Python Code: class Node: def __init__(self, initial_data): self.data = initial_data self.next = None def __str__(self): return str(self.data) class LinkedList: def __init__(self): self.head = None self.tail = None def append(self, new_node): if self.head == None: self.head = new_node self.tail = new_node else: self.tail.next = new_node self.tail = new_node def Generate(self,num_nodes): # Your code goes here def printList(self): # Your code goes here def swap(self): # Your code goes here if __name__ == '__main__': LL = LinkedList() num_nodes = int(input()) LL.Generate(num_nodes) LL.swap() LL.printList()
Python Code:
class Node:
def __init__(self, initial_data):
self.data = initial_data
self.next = None
def __str__(self):
return str(self.data)
class LinkedList:
def __init__(self):
self.head = None
self.tail = None
def append(self, new_node):
if self.head == None:
self.head = new_node
self.tail = new_node
else:
self.tail.next = new_node
self.tail = new_node
def Generate(self,num_nodes):
# Your code goes here
def printList(self):
# Your code goes here
def swap(self):
# Your code goes here
if __name__ == '__main__':
LL = LinkedList()
num_nodes = int(input())
LL.Generate(num_nodes)
LL.swap()
LL.printList()


Step by step
Solved in 5 steps with 3 images

I get the following errors for the code above
![Traceback (most recent call last):
File "main.py", line 54, in <module>
LL. Generate (num_nodes)
File "main.py", line 32, in Generate
self.append (Node (x[i]))
IndexError: list index out of range
Output differs. See highlights below. Special character legend
Input
Your output
Expected output
-1
-2
3
1
2
3
number of nodes can't be negative, please try again!
number of nodes can't be negative, please try again!
number of nodes can't be negative, please try again!
number of nodes can't be negative, please try again!
3 2 1
Output is nearly correct, but whitespace differs. See highlights below. Special character legend
Input
1
2
24
Your output
Expected output 2
N](https://content.bartleby.com/qna-images/question/45d19586-ebc3-4f1a-8702-47e93c6193b1/726ac3fc-cb51-478e-9622-93ab9b75b5f4/tmwrbdc_thumbnail.png)
![Traceback (most recent call last):
File "main.py", line 54, in <module>
LL.Generate (num_nodes)
File "main.py", line 32, in Generate
self.append (Node (x[i]))
IndexError: list index out of range
Input
5
Morning
Noon
Input
Afternoon
Evening
Night
Your output
Your program produced no output
Expected output Night Noon Afternoon Evening Morning
Traceback (most recent call last):
File "main.py", line 54, in <module>
LL.Generate (num_nodes)
File "main.py", line 32, in Generate
self.append (Node (x[i]))
IndexError: list index out of range
5
a
b
e
Your output Your program produced no output
Expected output e b c da
Traceback (most recent call last):
File "main.py", line 54, in <module>
LL. Generate (num_nodes)
File "main.py", line 29, in Generate
value1=input()
EOFError: EOF when reading a line
Input 0
Your output Your program produced no output
Expected output Empty List!](https://content.bartleby.com/qna-images/question/45d19586-ebc3-4f1a-8702-47e93c6193b1/726ac3fc-cb51-478e-9622-93ab9b75b5f4/9h8l2sqf_thumbnail.png)
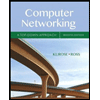
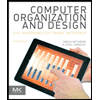
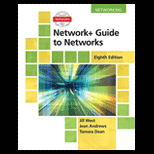
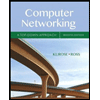
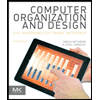
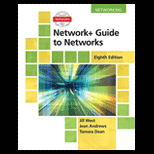
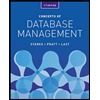
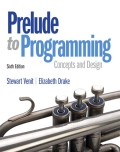
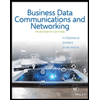