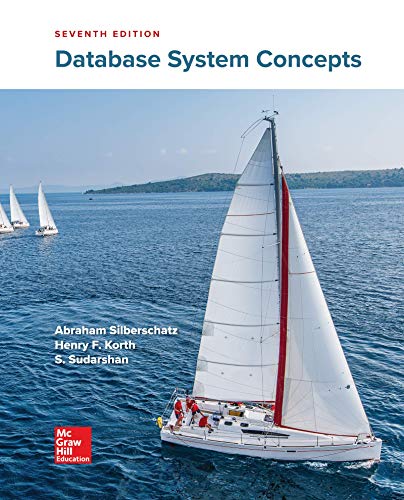
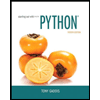
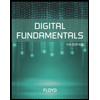
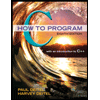
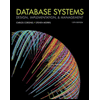
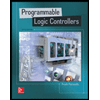